Introduction
This post will show you how to serve static files using Node.js and the Express server framework.
Prerequisites
You should have installed Node.js on your machine and knows the basic of how to create a web application using the Express web framework.
Related Content:
Install Node.js on Windows
At the end of this article, we will be creating a sample web application created using Node.js and an Express server that serves static HTML/CSS/Javascript files.
Below is the sample image of the sample static web application that we are gonna be creating using our Node.js and Express server.
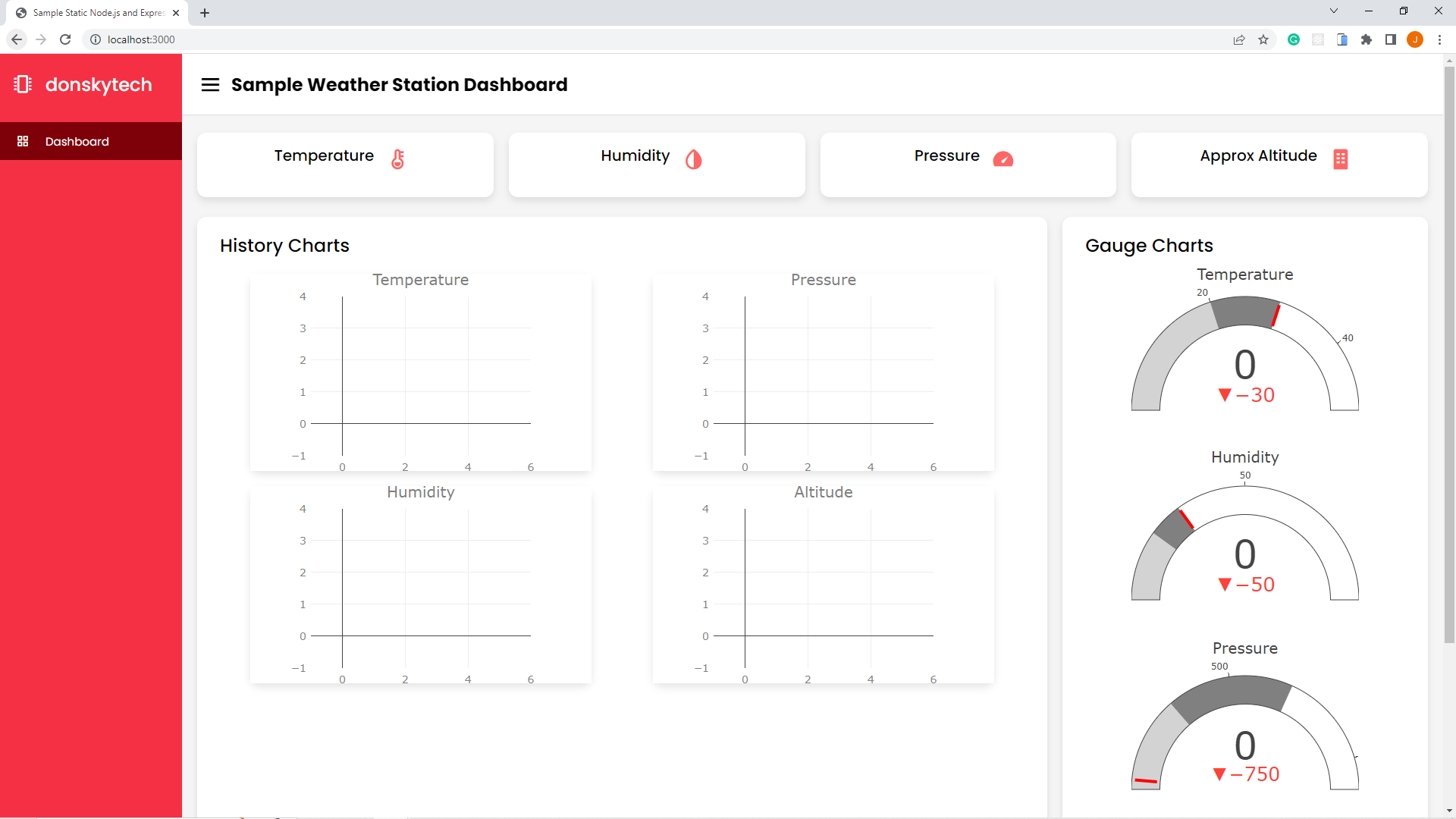
Steps on how to serve static files in Node.js and Express Server
1. Set up Express Server
Create a folder where we could create our project files
mkdir sample-static-node-express-web-application
cd sample-static-node-express-web-application
Let’s initialize our application with Node.js so that we can have a package.json
file where we could list down our dependencies.
npm init -y
Install our Express dependencies.
npm install express --save
2. Create our Express Server Web Application
Create an index.js file where we would place the logic of our Express server.
touch index.js
Edit your index.js
and place the following content.
const express = require('express');
const app = express();
const PORT = 3000;
app.get('/', (req, res) => {
res.send('Hello World!');
});
app.listen(PORT, () => console.log(`Server listening on port: ${PORT}`));
The code above will open a very basic Express server web application that will print “Hello World” on the browser.
3. Configure our package.json script
Edit your package.json file and add the following script to it. Replace any other scripts if there are any.
{
"name": "sample-static-node-express-web-application",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"start": "node index.js"
},
"keywords": [],
"author": "",
"license": "ISC",
"dependencies": {
"express": "^4.18.2"
}
}
Now open another terminal and type in the following command to start our web application.
npm start
This should show the following messages.
> sample-static-node-express-web-application@1.0.0 start C:\git\sample-static-node-express-web-application
> node index.js
Server listening on port: 3000
Open your browser and type in the following URL
http://localhost:3000
This should open up the following image.
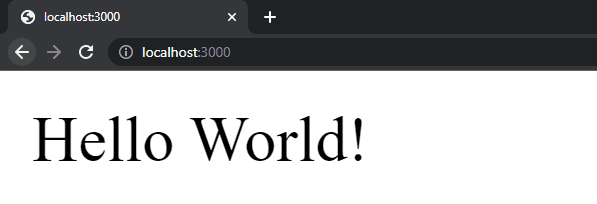
4. Setup our directory structure
Create a directory named “public” inside your project files then copy the sample files from my GitHub repository. The HTML/CSS/Javascript file contains the static file that will create our test web application.
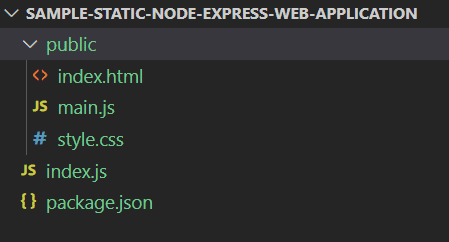
I won’t be explaining so much about the code in these files as that is not the point of this post but these are the static files that make up our test web application.
5. Serve static files in your Express Server
The Express web framework provides a way to serve static files by adding the following middleware function.
app.use(express.static('public'));
The argument you pass in the express.static()
function is the name of the directory where you want Express to load static files which in this case here is our “public” folder.
Edit your index.js
file and make sure that it will look like this.
const express = require('express');
const app = express();
const PORT = 3000;
app.use(express.static('public'));
app.listen(PORT, () => console.log(`Server listening on port: ${PORT}`));
Now close the currently running node.js terminal by sending the Ctrl+C keyboard command and then restart the application again by executing the below command.
npm start
If everything goes well then you should be able to see the following displayed in your browser.
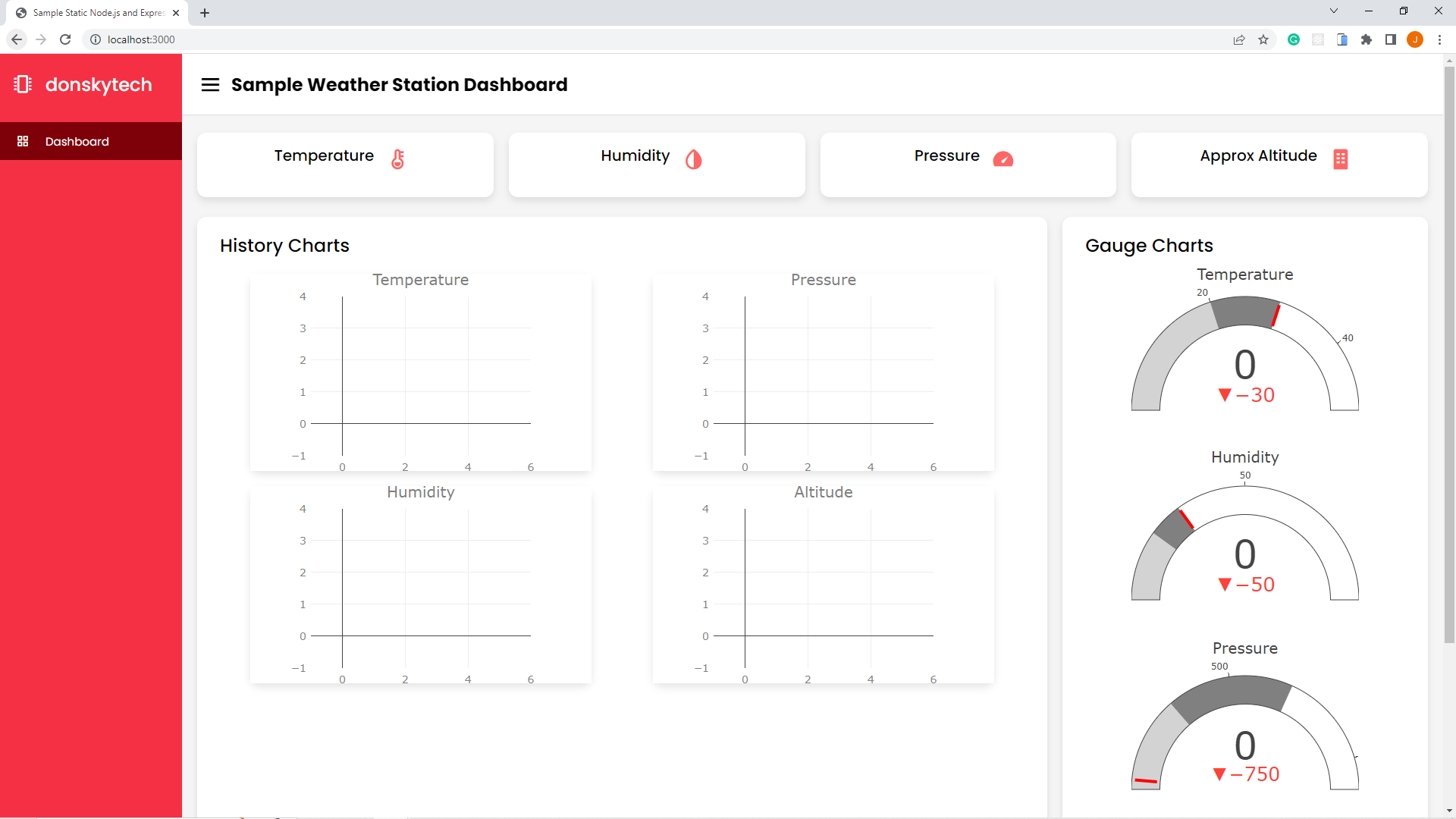
Wrap Up
We have discussed how to display static files like HTML/CSS/Javascript or even images when using Node.js and Express server in this post.
Related Content:
Build REST API Using Node.js, Express, and MongoDB
Using Node.js and React to display a chart of real-time sensor readings
Leave a Reply