Introduction
Do you feel the need to interface your Internet of Things (IoT) projects with a database? Maybe you could try incorporating the MongoDB NoSQL database and see how powerful your projects would become.
If you want to see a video demo presentation of this project then please see below or watch it on my YouTube channel.
What is this tutorial series all about?
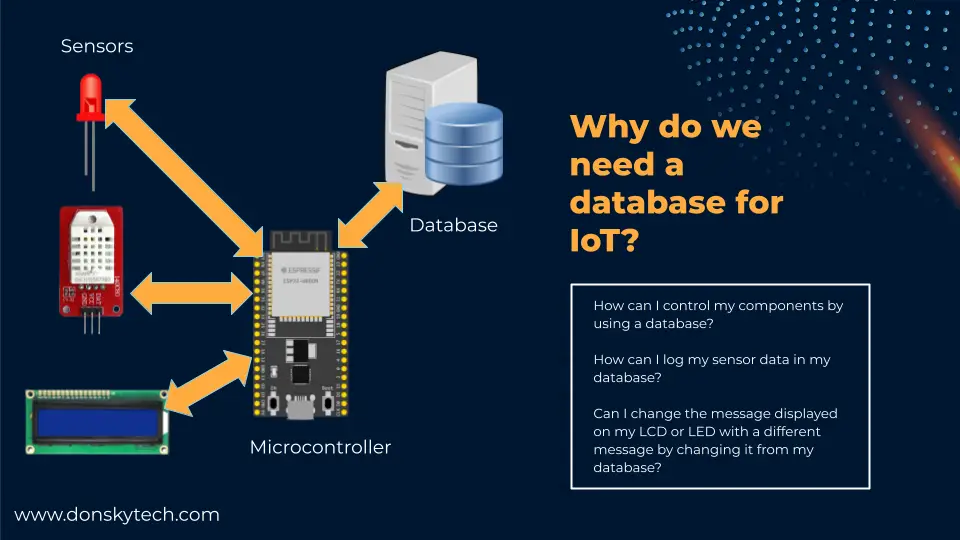
In this tutorial series, I would try to answer the common questions that I often see on the internet like:
- How can I control my components by using a database?
- How can I log my sensor data in my database?
- Can I change the message displayed on my LCD or LED with a different message by changing it from my database?
This is my own unique series that will give you an idea of why incorporating a database particularly the MongoDB database will help you a lot in your Internet of Things (IoT) projects. I hope that this series would help you in your next projects.
MongoDB IoT Tutorial Series Roadmap
The below list would tell you the different topics that we are going to discuss in this series.
- Part 1 – What is MongoDB database and why do we need it in our IoT projects?
- Part 2 – MongoDB Atlas: An Overview and how to get started
- Part 3 – Create a REST API Server with Python, Flask, and MongoDB
- Part 4 – Control your Arduino IoT projects with a MongoDB database
- Part 5 – Control your Raspberry Pi IoT projects with a MongoDB database
- Part 6 – Control your MicroPython IoT projects with a MongoDB database
- Part 7 – Arduino Data Logger using MongoDB Database
- Part 8 – Raspberry Pi Temperature Logger using MongoDB Database
- Part 9 – MicroPython Sensor Logger using MongoDB Database
This post is Part 1 of this series where I would introduce to you what the MongoDB database is and the different major objects you need to know.
What is a database?
When we talk about a database in a traditional sense such as the popular MySQL, PostgreSQL, or even the enterprise-grade Oracle Database then we are talking about the RDBMS(relational database management system) type or relational database in short.
A relational database is composed of tables, fields, indexes, etc. Each blue box is a table and each table contains fields that represent a particular entity. Each field in a table represents particular information such as the first name, last name, etc. At the same time, the collection of fields represents the schema of that table.
The employees table represents information regarding each employee like “John Doe”. The departments table represents the departments in a company such as the “purchasing”, “hr” or “manufacturing”
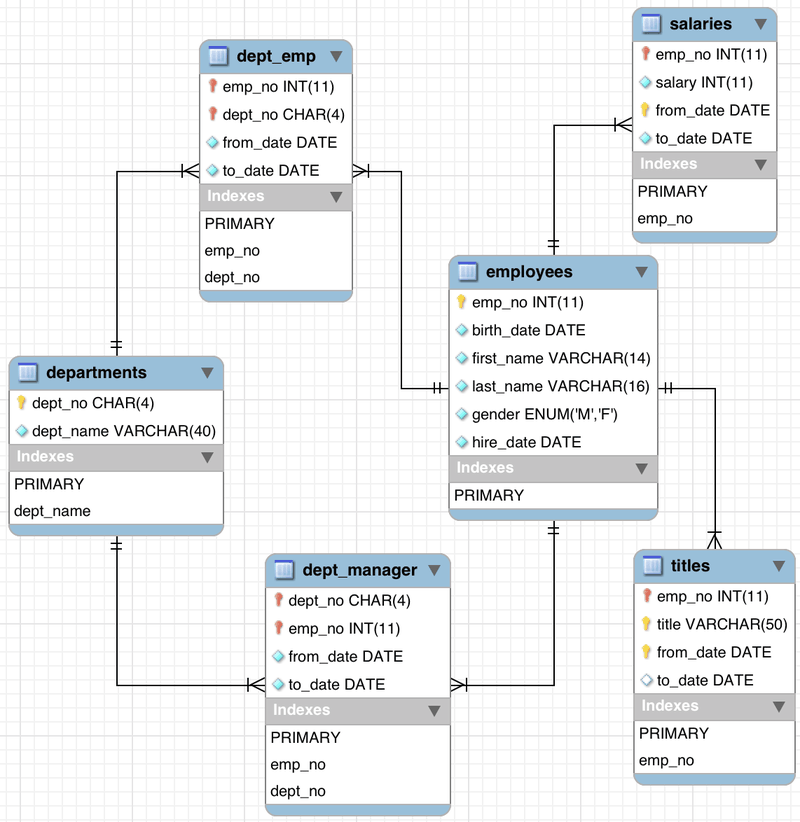
The line between the tables represents the relationship between each table and that is why it is called relational in some way. In real life, we can say that each employee is assigned to one or more departments, etc.
What is a table schema?
Below is a sample “employees” table where there is a row for each employee’s details such as first name, last name, or email. As you can see also, each row has a fixed set of columns and all rows have the same schema. If you don’t want to assign a value to a particular column then we set its value to NULL. This makes an RDBMS table have a fixed schema.
From the table below, the first row has a NULL value to the reportsTo column (or field) which means that this employee record is not reporting to anyone as she is the President of the company.
What is SQL?
After you have designed it now comes the exciting part which is how we could get the information from our database. The process of getting the records that we are interested in is called querying. We used a language called SQL (or Structured Query Language) to retrieve information from our tables.
An example SQL is below which will retrieve row(s) from our employees table that has the last name ‘Murphy’
SELECT
lastname, firstname, jobtitle
FROM
employees
where lastname = 'Murphy';
Designing each table, field, and relationship requires complex analysis. Sounds confusing right? Hold on, we are not going to discuss so much about the relational database as we just need a baseline for us to understand MongoDB later.
What is MongoDB?
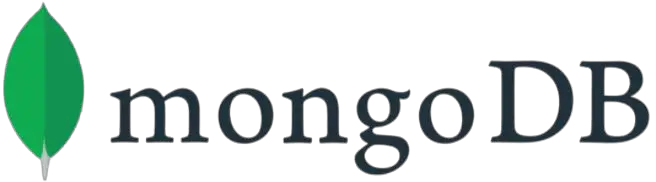
MongoDB is a type of NoSQL database touted as an alternative to the earlier RDBMS systems. If an RDBMS system is called a relational database then MongoDB is a NoSQL or non-relational database. NoSQL is sometimes called Not Only SQL.
NoSQL systems are also sometimes called Not only SQL to emphasize that they may support SQL-like query languages or sit alongside SQL databases in polyglot-persistent architectures
Wikipedia
These NoSQL databases are appropriate for Big Data and real-time systems such as capturing an enormous number of sensor readings.
RDBMS system follows the concept of tables, rows, and fix schema to represent information while MongoDB NoSQL database is schema-less and uses the concept of a document with key-value pairs similar in structure to JSON object in Javascript.
RDBMS | MongoDB (NoSQL) |
---|---|
Database | Database |
Table | Collection |
Row | Document |
Rows/Columns | Key-Value Pairs |
That looks like a lot of definitions so let us try to break it down to nail the concept.
What is JSON?
JSON or Javascript Object Notation is a file interchange format that uses human-like readable key-value pairs or even arrays mostly used in web applications and electronics exchange.
Below is a sample JSON object that represents information about a particular person. Can you see how easy it is to read what the following structure is showing? This makes JSON format human-readable as we can see and understand at once what message the structure is trying to convey.
{
"firstName": "John",
"lastName": "Smith",
"isAlive": true,
"age": 27,
"address": {
"streetAddress": "21 2nd Street",
"city": "New York",
"state": "NY",
"postalCode": "10021-3100"
},
"phoneNumbers": [
{
"type": "home",
"number": "212 555-1234"
},
{
"type": "office",
"number": "646 555-4567"
}
],
"children": [
"Catherine",
"Thomas",
"Trevor"
],
"spouse": null
}
From the JSON document, we have the following parts:
- “firstName”: “John” – is a sample key-value pair where firstName is the key and John is the value that it points to.
- “address”: {} – is what we call an embedded JSON object document.
- “phoneNumbers”: [] – is an array of JSON object
- “children”: [] – is an array that contains an individual string
When trying to interact with the MongoDB from our application then it is necessary to work with the JSON format.
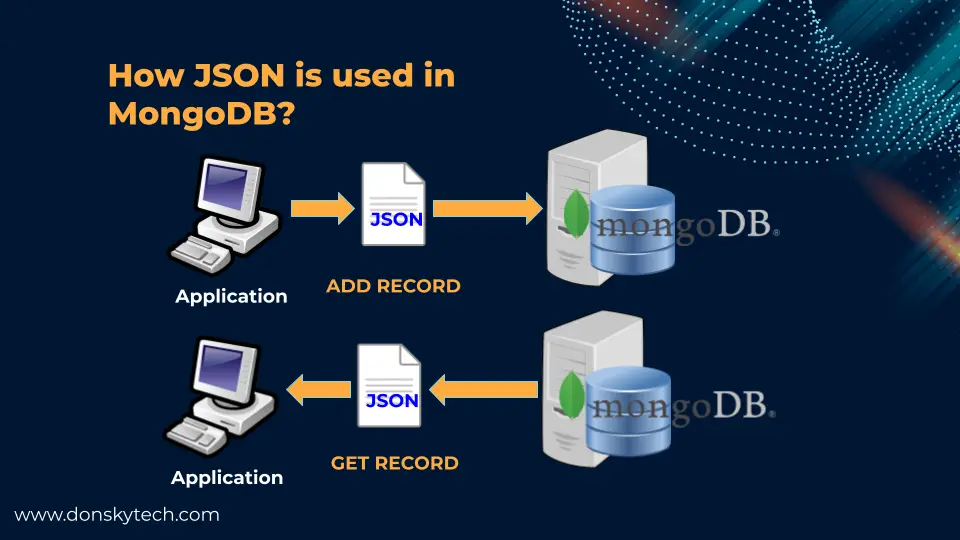
What I mean is, if you want to add a record to your MongoDB database then you would pass a JSON structure from your application. On the other hand, if you are retrieving information then you would be getting a JSON structure.
The application is any “client” that you will use to interact with the MongoDB database server. The next post of this series will show what a client is and we will create our own client as well.
What is a document?
A document is how MongoDB stores the record for each JSON object that we send it.
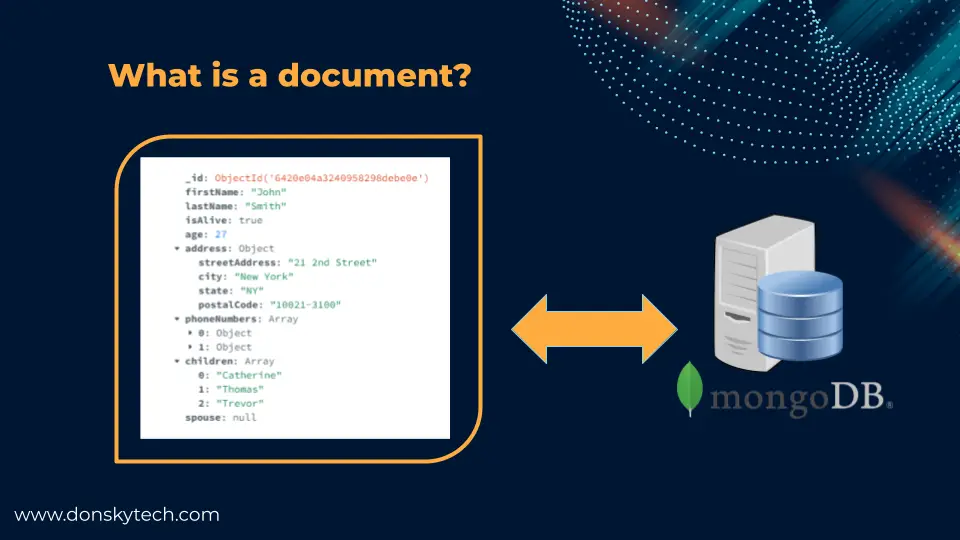
Our representation of the person above is stored in our MongoDB database in the following format.
- _id – is the Object ID that identifies a unique object in our database. Usually, this is auto-generated by the MongoDB server when we insert a row.
- firstName and other fields – are represented as string key-value pair
- address – is represented as an object
- phoneNumbers – are represented as an array of object
- children – are represented as an array
- spouse – has a null value which means the absence of any designation
Internally, MongoDB stores record in BSON format or the Binary encoded version of the JSON document.
What makes MongoDB schema-less?
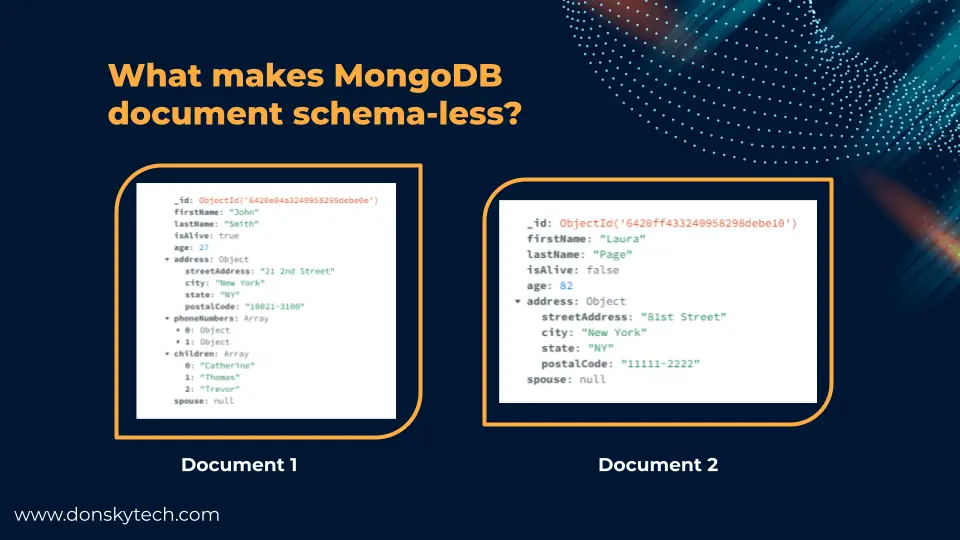
In the earlier discussion about the RDBMS table schema, I mentioned that it follows a rigid or fixed schema where each row has the same number of fields or columns. MongoDB on the other hand is a schema-less architecture.
The image above will show you where we have two documents that describe two unique people but the second document has no information for the children or phone numbers.
What is a MongoDB collection?
A MongoDB collection is similar to a table in RDBMS System. If the RDBMS table stores the record as a sequence of rows then a collection stores a series of documents inside it.
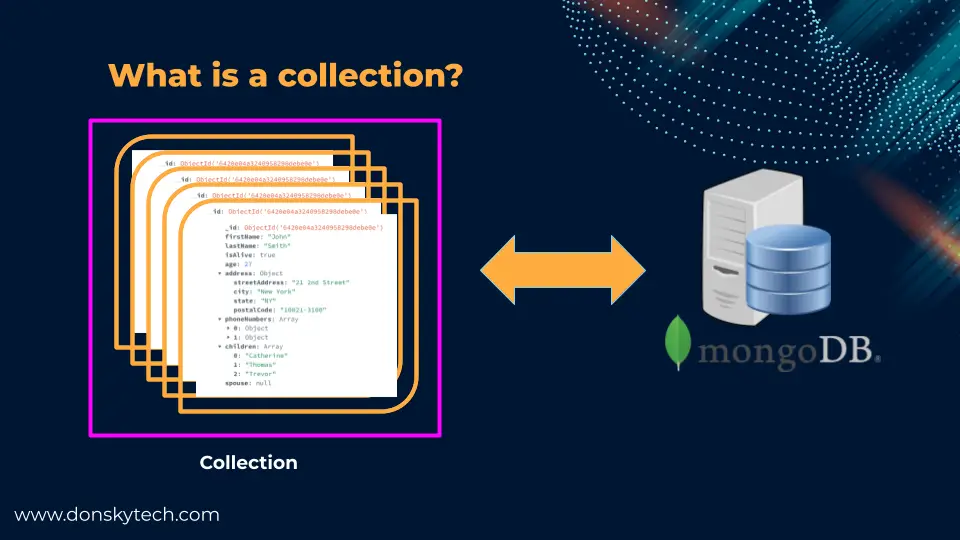
What is a MongoDB database?
A MongoDB database is composed of one or more collections. It is analogous to how an RDBMS database is composed of multiple tables.
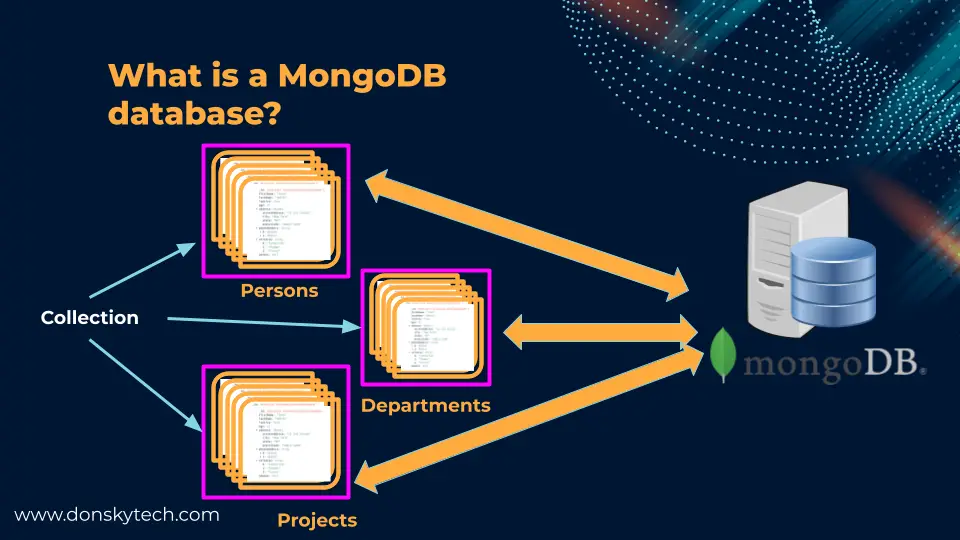
In the image above we can have a collection that stores Persons information, a Departments collection for department information, and a Projects collection that will store the list of projects that we are working on.
Of course, there are other objects we have not talked about like indexes and the like and they are part of the MongoDB ecosystem. I have just shown you the major components of the MongoDB database. If you would like to learn more about this database then take a look at the official documentation here.
Now that is a high-level overview of what MongoDB is and if you want to know more then you could start reading the official documentation. Next, we will discuss how we could get our hands on a MongoDB database server instance.
How to install the MongoDB database?
You can install a local instance of the MongoDB database in your workstation or laptop by using the Community Edition of this server. It is available in Windows, Linux, MacOS, and even docker installation. Visit the following page to check on the steps on how to install it on your specific platform.
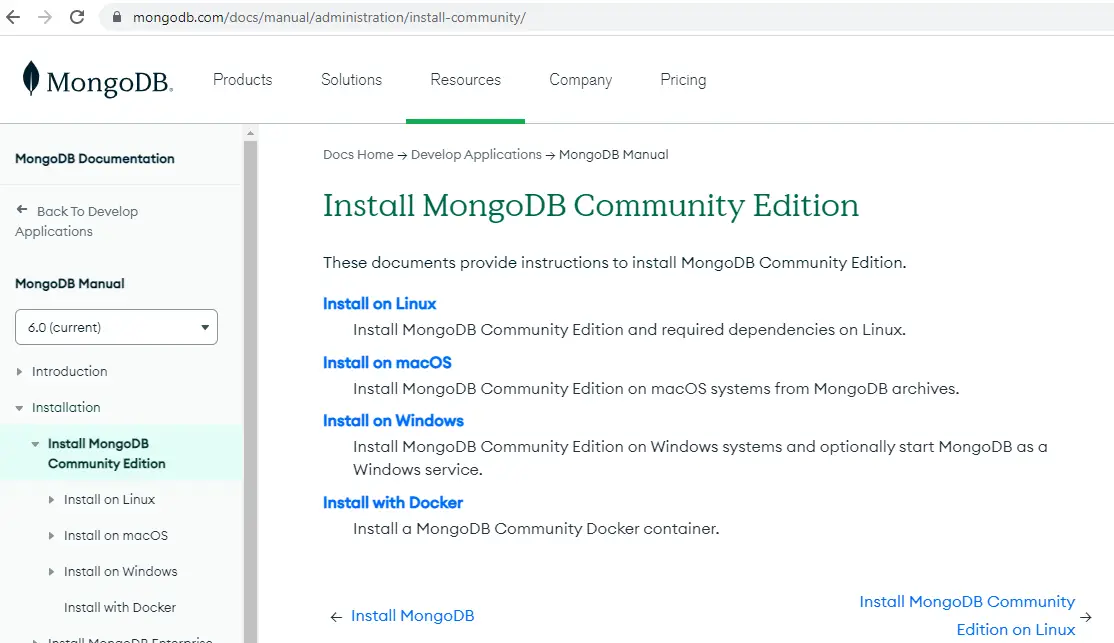
However, in this tutorial series, we will be using the cloud version of this database by using the free tier version of its MongoDB Atlas offering. If you are not familiar with a cloud database then it just means that the setup and maintenance are being done by the MongoDB company itself.
All we have to do is to sign up for a free tier version and we will get ourselves a running instance of a MongoDB database. The next post in this series will explain to you how to set up your own MongoDB Atlas database that you can play around with.
Arduino/Raspberry Pi/MicroPython with Database
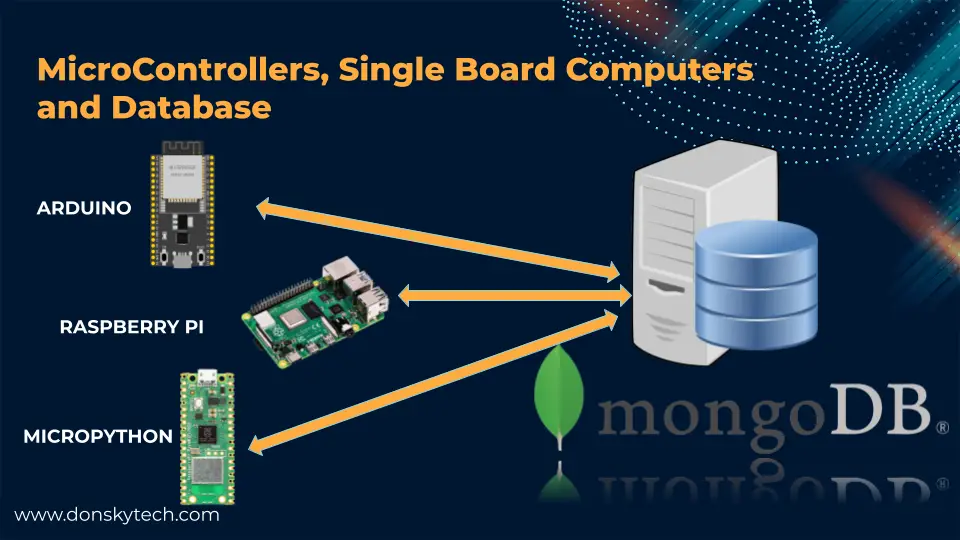
In the next posts of this tutorial series, we will explore how we can interface our Arduino boards, Raspberry Pi Single Board Computers (SBC), and MicroPython devices with the MongoDB database.
I will show you how you can control your physical electronic components with data coming from our MongoDB database.
Wrap Up
I have discussed an overview of how you can add the popular MongoDB NoSQL database in this post. I have introduced to you the differences between a traditional relation RDBMS and the MongoDB architecture. We came across the important objects in a MongoDB database system and how it relates to the traditional RDBMS system.
The next post in this series will discuss how you can have your own running instance of a MongoDB database on the cloud that you can use in your next IoT projects.
Until then! Happy exploring!
Leave a Reply