Introduction
This short post will discuss how we can read sensor values coming from a Light Dependent Resistor (LDR) or a photoresistor using a NodeMCU ESP8266 board. The Serial Monitor of our PlatformIO IDE then outputs the values read from our sensor.
A photoresistor or an LDR or a photocell acts like a variable resistor whose value changes depending on the intensity of light that is exposed. If exposed to high light intensity then the resistance is low otherwise it could be high as in megaohms when it is in the dark. This makes this sensor ideal for Internet of Things (IoT) project that needs to act differently depending on the amount of light. Home automation projects that get triggered when it is nighttime can make use of this sensor.
How does LDR or Photoresistor work?
If you need to detect the presence (or absence) of light then use LDR or Photoresistors (or PhotoCells) in your projects The intensity of light causes the resistance to vary which could be from zero to mega ohms in values.
Semiconductor materials that have high light sensitivity properties are used in creating this component. Semiconductors tend to exhibit high resistance by default. The number of electrons is few but when the semiconductor materials receive light photons then it triggers the electrons to break free causing conductivity.
These components are widely available and are easy to use in microcontroller projects.
Components/Tools
- LDR or Photoresistor – Amazon | AliExpress
- ESP8266 module (I used NodeMCU ESP8266 board here) – Amazon | AliExpress | Bangood
- Breadboard – Amazon | AliExpress | Bangood
- Connecting wires – Amazon | AliExpress | Bangood
Disclosure: These are affiliate links and I will earn small commissions to support my site when you buy through these links.
IDE/Software
I used Visual Studio Code with the PlatformIO extension installed in developing this project. If you are in Windows and have not yet installed Visual Studio Code then you can check my post about how to Install Visual Studio Code or VSCode in Windows. If you are not familiar with PlatformIO then please read this PlatformIO Tutorial for Arduino Development where I detailed how to get started with development using the PlatformIO IDE extension
Schematic/Wiring Diagram – ESP8266 with LDR/Photoresistor
The following image below is the schematic diagram of our circuit. The Photoresistor/LDR is in series with a resistor to create a voltage divider circuit. The resistance of the Photoresistor/LDR varies depending on the intensity of the light. The A0 pin of the NodeMCU 8266 reads the amount of voltage drop. Note that the resistance of the Photoresistor/LDR is inversely proportional to the intensity of light captured by the sensor.
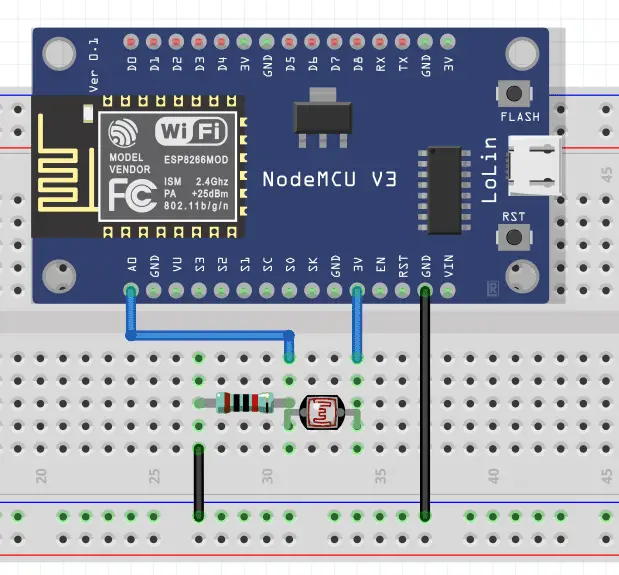
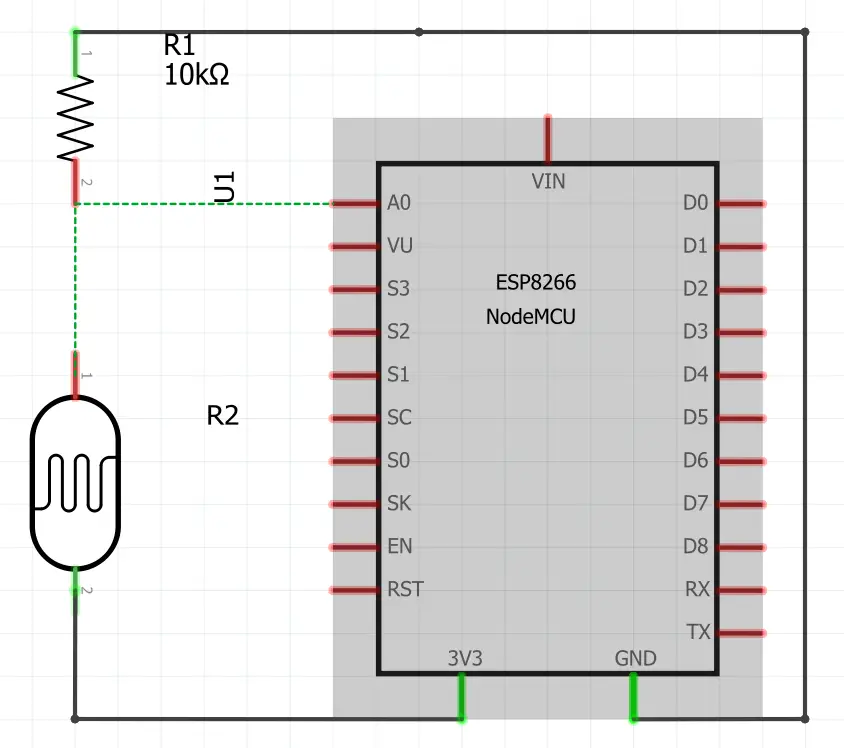
Code
The code for this project is in my GitHub account and you can view it here.
/*
Title: Interfacing Photocell or LDR into NodeMCU ESP8266
Description: Read LDR or Phot0resistor using ESP8266
Author: donsky
For: www.donskytech.com
Date: September 20, 2022
*/
#include <Arduino.h>
int sensorVal = 0;
const int ANALOG_READ_PIN = A0;
void setup()
{
Serial.begin(115200);
}
void loop()
{
sensorVal = analogRead(ANALOG_READ_PIN);
// Values from 0-1024
Serial.println(sensorVal);
// Convert the analog reading to voltage
float voltage = sensorVal * (3.3 / 1023.0);
// print the voltage
Serial.println(voltage);
}
We declare our ANALOG_READ_PIN and set it to the A0 pin of our NodeMCU ESP8266 microcontroller.
In the loop function, we use the analogRead Arduino function to read the voltage drop at the A0 pin. Values read from the A0 pin are mapped from the 0 to 1023 range.
If you want to see the voltage drop then you can compute it by using the method above.
Wrap up
That’s it! Happy Exploring!
Related Content:
Plot Real-time Chart display of Sensor Readings – ESP8266/ESP32
PlatformIO Tutorial for Arduino Development
Leave a Reply