Introduction
This post will show you how to troubleshoot runtime exceptions on your ESP32 microcontroller powered by the Arduino framework. We will use ESP32 exception decoder tools to debug errors being thrown by our microcontroller in both Arduino IDE and PlatformIO IDE.
If you want to see a video demo presentation of this post then please see the below video or watch it on my YouTube channel.
What is Guru Meditation Error: Core 1 panic’ed…
Encountering errors and exceptions when programming using the versatile ESP32 WiFi-enabled Microcontroller unit (MCU) is normal. You might have forgotten to initialize your variables or your wiring or schematic is not correct causing the library that you are using to throw an error. Unfortunately, the ESP32 runtime errors that you are seeing do not show you the cause but some register dumps that aren’t helpful.
The Guru Meditation Error coming from your ESP32 is a generic error and the cause are varied and could be circuit/program specific. It usually shows you the general cause of the error, some register dumps, and the backtrace objects.
Guru Meditation Error: Core 1 panic'ed (LoadProhibited). Exception was unhandled.
Core 1 register dump:
PC : 0x400014e8 PS : 0x00060830 A0 : 0x800d133c A1 : 0x3ffb96d0
A2 : 0xa341aa26 A3 : 0xa341aa24 A4 : 0x000000ff A5 : 0x0000ff00
A6 : 0x00ff0000 A7 : 0xff000000 A8 : 0x00000000 A9 : 0x00000001
A10 : 0x00000001 A11 : 0x00060823 A12 : 0x00060820 A13 : 0x00000020
A14 : 0x00000000 A15 : 0x00000000 SAR : 0x0000000a EXCCAUSE: 0x0000001c
EXCVADDR: 0xa341aa24 LBEG : 0x4000c2e0 LEND : 0x4000c2f6 LCOUNT : 0xffffffff
ELF file SHA256: 0000000000000000
Backtrace: 0x400014e8:0x3ffb96d0 0x400d1339:0x3ffb96e0 0x400d136d:0x3ffb9700 0x400d0509:0x3ffb9720 0x40089792:0x3ffb9750
Looking at the terminal logs can you guess what the exact error is? I guess if you are a hero in a Marvel universe then you could make that error human-readable. The process of converting those values into a language that humans could understand is called “decoding” and we will be using a tool called the EspExceptionDecoder in Arduino and adding a monitor filter in PlatformIO IDE.
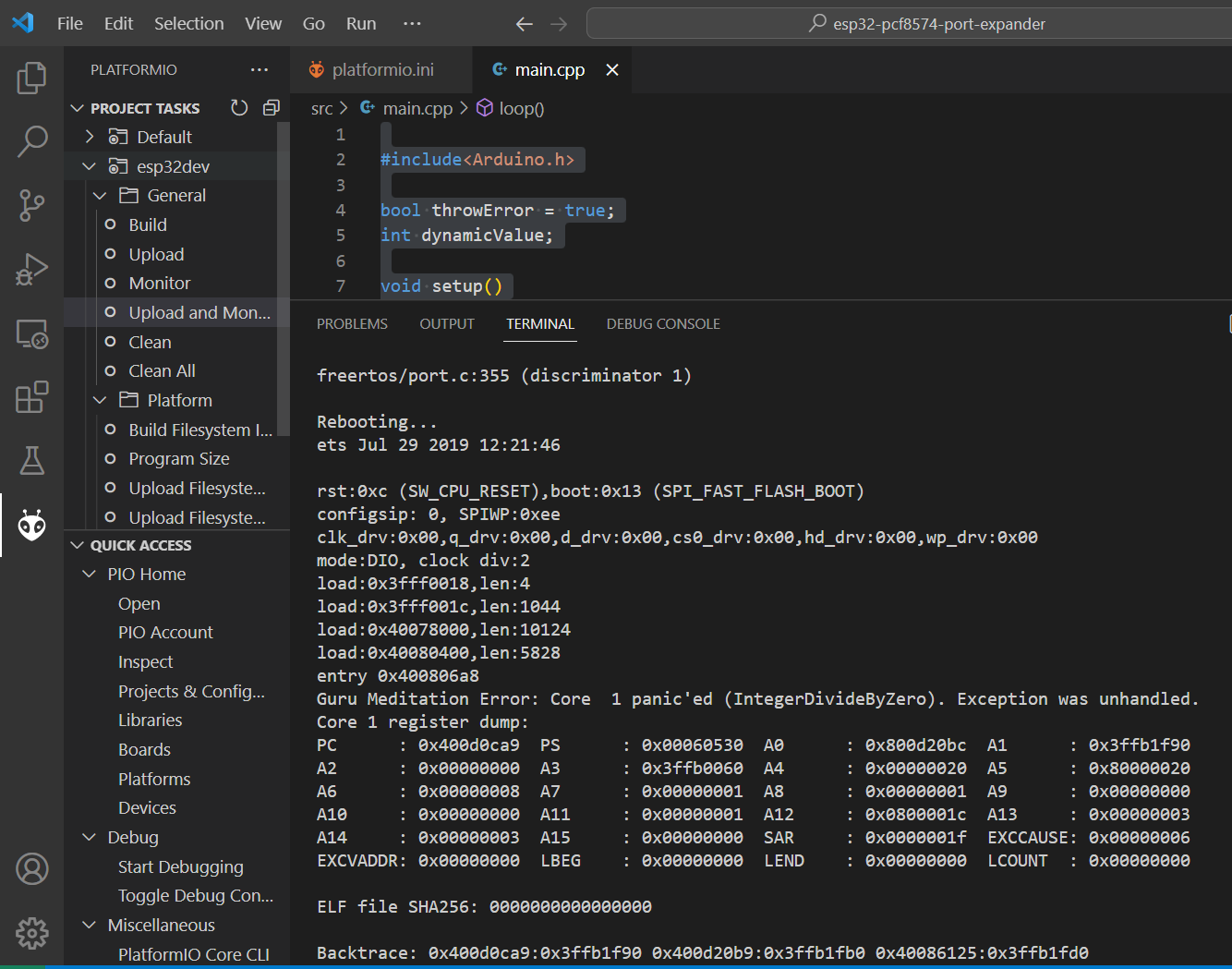
Parts/Components Required
The followings are the component needed to follow along with this post.
- ESP32 – Amazon | AliExpress | Bangood
- Breadboard – Amazon | AliExpress | Bangood
Disclosure: These are affiliate links and I will earn small commissions to support my site when you buy through these links.
Simulate an ESP32 error
Let’s create a very simple Arduino program that would throw a runtime exception.
#include<Arduino.h>
bool throwError = true;
int dynamicValue;
void setup()
{
Serial.begin(115200);
}
void loop()
{
delay(5000);
if (throwError)
dynamicValue = 0;
else
dynamicValue = 1;
float error = 1 / dynamicValue;
Serial.println(error);
}
In the function loop()
, we are simulating some logic here but the endpoint is that we would be dividing 1 by zero which is not possible and will cause a runtime exception.
Fortunately, we have a tool that could help us analyze the problem and I will show you how to do it in both Arduino IDE and PlatformIO IDE.
Related Content:
PlatformIO Tutorial for Arduino Development
Arduino IDE
Before we continue let me remind you that as of this time (April 2023), the Arduino IDE 2 support for tools and plugins is still not possible. The EspException decoder is a tool written in Java and is a plugin that we can use to decode the exception. Since plugins are still not supported in Arduino IDE 2 then you can follow along with this article by downloading the old (legacy) Arduino IDE 1.8.19 from here.
How to install ESPException Decoder in Arduino IDE?
We can follow the below steps from the project documentation to install this software.
- Make sure you use one of the supported versions of Arduino IDE and have ESP8266/ESP32 core installed.
- Download the tool archive from the releases page.
- In your Arduino sketchbook directory, create a tools directory if it doesn’t exist yet.
- Unpack the tool into the tools directory (the path will look like /Arduino/tools/EspExceptionDecoder/tool/EspExceptionDecoder.jar).
- On newer versions of Linux (Ubuntu 20.04), you may need to install the libncurses5 and libpython2.7 packages:
sudo apt install libncurses5 libpython2.7
- Restart Arduino IDE.
On my Windows/PC laptop, I have the following setup after following the steps above.
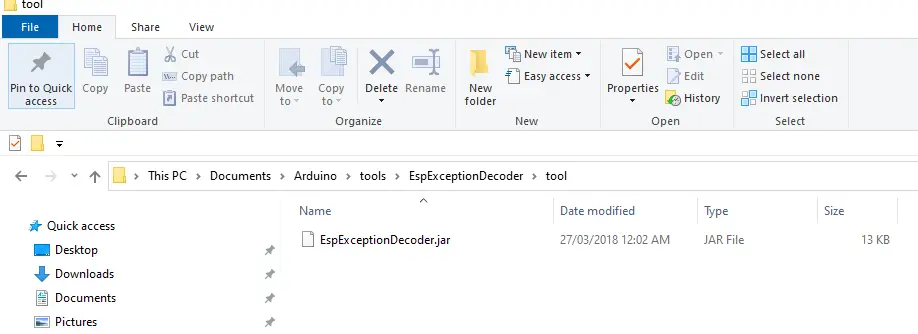
How to use the ESPException Decoder?
Create a sketch and write the sample program above that would throw an exception and upload it into the flash memory of your ESP32 MCU. Afterward, open the Serial Monitor in Arduino IDE.
You would encounter the following error. Currently, our program is short and perhaps you would get a hint that the exact error is Guru Meditation Error: Core 1 panic’ed (IntegerDivideByZero). But what if you are writing a big program then it would be hard to pinpoint what part of the code is throwing the exception.
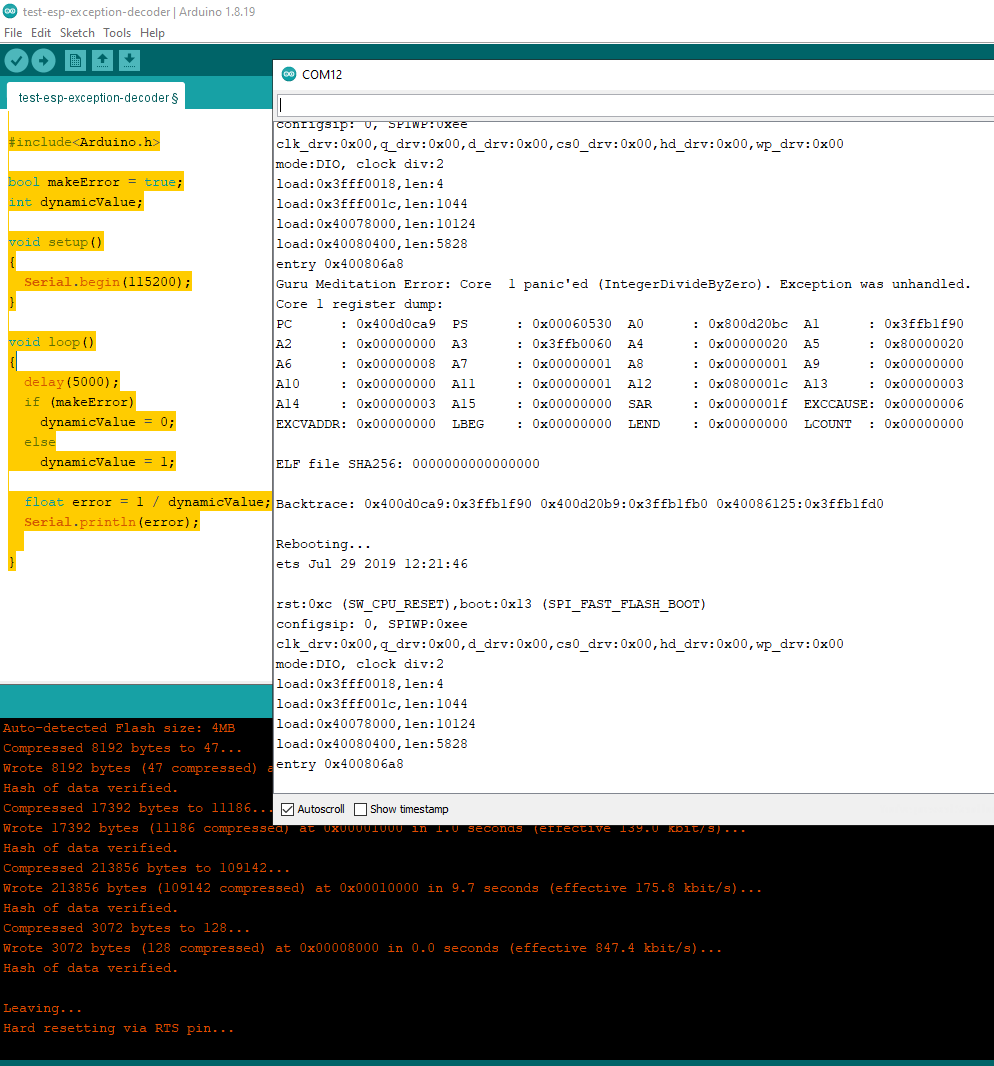
When you see the error, click Tools then ESP Exception Decoder
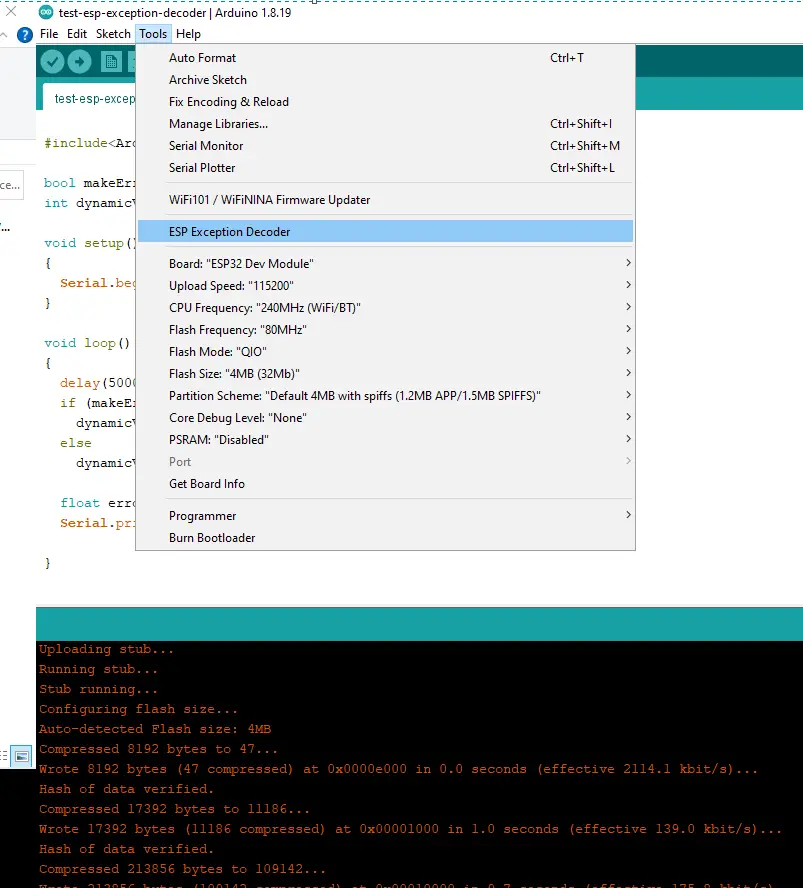
A new window would pop up and then you can paste the error that you are seeing from your Serial Monitor. I usually copy anything that starts from the “Guru Meditation Error” up to the “Backtrace“.
Next, you would see the line number where the exception has occurred and you can check that the cause of the error was our logic where we divide a positive number by zero.
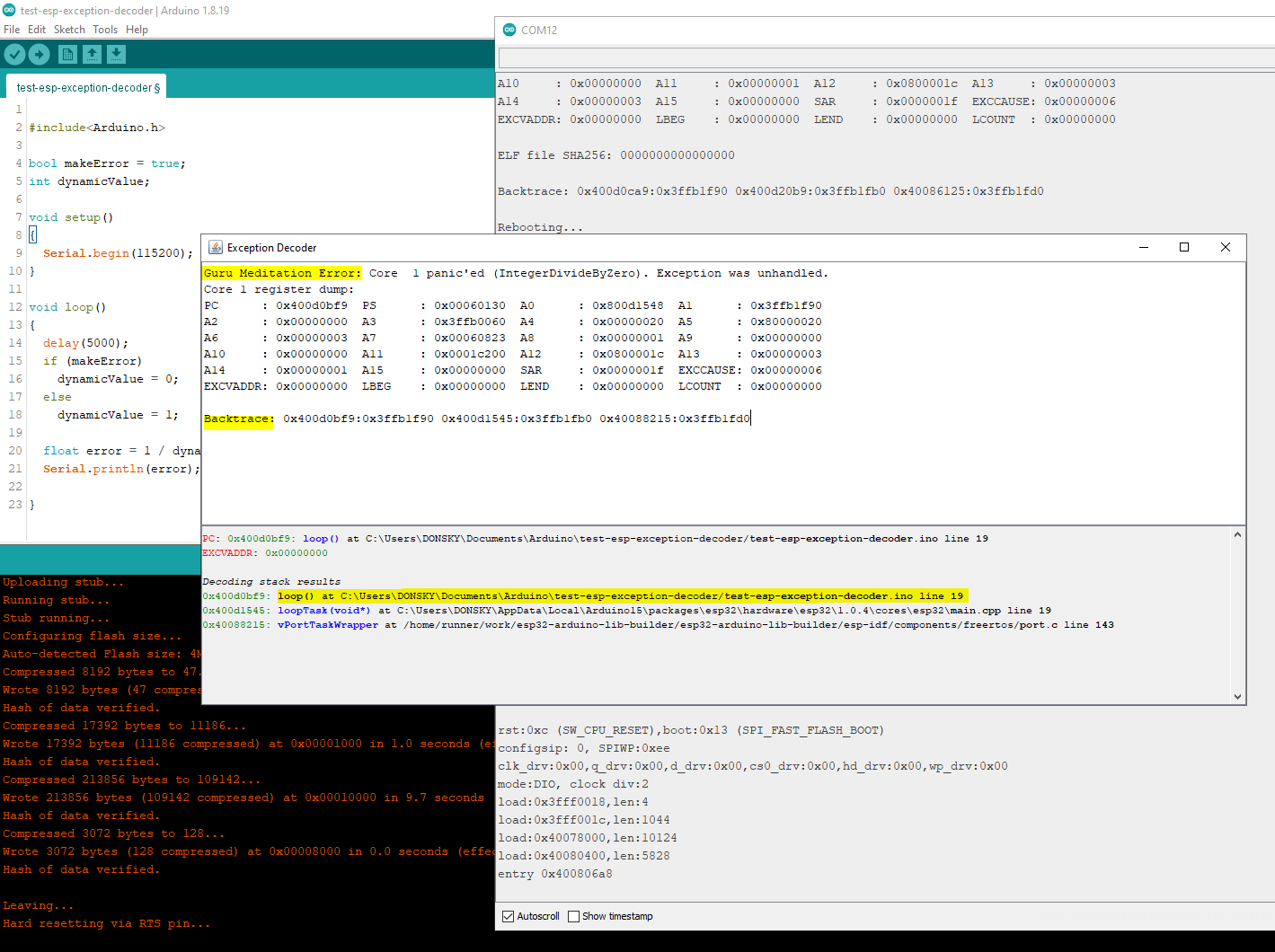
This trace is really helpful when you are programming your ESP32 as it would pinpoint the line that is causing the exception so this ESP Exception Decoder plugin is really cool!
Unfortunately, this is still not working on the newest Arduino 2 IDE so you may have to keep a copy of the legacy Arduino IDE 1 on your workstation.
PlatformIO IDE
If you are using the PlatformIO IDE then you are in luck as we don’t need to do anything compared to using Arduino IDE (especially the legacy Arduino IDE 1). By just configuring something in your platform.ini file then you would be able to activate the ESP32 Exception Decoder on the fly.
Create a new program and select ESP32 as the board and then paste our test program on the main.cpp file.
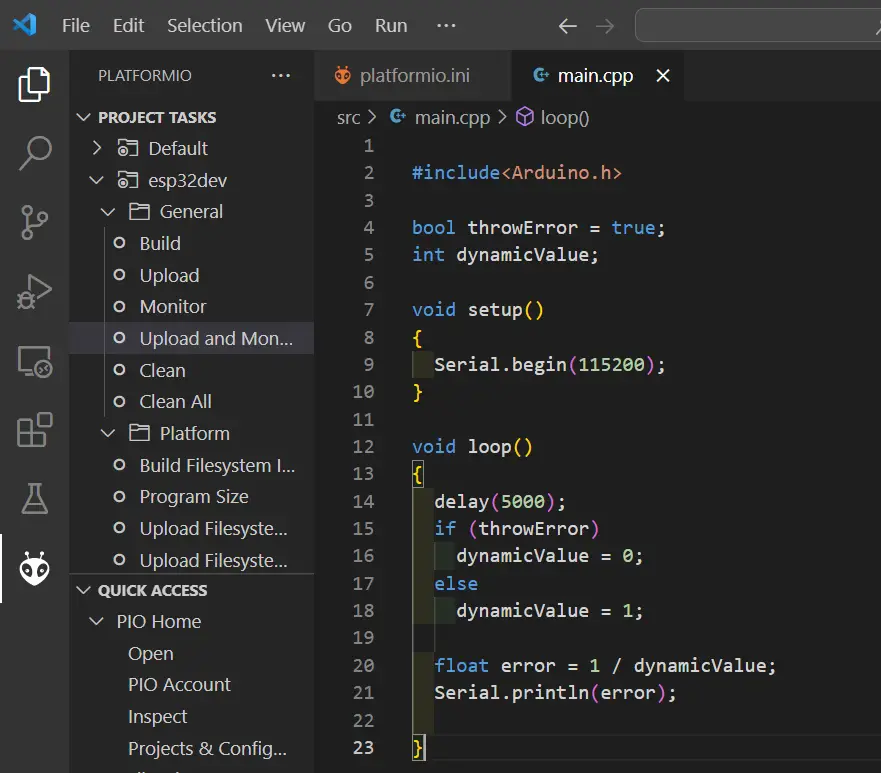
Open your platform.ini file and set the following monitor filter.
[env:esp32dev]
platform = espressif32
board = esp32dev
framework = arduino
monitor_speed = 115200
monitor_filters = esp32_exception_decoder
Upload your sketch into the ESP32 the microcontroller flash file system using the task Upload and Monitor and then check the terminal window.
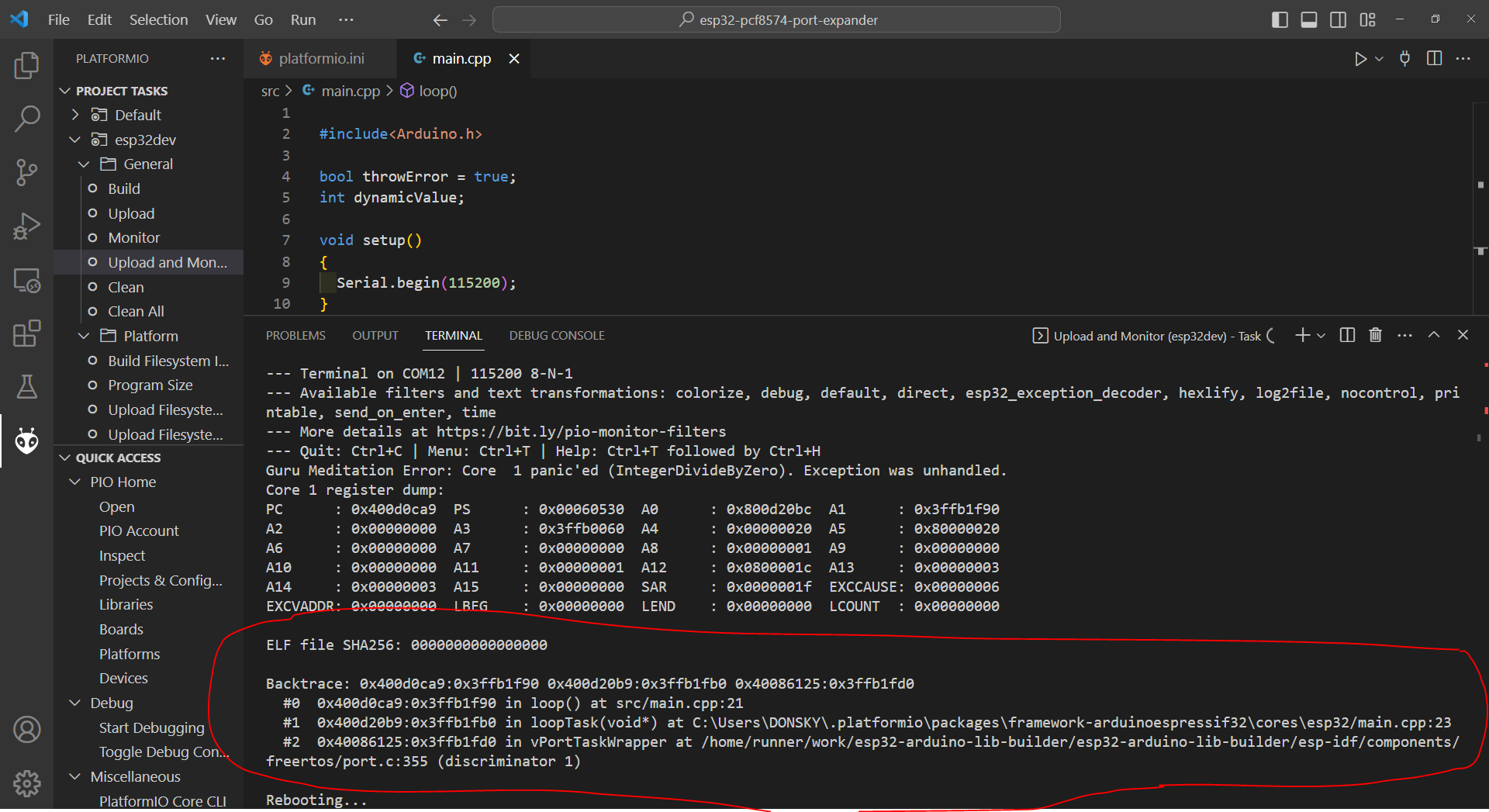
As you can see, it automatically decodes the hex dump and shows you what line of your program is causing this runtime exception. How cool and simple isn’t it? This is the exact reason why I have used PlatformIO on any of my Arduino development projects.
Wrap Up
In this post, we were able to explore how to figure out the cause of your runtime exception on your ESP32 microcontroller. Using these cool ESP32 exception decoder tools and then figuring out the solution to your issues would be a walk in the park.
I hope you learned something!
Leave a Reply