Introduction
This post will show you how to interface and read sensor values from your Light Dependent Resistor (LDR) or photoresistor using your MicroPython device.
If you want to see this post in video format then please see below or watch it on my YouTube channel.
What is an LDR or Photoresistor?
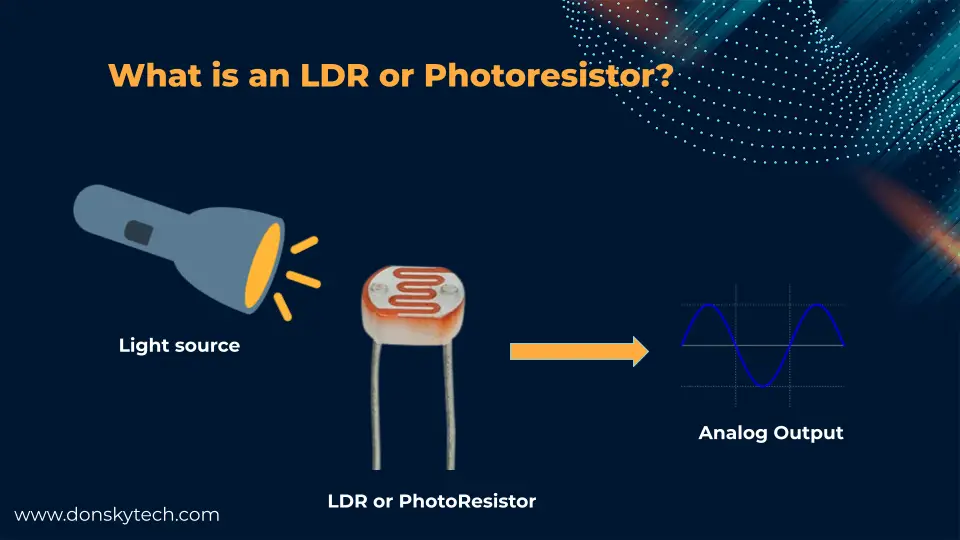
If you need to detect the presence (or absence) of light then use LDR or Photoresistors (or PhotoCells) in your Internet of Things (IoT) projects. The intensity of light causes the resistance to vary which could be from zero to mega ohms in value.
Semiconductor materials that have high light sensitivity properties are used in creating this component. Semiconductors tend to exhibit high resistance by default. The number of electrons is few but when the semiconductor materials receive light photons then it triggers the electrons to break free causing conductivity.
A note about ADC
The output of an LDR or a photoresistor is an analog signal and for MicroController to understand its value then an Analog to Digital Converter (ADC) is needed. The majority of the popular microcontrollers have pins that have a built-in ADC so reading this component does not need to be complicated.
I am using a Raspberry Pi Pico W device in this post but the logic here is applicable to other microcontroller devices such as the ESP32/ESP8266 MicroPython device. The image below shows our Raspberry Pi Pico W pinout with the ADC0-ACD2 pins (GP26, GP27, and GP28) available to be used as inputs.
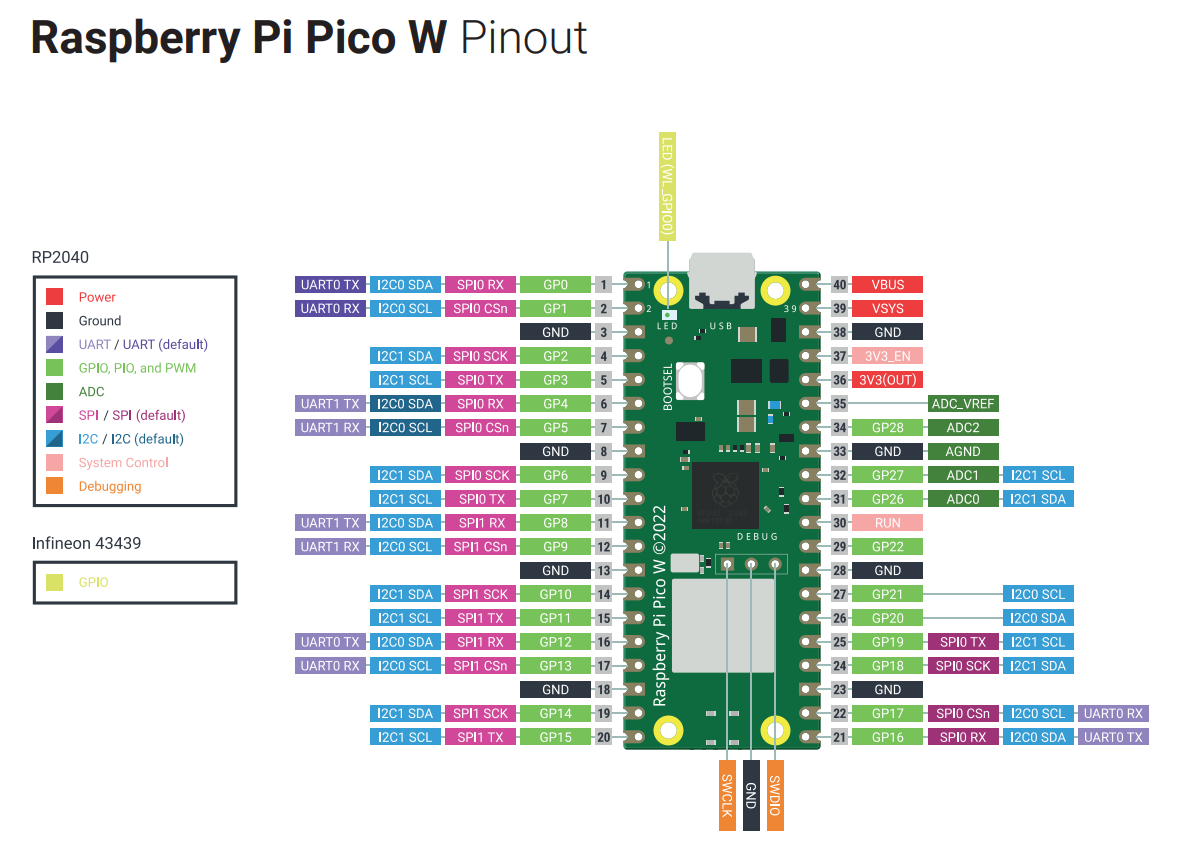
Parts/Components
Below are the parts or components needed to follow along with this post.
- Raspberry Pi Pico W – Amazon | AliExpress
- LDR or Photoresistor – Amazon | AliExpress
- Resistor – Amazon | AliExpress | Bangood
- Breadboard – Amazon | AliExpress | Bangood
- Connecting Wires – Amazon | AliExpress | Bangood
Disclosure: These are affiliate links and I will earn small commissions to support my site when you buy through these links.
Prerequisites
You should have installed the latest MicroPython firmware on your device.
Related Content:
How to Install MicroPython firmware on Raspberry Pi Pico?
How to install MicroPython on ESP32 and download firmware
I have used Thonny IDE in developing this project.
Related Content:
MicroPython Development Using Thonny IDE
How to guides in Thonny IDE using MicroPython
Wiring/Schematic
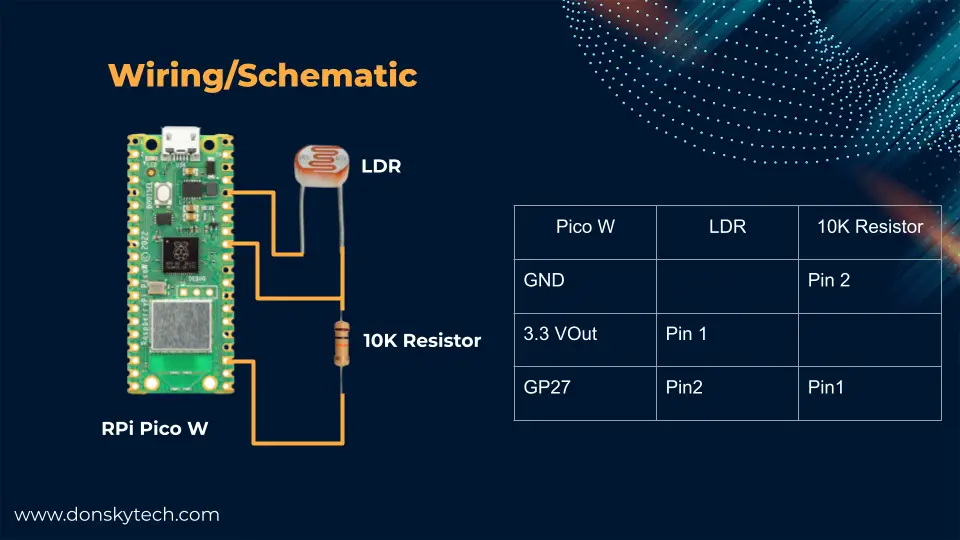
The above image will show how you can connect your LDR or Photoresistor with your MicroPython device. As you can see, we are creating a voltage divider circuit with the LDR and the 10KOhm resistor. We then connect our GP27 (or any other ADC Pin) to the connection between the LDR and the resistor.
Code
The code for this project is available in my GitHub repository and you can download it as a zip file or clone it using Git.
git clone https://github.com/donskytech/micropython-raspberry-pi-pico.git
cd micropython-raspberry-pi-pico
Open this project folder in Thonny IDE.
Basic Read
The simplest way to read an LDR or photoresistor using MicroPython is shown below. The read_u16()
function of the ADC
class will return a value between 0-65535. Values from zero mean there is an ample supply of light and those close to 65535 mean the absence of it.
from machine import Pin
import time
adc = machine.ADC(Pin(27))
while True:
print(adc.read_u16())
time.sleep(1)
Below is the sample output when running this python file.
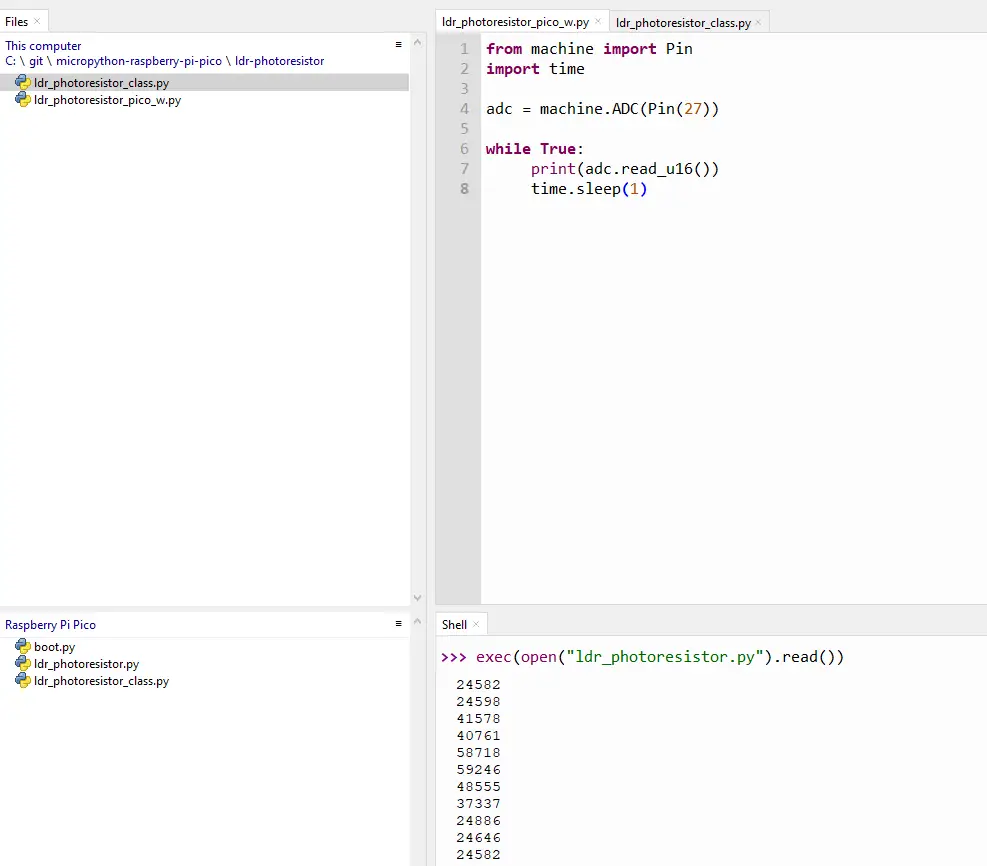
Using Class
The code above is enough if you want to just read the values of an LDR but if you wanted to make it modular then using a Python class is much more helpful. Below is the sample code on how to do this.
from machine import Pin
import time
class LDR:
def __init__(self, pin):
self.ldr_pin = machine.ADC(Pin(pin))
def get_raw_value(self):
return self.ldr_pin.read_u16()
def get_light_percentage(self):
return round(self.get_raw_value()/65535*100,2)
ldr = LDR(27)
while True:
print(ldr.get_light_percentage())
time.sleep(1)
Below is the sample output when running this python file.
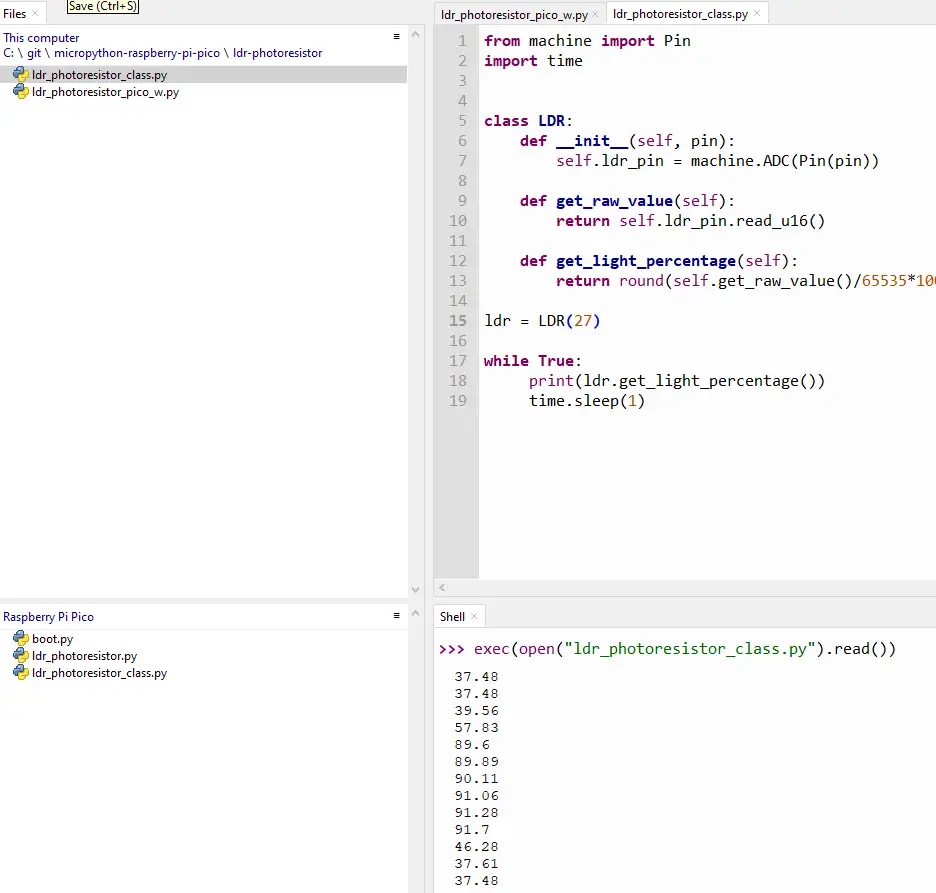
Let us run through how this code works.
from machine import Pin
import time
Import the necessary packages to communicate with the GPIO pin.
class LDR:
def __init__(self, pin):
self.ldr_pin = machine.ADC(Pin(pin))
def get_raw_value(self):
return self.ldr_pin.read_u16()
def get_light_percentage(self):
return round(self.get_raw_value()/65535*100,2)
ldr = LDR(27)
We create an LDR class with the pin parameter as input. We have declared the two functions get_raw_value()
and get_light_percentage()
. The get_raw_value()
is similar to the basic code above while the get_light_percentage()
computes the light percentage by dividing the raw value by the maximum amount which is 65535 and multiplying by 100. We then declare an object named ldr and pass it in the GPIO pin 27.
while True:
print(ldr.get_light_percentage())
time.sleep(1)
This is an infinite loop that will read the LDR or photoresistor using our class LDR
.
That is how easy it is to read this sensor.
How to run the python files?
Upload the project files to your MicroPython device using the Thonny IDE. If you do not know how this is done then you can follow this.
Once uploaded then execute the below command.
exec(open("ldr_photoresistor.py").read())
exec(open("ldr_photoresistor_class.py").read())
Wrap Up
I have discussed how you can read the LDR or Photoresistor using your MicroPython device in this post. We will use this project in future Internet of Things (IoT) projects.
I hope you learned something. Happy Exploring!
Leave a Reply