Introduction
If are you tired of using LCD (Liquid Crystal Display) screens in your Internet of Things (IoT) projects then please consider using OLED (Organic Light Emitting Diode) display. These OLED displays are capable of showing better text and support for image display. This post will show you how to interface your MicroPython-based project with these beautiful OLED displays.
If you want to see this project in a video format then please see below. You can also watch this on my YouTube channel.
Prerequisites
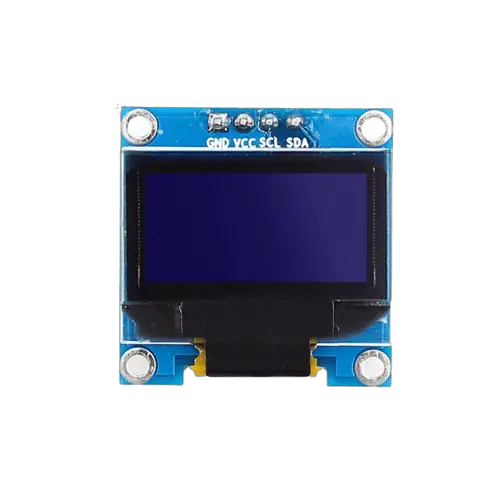
The following are the components needed to follow along with this post.
- ESP32 Dev Board – Amazon | AliExpress | Bangood
- SSD1306 OLED (I2C) – Amazon | AliExpress | Bangood
- Breadboard – Amazon | AliExpress | Bangood
- Jumper wires – Amazon | AliExpress | Bangood
Disclosure: These are affiliate links and I will earn small commissions to support my site when you buy through these links.
You should have installed the latest MicroPython firmware on your device and we are using the Thonny IDE as our development tool.
Please check the communication protocol of your OLED display as I am using an I2C (4 Pins) model. If your model is using SPI then the wiring/schematic layout might be different but the code will still work.
Related Content:
How to install MicroPython on ESP32 and download firmware
MicroPython Development Using Thonny IDE
What is an OLED display?
These colorful OLED displays come in different colors and you would most often see them in white or blue. They come in different sizes and resolutions with 128×64 and 128×32 as common variants. These OLEDs used in Arduino, Raspberry Pi, or other board microcontrollers and single-board computers (SBC) are often powered by the SSD1306 CMOS controller.
The following are the usual specifications for OLED displays on the market.
Operating Voltage | 3.3 – 5 V |
Size | 0.96 inches |
Resolution | 128×64 |
Number of Characters per Row | 21 |
Number of Rows | 7 |
Communication Protocols | SPI or I2C |
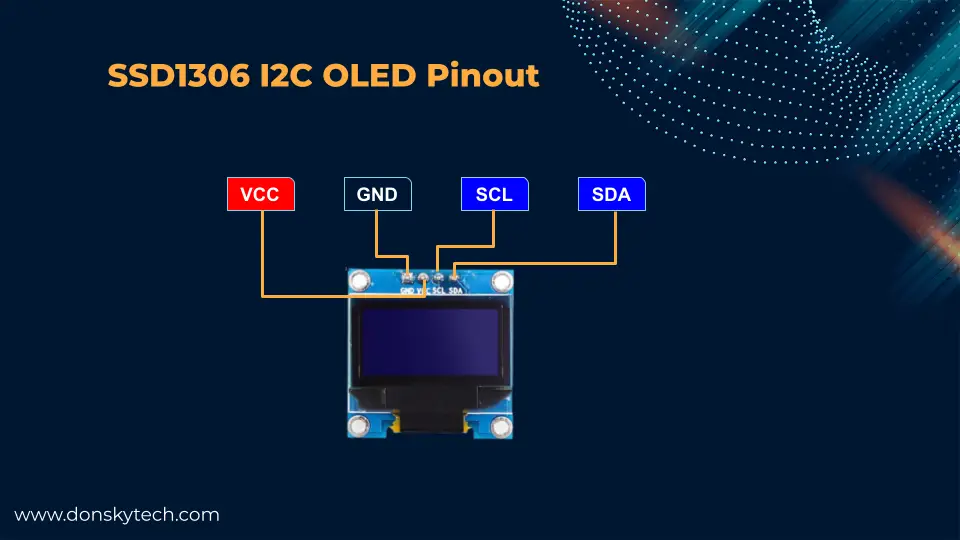
The image above is the usual pinout of an SSD1306 I2C OLED with the usual power pins VCC and Ground. The SCL and SDA pins are used for I2C communication protocol.
Wiring/Schematic
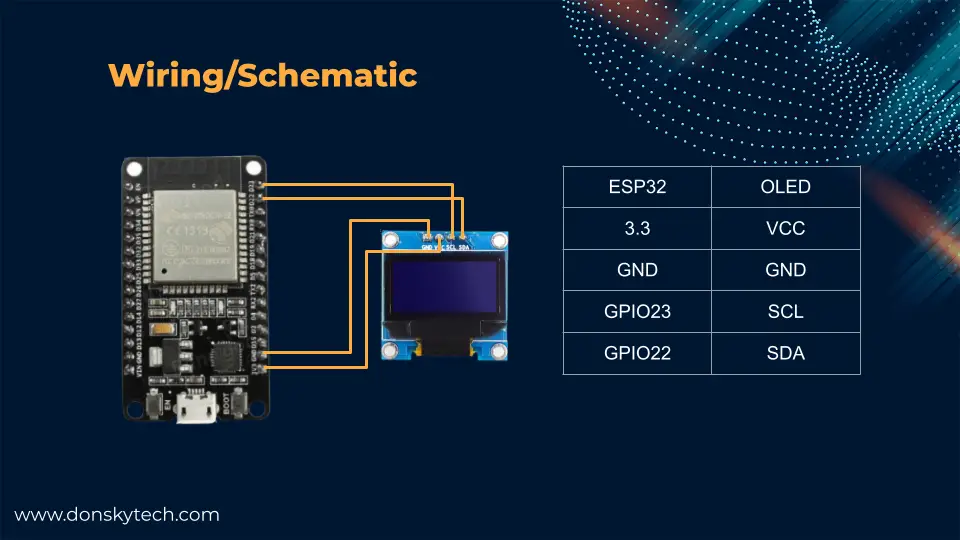
The image above is the wiring/schematic for this particular project. As we are using Software I2C in this project so we could use other GPIO pins that are available to you.
Interfacing MicroPython with SSD1306 OLED display
The complete code for this project is available in my GitHub repository. You can download the project as a zip file or clone it using the below command.
git clone https://github.com/donskytech/micropython-ESP32-ESP8266.git
Open the following in Thonny IDE to view each file and click the run button to know how it would work.
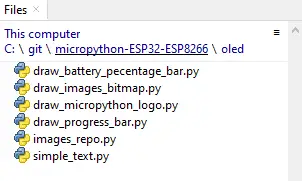
Install the SSD1306 MicroPython Library
Install the micropython-ssd1306 using the Thonny IDE. You can follow the below link if you do not know how to install libraries in MicroPython using Thonny IDE.
Related Content:
How to install MicroPython libraries or packages in Thonny IDE?
Understanding the SSD1306 OLED coordinates
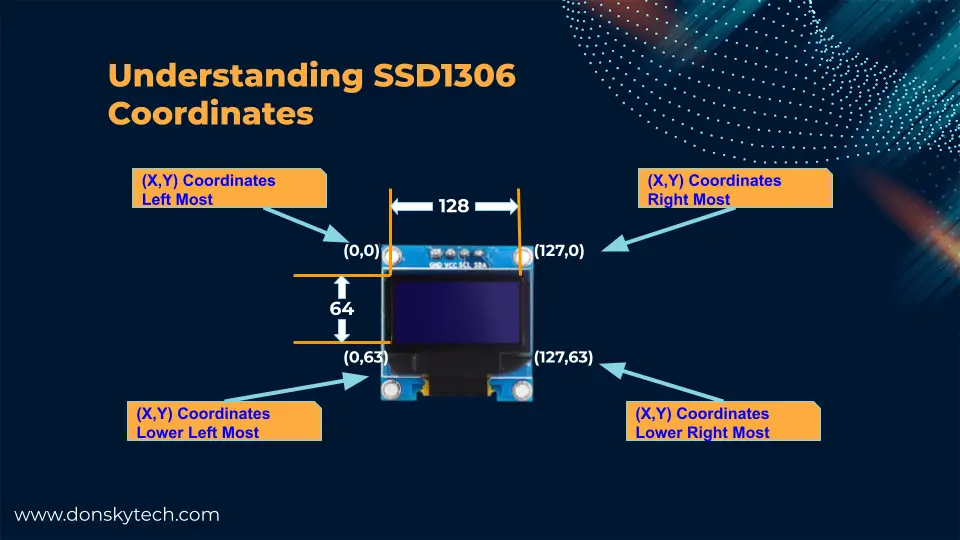
In the next sections to follow, you would see me talking about passing in the OLED display coordinates so I wanted to make it clear already before we proceed.
As you can see from the image above, I have a 0.96 Inch OLED with a 128X64 resolution where 128 is the width and 64 is the height. The coordinates start at the topmost left and are marked as (0,0) while the top right is marked as (127,0). The lower left-most coordinate is at (0,63) while the lower right-most is marked as (127, 63).
We will use this coordinate system later when we are programming where to place our text or graphics in an SSD1306 OLED.
How to draw text in SSD1306 using MicroPython?
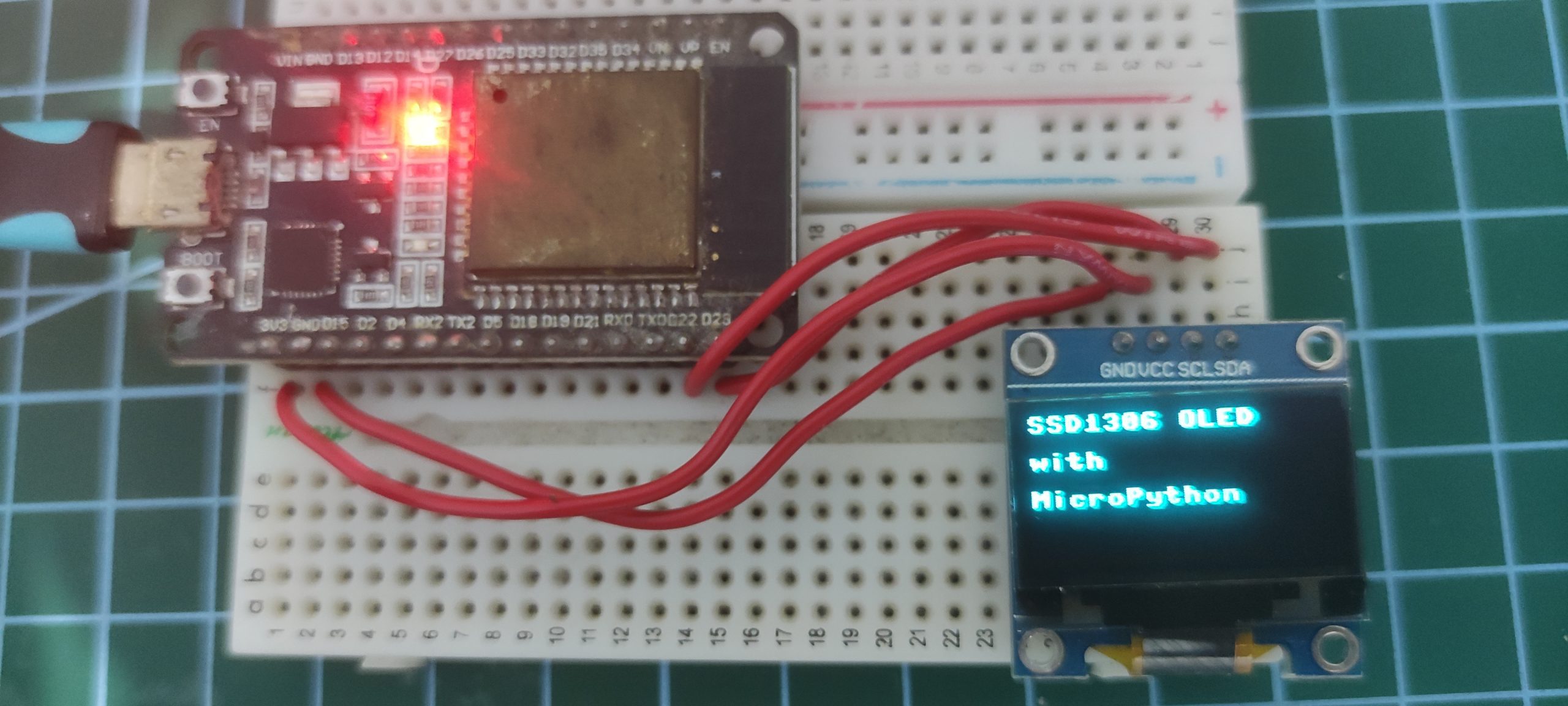
Writing text in an SSD1306 OLED is really simple and the below code is how you could display text into it.
from machine import Pin, SoftI2C
import ssd1306
SDA_PIN = 22
SCL_PIN = 23
# using SoftI2C
i2c = SoftI2C(sda=Pin(SDA_PIN), scl=Pin(SCL_PIN))
display = ssd1306.SSD1306_I2C(128, 64, i2c)
display.fill(0)
display.text('SSD1306 OLED', 0, 0)
display.text('with', 0, 16)
display.text('MicroPython', 0,32)
display.show()
Let us go through what each line of code does.
from machine import Pin, SoftI2C
import ssd1306
Import the necessary package to drive our MicroPython pins, SoftI2C, and ssd1306 library.
SDA_PIN = 22
SCL_PIN = 23
# using SoftI2C
i2c = SoftI2C(sda=Pin(SDA_PIN), scl=Pin(SCL_PIN))
display = ssd1306.SSD1306_I2C(128, 64, i2c)
Next, declare our GPIO pins that will serve as I2C SDA, SCL, and the SoftI2C
class by passing in our GPIO pins. We create an instance of the SSD1306_I2C
passing in the width and height of our OLED including our SoftI2C class.
display.fill(0)
display.text('SSD1306 OLED', 0, 0)
display.text('with', 0, 16)
display.text('MicroPython', 0,32)
display.show()
We first fill our OLED display with black using the fill(color)
method and then we call a series of text(text, x_coordinate, y_coordinate)
methods passing in the text to be displayed, the X coordinates starting with the left-hand corner, and the Y coordinate from topmost to bottom.
Also, notice from the methods above that the y_coordinate is adjusted to display the text on its own line. Finally, we call the show()
method so that it will be displayed by our SSD1306 OLED display. This is needed as the text or images we draw are first saved in a memory buffer and it only gets displayed when the show()
method is displayed.
How to draw graphic primitives in SSD1306 OLED using MicroPython?
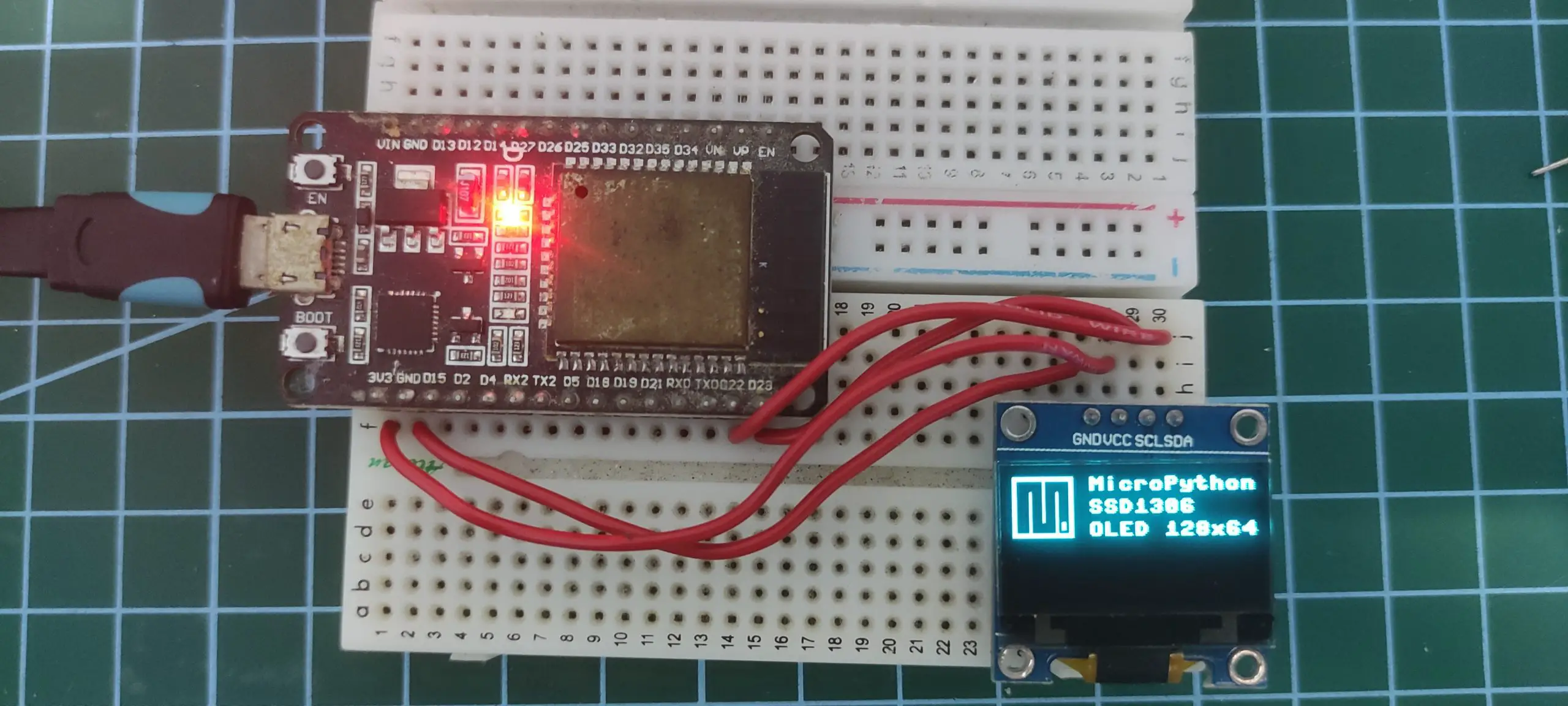
Aside from text, you can also draw simple primitive graphics on an SSID1306 OLED using MicroPython. This is done by executing methods on a FrameBuffer class.
Some of the more common methods for drawing graphic shapes are shown below. They are called primitive graphics because as you can see the shapes that you are drawing are simple pixels, lines, and rectangle shapes.
display.fill(0) # fill entire screen with colour=0
display.pixel(0, 10) # get pixel at x=0, y=10
display.pixel(0, 10, 1) # set pixel at x=0, y=10 to colour=1
display.hline(0, 8, 4, 1) # draw horizontal line x=0, y=8, width=4, colour=1
display.vline(0, 8, 4, 1) # draw vertical line x=0, y=8, height=4, colour=1
display.line(0, 0, 127, 63, 1) # draw a line from 0,0 to 127,63
display.rect(10, 10, 107, 43, 1) # draw a rectangle outline 10,10 to 117,53, colour=1
display.fill_rect(10, 10, 107, 43, 1) # draw a solid rectangle 10,10 to 117,53, colour=1
display.text('Hello World', 0, 0, 1) # draw some text at x=0, y=0, colour=1
display.scroll(20, 0) # scroll 20 pixels to the right
An example of drawing graphics is shown below which I have taken from the MicroPython site. The code below is a drawing of the MicroPython logo with some text.
display.fill(0)
display.fill_rect(0, 0, 32, 32, 1)
display.fill_rect(2, 2, 28, 28, 0)
display.vline(9, 8, 22, 1)
display.vline(16, 2, 22, 1)
display.vline(23, 8, 22, 1)
display.fill_rect(26, 24, 2, 4, 1)
display.text('MicroPython', 40, 0, 1)
display.text('SSD1306', 40, 12, 1)
display.text('OLED 128x64', 40, 24, 1)
display.show()
The best way to learn how this code works is by running it first and then seeing its output displayed on your SSD1306 OLED. Next, try commenting on all lines of code except for the display.show()
one and then try to uncomment each line of code starting from the top and run it one by one. You would appreciate how the graphics are being drawn on the OLED one by one and then you will have a clear understanding of how to use each method.
SSD1306 OLED Mini Projects
The best way to learn how to program this component and any other components is to create projects that will reinforce your understanding. Here I present to you two mini SSD1306 OLED mini projects to help you get comfortable programming this component.
Battery Progress Bar Display
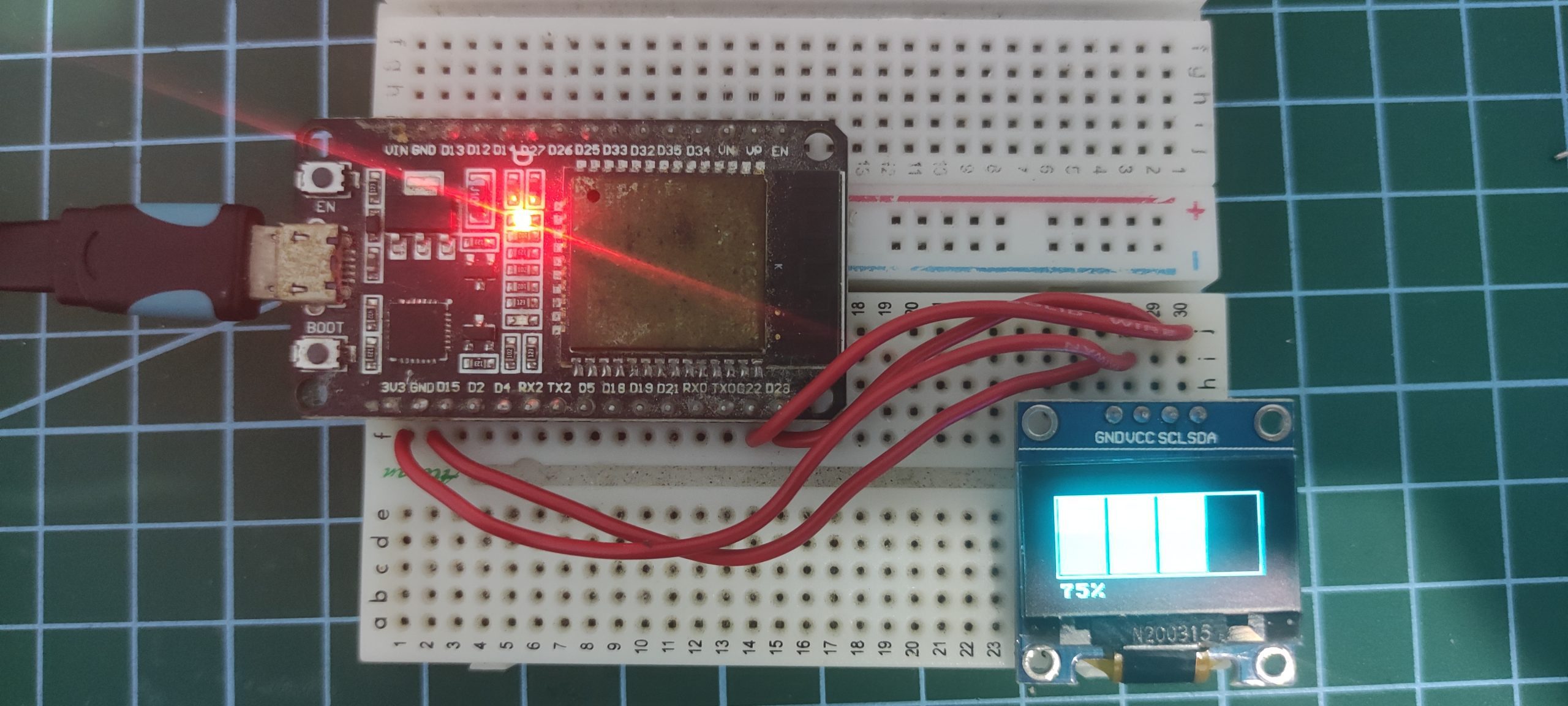
This project will show you the charge percentage of your battery storage displayed thru your SSD1306 OLED screen. Also, using only the knowledge about writing text and drawing primitives graphics then we would be able to accomplish this project.
Below is the code for our project.
from machine import Pin, SoftI2C
import ssd1306
import framebuf
import time
# using default address 0x3C
i2c = SoftI2C(sda=Pin(22), scl=Pin(23))
display = ssd1306.SSD1306_I2C(128, 64, i2c)
display.fill(0) # fill entire screen with colour=0
display.rect(10, 10, 107, 43, 1) # draw a rectangle outline 10,10 to width=107, height=53, colour=1
# draw battery percentage
x_pos = [12, 38, 64, 90]
percentages = [.25, .50, .75, 1.0]
while True:
for ctr in range(4):
display.fill_rect(x_pos[ctr],11,24,40,1)
display.fill_rect(0,56,128,40,0)
display.text("{:.0%}".format(percentages[ctr]), 11, 56)
display.show()
time.sleep_ms(1000)
# This will clear the battery charge percentage
for ctr in range(4):
display.fill_rect(x_pos[ctr],11,24,40,0)
display.show()
Let’s run through what each line of code does.
from machine import Pin, SoftI2C
import ssd1306
import framebuf
import time
Import the necessary packages and libraries needed to drive our SSD1306 OLED display using MicroPython.
# using default address 0x3C
i2c = SoftI2C(sda=Pin(22), scl=Pin(23))
display = ssd1306.SSD1306_I2C(128, 64, i2c)
We declare our SoftI2C
class that will handle our communication with the SSD1306 display. Next, we created an instance of SSD1306_I2C
class by passing in the width, height, and SoftI2C
object.
display.fill(0) # fill entire screen with colour=0
display.rect(10, 10, 107, 43, 1) # draw a rectangle outline 10,10 to width=107, height=53, colour=1
The first line will fill our display with black (0) and then we will draw the outer rectangle that will house our progress bar display.
# draw battery percentage
x_pos = [12, 38, 64, 90]
percentages = [.25, .50, .75, 1.0]
while True:
for ctr in range(4):
display.fill_rect(x_pos[ctr],11,24,40,1)
display.fill_rect(0,56,128,40,0)
display.text("{:.0%}".format(percentages[ctr]), 11, 56)
display.show()
time.sleep_ms(1000)
# This will clear the battery charge percentage
for ctr in range(4):
display.fill_rect(x_pos[ctr],11,24,40,0)
display.show()
This is the main logic of our program that will draw the battery percentage on our SSD1306 OLED display.
The x_pos
will tell the x coordinate for which the value of the progress bar will start while the percentages
will contain the value.
We define an infinite loop that will continually show and re-show our progress bar percentage. Inside the loop, we fill our outside rectangle will mini rectangles at specific x coordinates. Next, below it we display the progress bar value. This will run the loop from 25% to 100% and then back again to zero.
Finally, the last for loop will clear all the progress bars or mini rectangles that we have created initially so that only the outer rectangle is displayed.
How to draw an image in SSD1306 OLED using MicroPython?
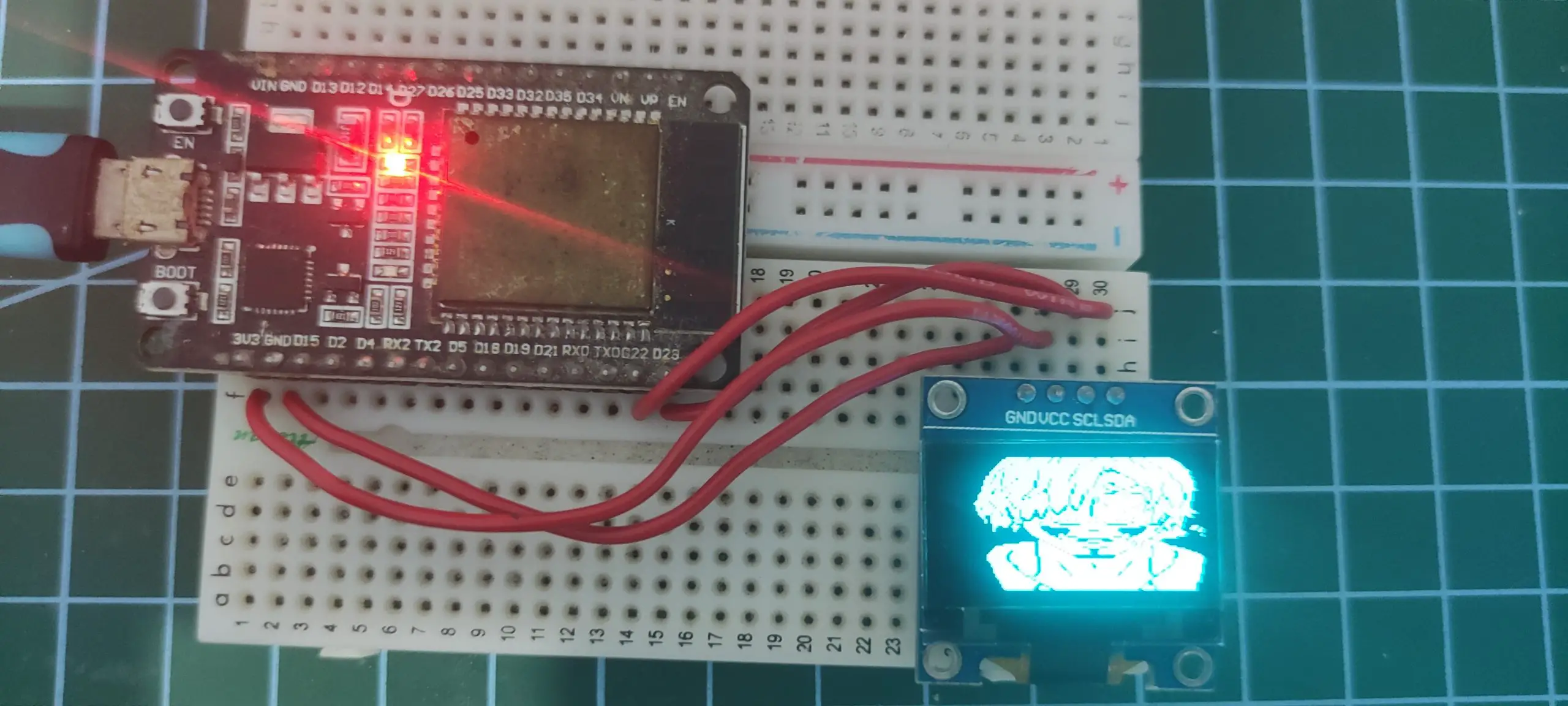
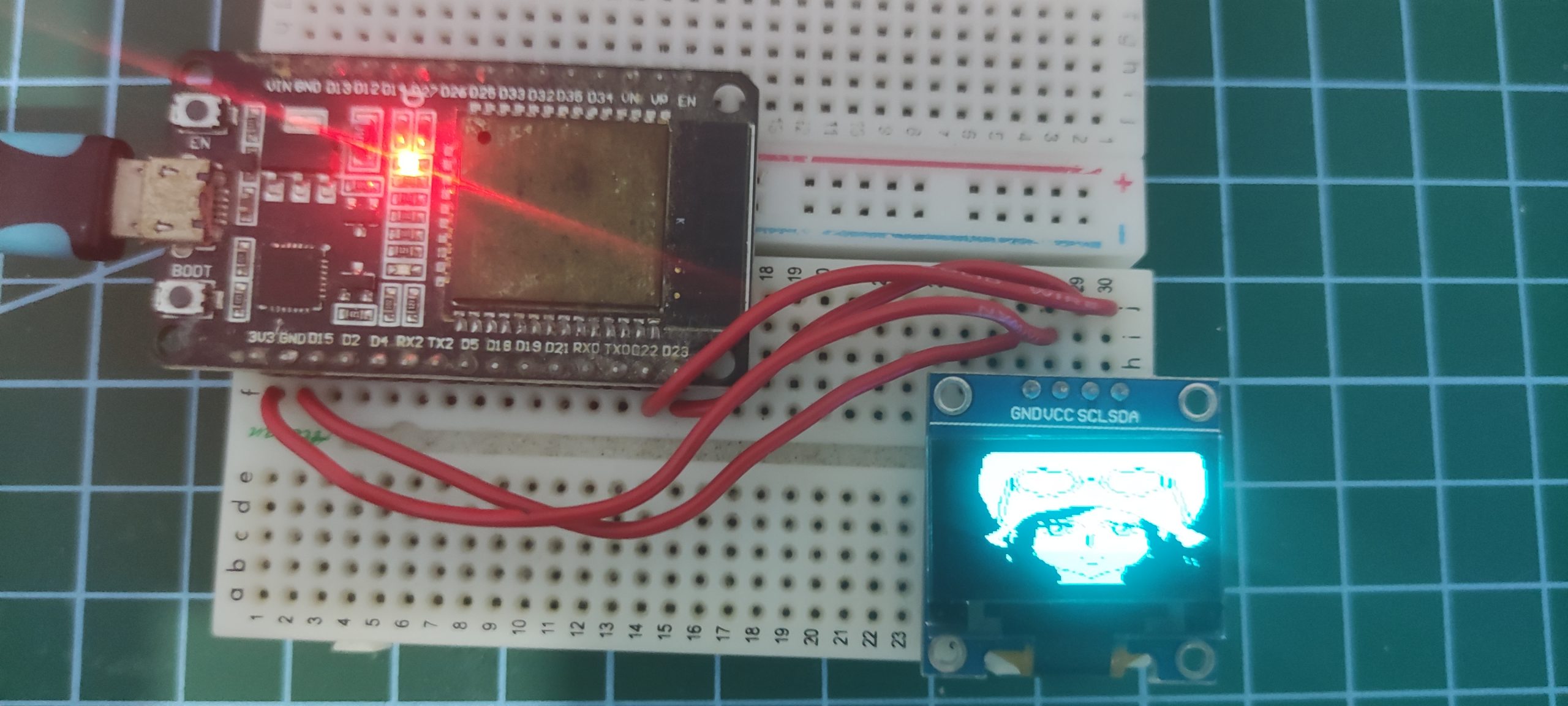
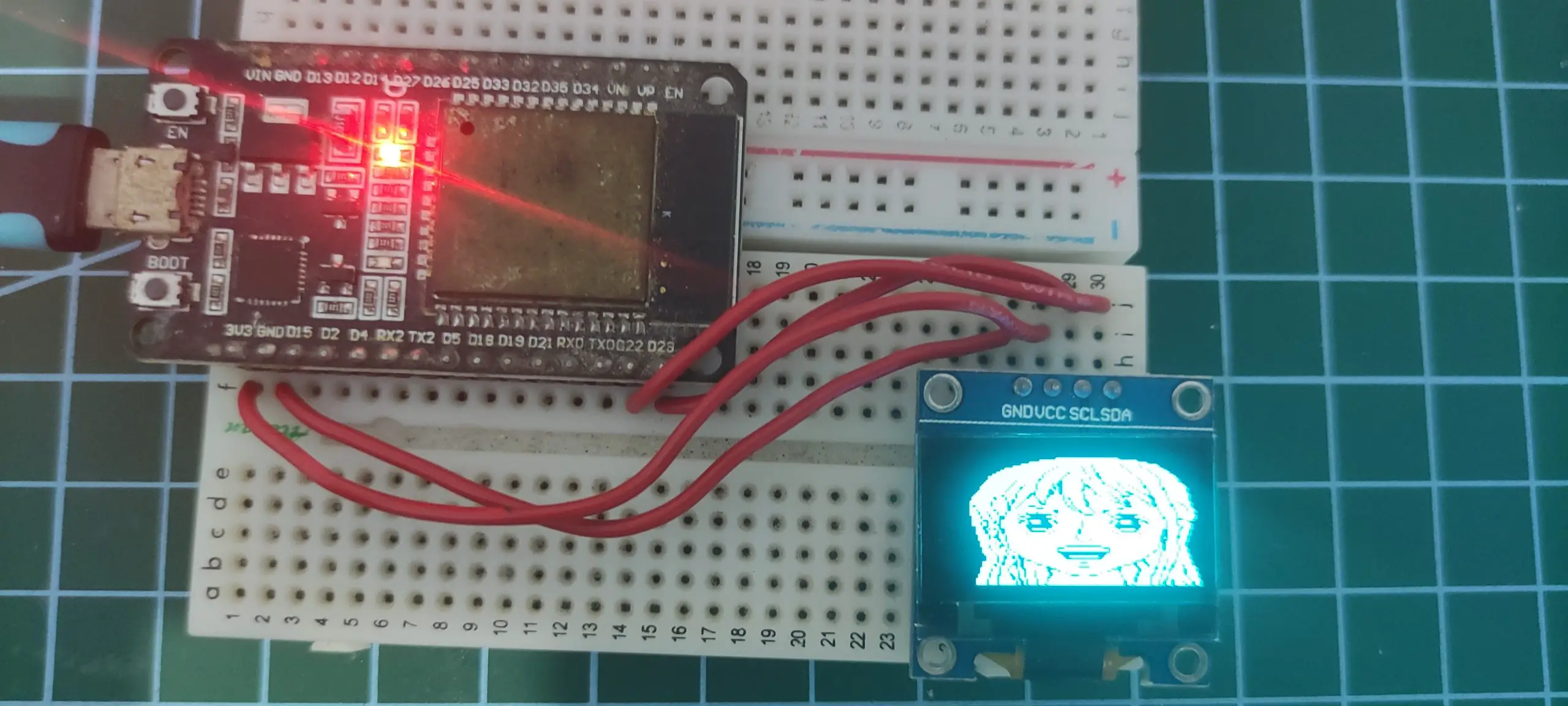
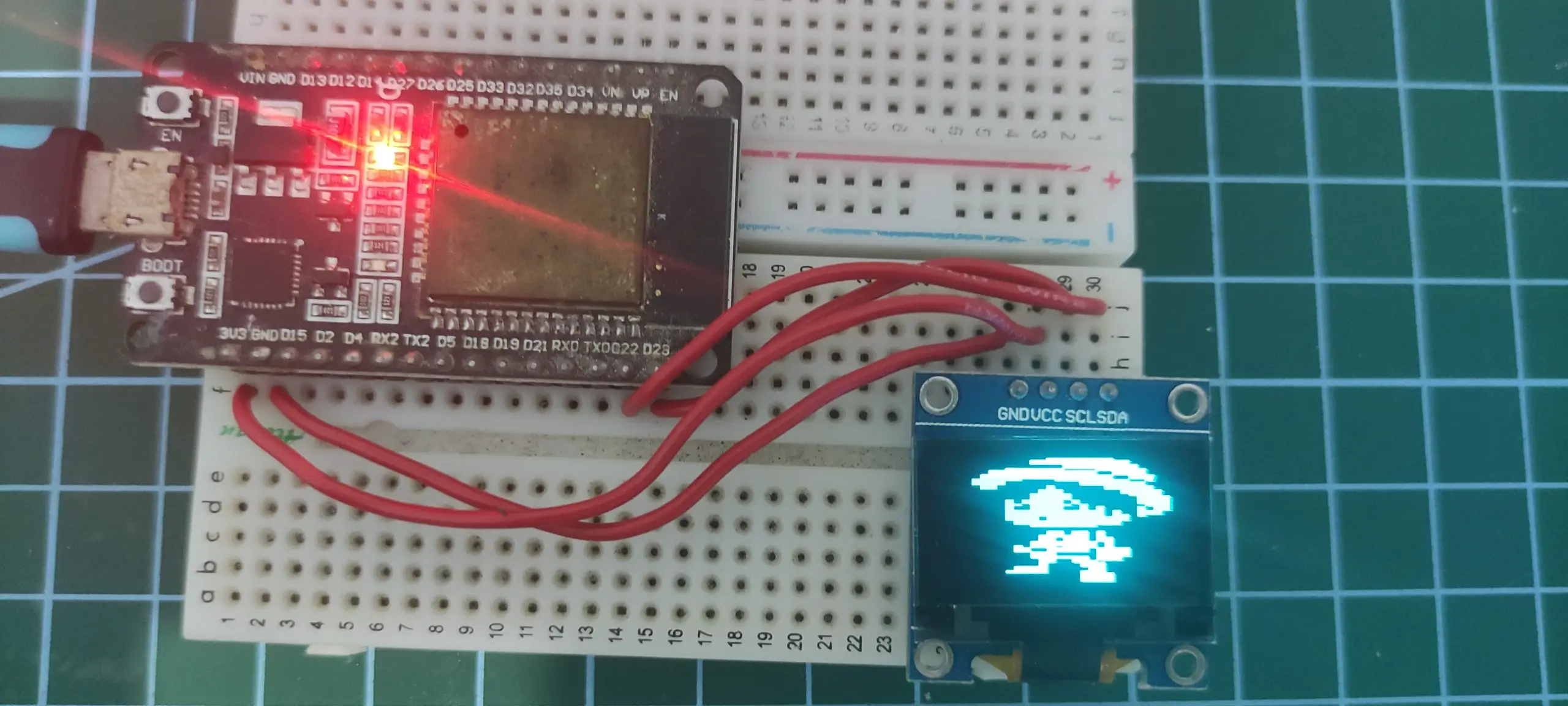
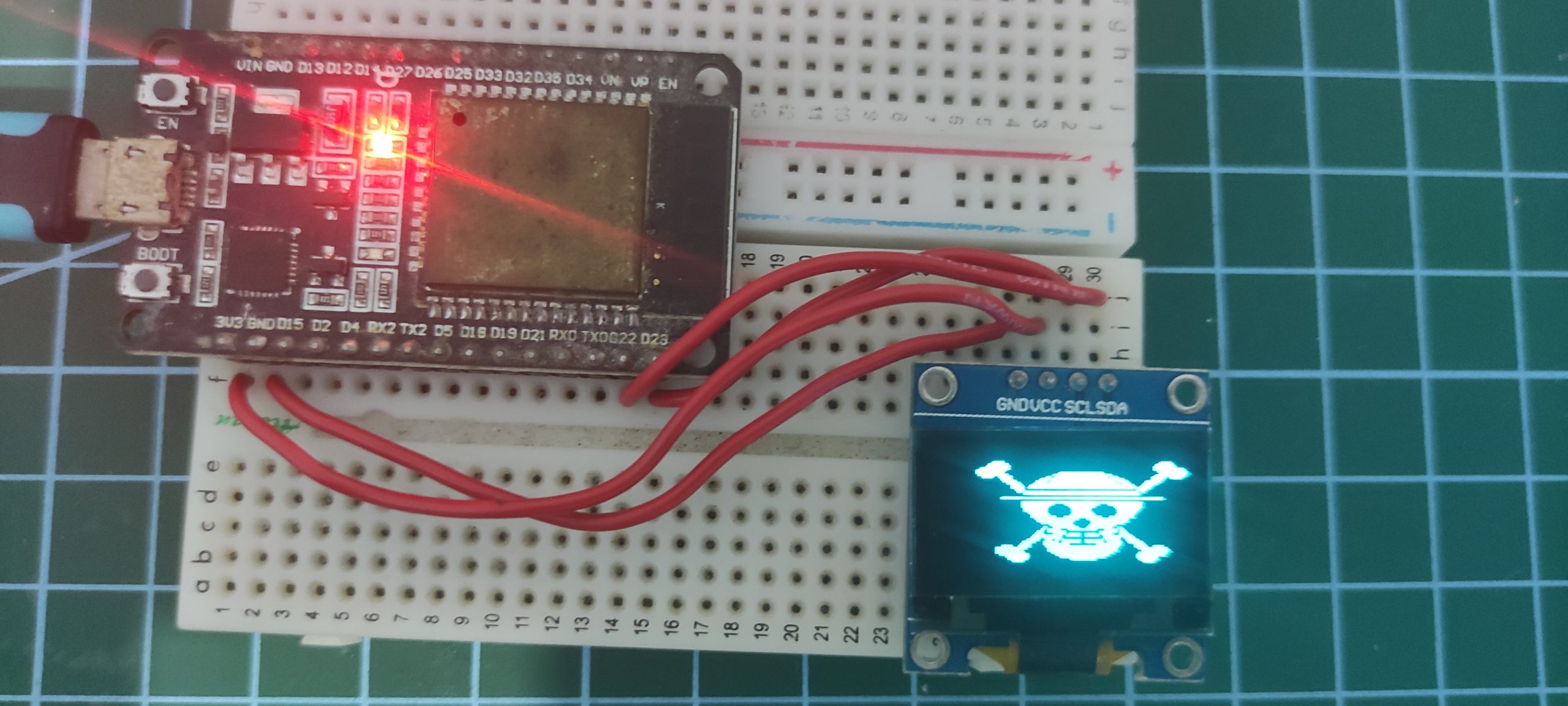
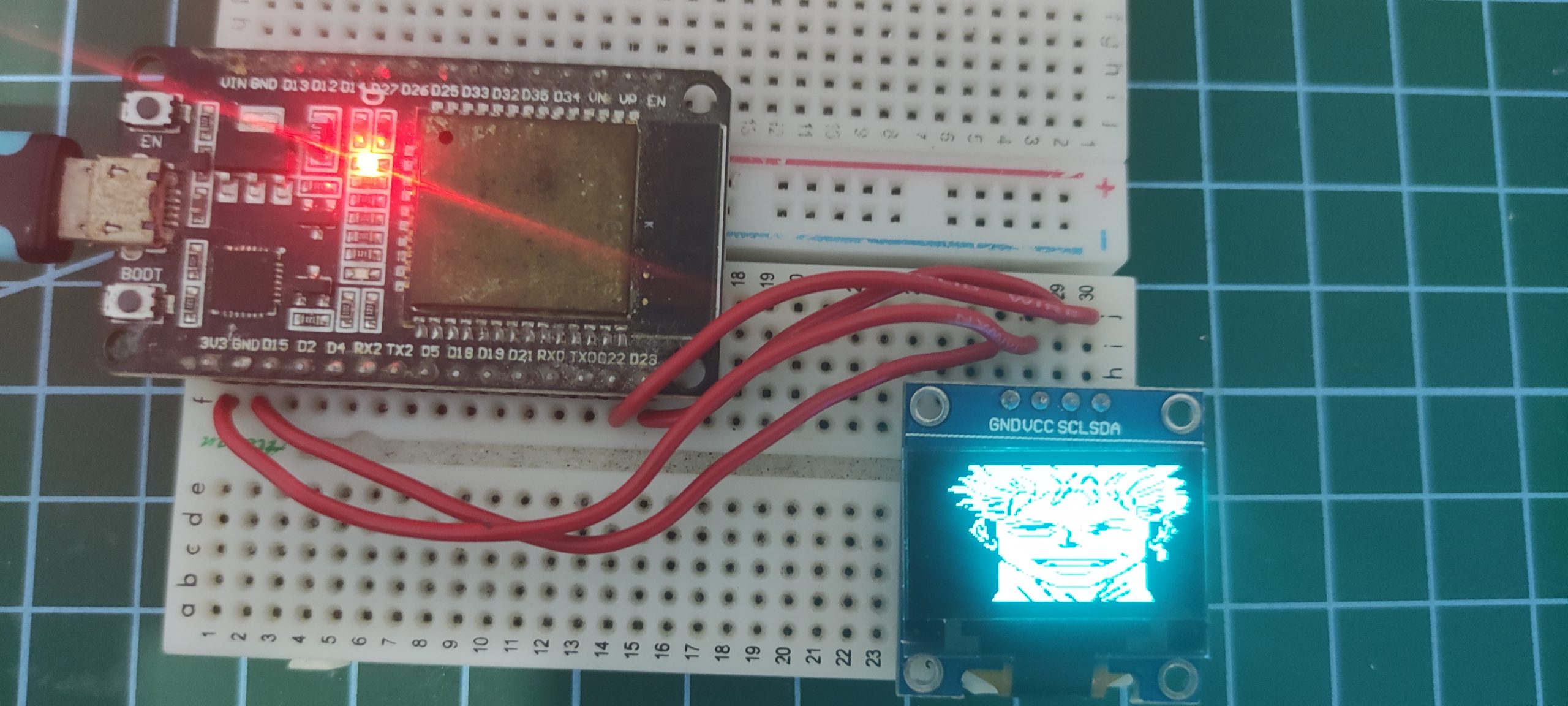
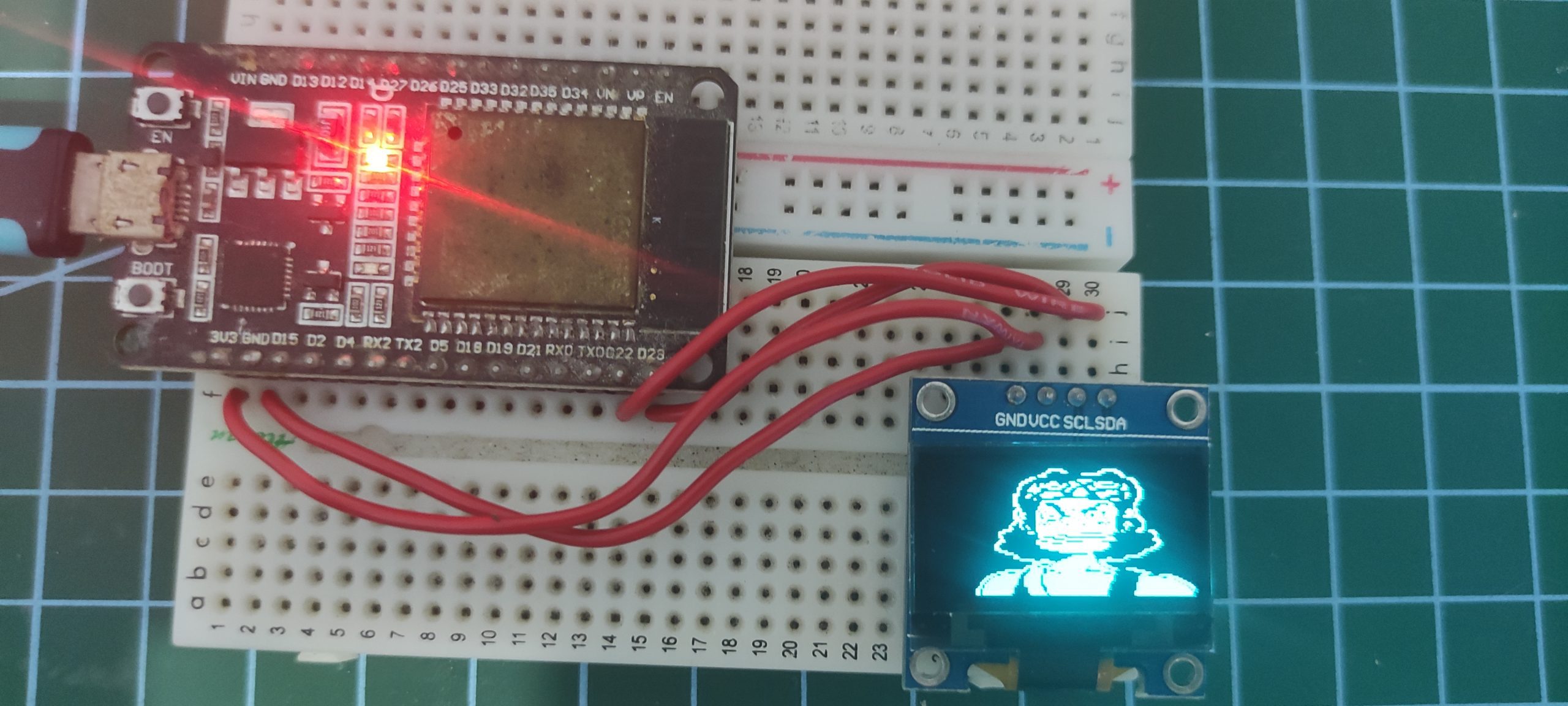
The next SSD1306 OLED mini project will show you how to display an image or bitmap in your SSD1306 OLED display using MciroPython code. I am a big fan of the One Piece Japanese anime since I was a child so I am showing you the pixel art of some of the characters of the said television program 🙂
The steps that I am going to show you are using the FrameBuffer class and I got inspiration reading the following post from the MicroPython forum. I made some manual steps and these may not be the best way but I just made it work. Let me know in the comment section below if you see another optimal way of doing this.
Step 1 – Download Images
I searched the internet for the images that I want to display. Look for the “pixel art” of the images as this is easy to display in SSD1306 OLED display. You can also create your own images using Adobe Photoshop, Gimp, or other photo editing software that supports pixel art.
Step 2 – Convert your images to byte array
Go to this site and upload your image and set the following configurations. You may have to do several trials and errors on images here as some images do not display perfectly well on the OLED.
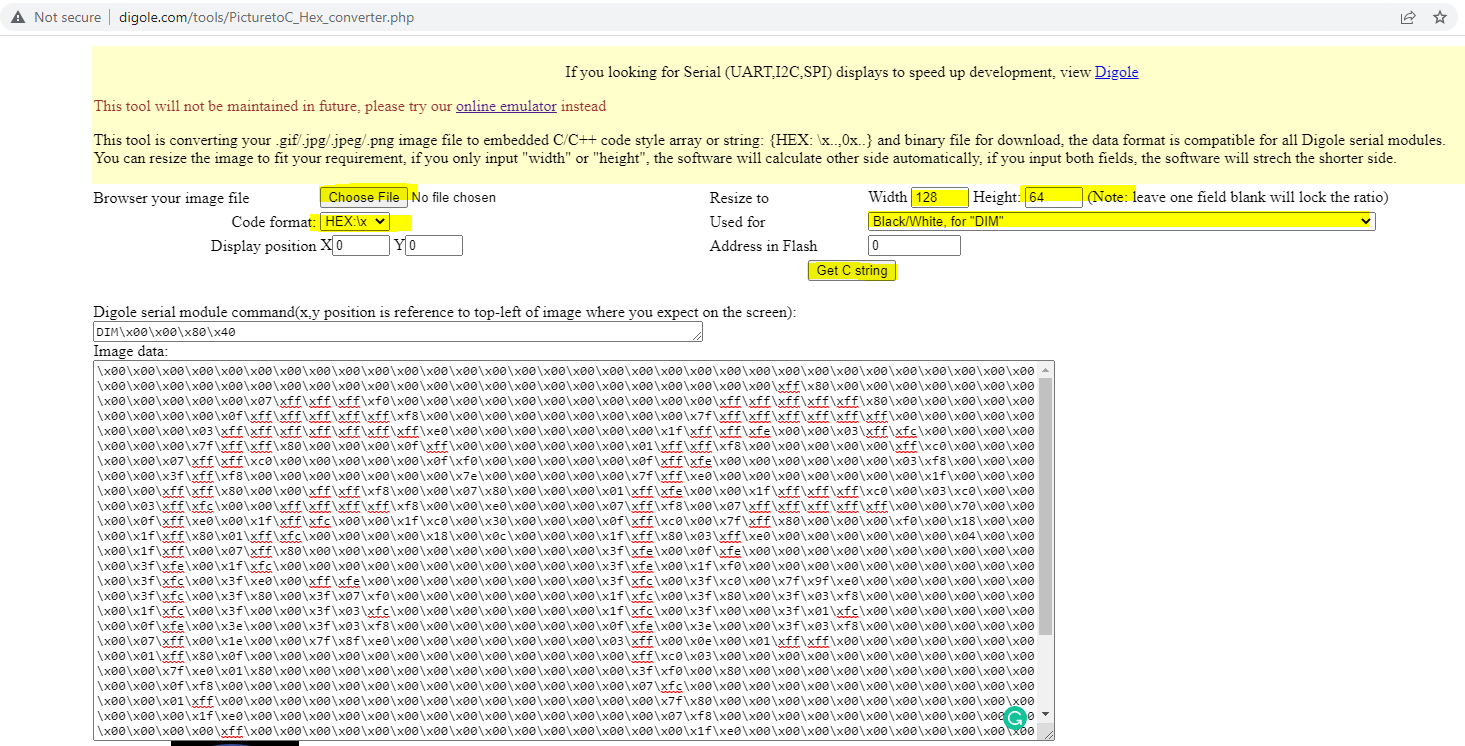
Another option for conversion is to use Python but I had issues converting images that are bigger than the 128×64 height and width sizes. Please see the post on the MicroPython forum on how this is done for the code.
Step 3: Code
List down all the byte arrays of all the images in a python file.
strawhat_jolly_roger = bytearray(b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\xf0\x00\x00\x00\x00\x00\x00\x00\x00\x00\xf8\x00\x00\x00\x00\x07\xfc\x00\x00\x00\x00\x00\x00\x00\x00\x03\xfc\x00\x00\x00\x00\x0f\xfe\x00\x00\x00\x00\x00\x00\x00\x00\x07\xfe\x00\x00\x00\x00\x7f\xfe\x00\x00\x00\x00\x00\x00\x00\x00\x07\xff\xe0\x00\x00\x01\xff\xf8\x00\x00\x00\x00\x00\x00\x00\x00\x01\xff\xf0\x00\x00\x01\xff\xf0\x00\x00\x01\xff\xff\xf0\x00\x00\x00\xff\xf8\x00\x00\x00\xff\xfc\x00\x00\x0f\xff\xff\xff\x00\x00\x03\xff\xf0\x00\x00\x00\x3e\x3f\x00\x00\x67\xff\xff\xfc\xe0\x00\x0f\xc7\xc0\x00\x00\x00\x00\x1f\x00\x03\xdf\xff\xff\xff\xbc\x00\x0f\x80\x00\x00\x00\x00\x00\x0f\xc0\x0f\x5f\xff\xff\xff\xaf\x00\x7f\x00\x00\x00\x00\x00\x00\x03\xf0\x1d\xbf\xff\xff\xff\xd3\x80\xfc\x00\x00\x00\x00\x00\x00\x00\xf8\x7f\xff\xff\xff\xff\xff\xe1\xf0\x00\x00\x00\x00\x00\x00\x00\xfc\xff\xff\xff\xff\xff\xff\xf7\xe0\x00\x00\x00\x00\x00\x00\x00\x3c\x00\x00\x00\x00\x00\x00\x07\x80\x00\x00\x00\x00\x00\x00\x00\x1b\xff\xff\xff\xff\xff\xff\xf9\x00\x00\x00\x00\x00\x00\x00\x00\x03\xff\xff\xff\xff\xff\xff\xfc\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x1f\xff\xff\xff\xff\xff\xff\xff\xff\xff\x80\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x07\xff\xff\xff\xff\xff\xff\xfe\x00\x00\x00\x00\x00\x00\x00\x00\x07\xff\xf0\x3f\xff\xc1\xff\xfe\x00\x00\x00\x00\x00\x00\x00\x00\x07\xff\xc0\x0f\xff\x00\x3f\xfe\x00\x00\x00\x00\x00\x00\x00\x00\x03\xff\x80\x07\xfc\x00\x1f\xfc\x00\x00\x00\x00\x00\x00\x00\x00\x03\xff\x80\x07\xfc\x00\x1f\xf8\x00\x00\x00\x00\x00\x00\x00\x00\x00\xff\x80\x07\xfe\x00\x1f\xf0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x7f\xc0\x0f\xff\x00\x3f\xe0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x7f\xf8\x7f\xff\xe1\xff\xe0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x1f\xff\xff\x0f\xff\xff\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x0f\xff\xfc\x03\xff\xff\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x03\xff\xf8\x03\xff\xfc\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x3f\xfc\x07\xff\xc0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\x8f\xff\x0f\xff\x18\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x03\x7e\x1f\xff\x87\xec\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x0e\x7f\xc0\x00\x3f\xee\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x1e\x0f\xdf\x9f\xbf\x0f\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x3f\xff\x3f\x9f\xcf\xff\xc0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x7d\xff\x00\x00\x0f\xf3\xe0\x00\x00\x00\x00\x00\x00\x00\x00\x01\xf8\xe6\xff\x9f\xf6\xf1\xf8\x00\x00\x00\x00\x00\x00\x00\x00\x07\xe1\xfc\xff\x9f\xf3\xf8\xfe\x00\x00\x00\x00\x00\x00\x00\x00\x0f\x81\xff\xe0\x00\x7f\xf8\x1f\x00\x00\x00\x00\x00\x00\x00\x1f\x1f\x80\xff\xff\xff\xff\xf0\x1f\x8f\x80\x00\x00\x00\x00\x00\xff\xfe\x00\x7f\xff\xff\xff\xe0\x07\xff\xe0\x00\x00\x00\x00\x00\xff\xf8\x00\x7f\xff\xff\xff\xe0\x01\xff\xf0\x00\x00\x00\x00\x00\xff\xfc\x00\x1f\xff\xff\xff\x80\x07\xff\xe0\x00\x00\x00\x00\x00\x3f\xff\x00\x07\xff\xff\xfe\x00\x0f\xff\xc0\x00\x00\x00\x00\x00\x07\xff\x00\x01\xff\xff\xf8\x00\x0f\xfc\x00\x00\x00\x00\x00\x00\x03\xfe\x00\x00\x0f\xff\x00\x00\x07\xfc\x00\x00\x00\x00\x00\x00\x00\xf8\x00\x00\x00\x00\x00\x00\x01\xf0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00')
luffy_image = bytearray(b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xff\xff\xff\xff\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xff\xff\xff\xff\xff\xff\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xff\xff\xff\xff\xff\xff\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xff\xff\xff\xff\xf0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xff\xff\xff\xff\xf0\x00\x00\x00\x00\x00\x00\x00\x0f\xff\xff\xff\x00\x00\xff\xff\xff\x00\x00\x00\x00\x00\x00\x0f\xff\xff\xff\xff\xff\xff\x00\xff\xff\x00\x00\x00\x00\x00\x00\x0f\xff\xff\xff\xff\xff\xff\x00\xff\xff\x00\x00\x00\x00\x00\x00\xff\xff\xff\xff\xff\xff\xff\xff\x00\xff\xf0\x00\x00\x00\x00\x00\xff\xff\xff\xff\xff\xff\xff\xff\x00\xff\xf0\x00\x00\x00\x00\x0f\xff\xff\xf0\x00\x0f\xff\xff\xff\xff\x00\xf0\x00\x00\x00\x00\x0f\xff\xf0\x00\x00\x00\x00\xff\xff\xff\xff\x00\x00\x00\x00\x00\x0f\xff\xf0\x00\x00\x00\x00\xff\xff\xff\xff\x00\x00\x00\x00\x00\x00\xff\x00\x00\x00\xf0\x00\x00\x0f\xff\xff\xf0\x00\x00\x00\x00\x00\xff\x00\x00\x00\xf0\x00\x00\x0f\xff\xff\xf0\x00\x00\x00\x00\x00\x00\x00\x00\x0f\xff\x00\x00\x00\x0f\xff\xff\x00\x00\x00\x00\x00\x00\x00\x00\x0f\xff\x00\x00\x00\x0f\xff\xff\x00\x00\x00\x00\x00\x00\x00\x00\xff\xff\xf0\x00\x00\x00\xff\xff\x00\x00\x00\x00\x00\x00\x00\x00\xff\xff\xf0\x00\x00\x00\x0f\xff\xf0\x00\x00\x00\x00\x00\x00\x00\xff\xff\xf0\x00\x00\x00\x0f\xff\xf0\x00\x00\x00\x00\x00\x0f\x0f\xff\xff\x0f\x00\x00\x00\x0f\xff\xf0\x00\x00\x00\x00\x00\x0f\x0f\xff\xff\x0f\x00\x00\x00\x0f\xff\xf0\x00\x00\x00\x00\x00\x0f\xf0\xff\xff\xf0\xf0\x00\x00\x00\xff\xf0\x00\x00\x00\x00\x00\x0f\x00\xff\xff\x00\xf0\xf0\x00\x0f\x0f\xf0\x00\x00\x00\x00\x00\x0f\x00\xff\xff\x00\xf0\xf0\x00\x0f\x0f\xf0\x00\x00\x00\x00\x00\x0f\xff\xff\xff\xff\xff\xf0\xf0\x0f\xff\x00\x00\x00\x00\x00\x00\x0f\xff\xff\xff\xff\xff\xf0\xf0\x0f\xff\x00\x00\x00\x00\x00\x00\x0f\xff\xff\xff\xff\xff\xf0\xff\x0f\x00\x00\x00\x00\x00\x00\x00\x0f\xff\xf0\xff\xff\xff\xff\xff\x00\x00\x00\x00\x00\x00\x00\x00\x0f\xff\xf0\xff\xff\xff\xff\xff\x00\x00\x00\x00\x00\x00\x00\x00\x00\xff\xff\xff\xff\xff\xff\xf0\x00\x00\x00\x00\x00\x00\x00\x00\x00\xff\xff\xff\xff\xff\xff\xf0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xff\xff\xff\xff\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xff\x0f\xf0\xf0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xff\x0f\xf0\xf0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x0f\xff\xff\xf0\xf0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x0f\xf0\xff\xf0\xff\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x0f\xf0\xff\xf0\xff\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xff\xff\xff\xff\x0f\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xff\xff\xff\xff\x0f\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xf0\xff\xf0\xff\xff\x0f\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xff\x00\xff\xff\xf0\xff\xff\x00\x00\x00\x00\x00\x00\x00\x00\x00\xff\x00\xff\xff\xf0\xff\xff\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xff\xf0\x00\x00\xff\xff\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xff\xf0\x00\x00\xff\xff\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xff\xff\xff\xf0\xff\xf0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x0f\xf0\x0f\xf0\xff\xf0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x0f\xf0\x0f\xff\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x0f\x00\x00\xff\xf0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x0f\x00\x00\xff\xf0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xff\xff\x00\x00\x0f\xf0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xff\xff\x00\x00\x0f\xf0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x0f\xff\xff\x00\x00\xff\xf0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xff\xff\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xff\xff\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xff\xff\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xff\xff\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00')
ussop = bytearray(b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x3f\x80\x00\x0f\xe0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x60\xe0\x00\x38\x38\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\x80\x00\x3f\xe0\x0c\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x07\x80\x00\x00\x00\x06\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x70\x00\x00\x00\x00\x00\xe0\x00\x00\x00\x00\x00\x00\x00\x00\x01\xc0\x00\x00\xfe\x00\x00\x30\x00\x00\x00\x00\x00\x00\x00\x00\x07\x00\x1c\x0e\x30\xbf\x80\x18\x00\x00\x00\x00\x00\x00\x00\x00\x0c\x13\x7e\x67\x80\xe0\x32\x08\x00\x00\x00\x00\x00\x00\x00\x00\x08\x33\xe1\xc6\x06\x7f\xe3\x0c\x00\x00\x00\x00\x00\x00\x00\x00\x18\x7c\x3f\x18\xe3\x80\x07\x06\x00\x00\x00\x00\x00\x00\x00\x00\x10\xfc\x00\x31\xf0\x03\xa0\x06\x00\x00\x00\x00\x00\x00\x00\x00\x10\x10\xff\x03\xf8\x7f\xe1\x86\x00\x00\x00\x00\x00\x00\x00\x00\x70\xc3\xff\x83\xf0\x3e\x01\x86\x00\x00\x00\x00\x00\x00\x00\x00\x61\x81\xf0\x00\x00\x18\x01\x06\x00\x00\x00\x00\x00\x00\x00\x00\x60\x10\x00\xff\xf8\x00\xf0\x0c\x00\x00\x00\x00\x00\x00\x00\x00\x60\x01\xff\xff\xff\xfe\x00\x08\x00\x00\x00\x00\x00\x00\x00\x00\x60\x03\x07\xff\xff\x81\x80\x18\x00\x00\x00\x00\x00\x00\x00\x00\x70\x03\x81\xff\xff\x1d\x80\x10\x00\x00\x00\x00\x00\x00\x00\x00\x10\x07\xf0\xff\xf8\x7f\xc0\x10\x00\x00\x00\x00\x00\x00\x00\x00\x38\x07\xf8\xd9\xc0\x3f\xe0\xd8\x00\x00\x00\x00\x00\x00\x00\x00\x68\x0b\x7c\x3f\x87\xe3\xe1\xe8\x00\x00\x00\x00\x00\x00\x00\x00\x6e\x0d\xfe\x3f\xec\xf7\xe0\xe8\x00\x00\x00\x00\x00\x00\x00\x00\x6c\x0d\xc7\x07\xec\xf7\xc3\xdc\x00\x00\x00\x00\x00\x00\x00\x00\x76\x0c\xfe\x7d\xf7\xdb\xc0\x06\x00\x00\x00\x00\x00\x00\x00\x00\x38\x0f\x82\xff\x7f\xff\xc0\x03\x00\x00\x00\x00\x00\x00\x00\x00\x6c\x0d\xbf\x7f\xff\x84\xc1\x81\x00\x00\x00\x00\x00\x00\x00\x00\xc8\x0a\x5f\xcf\xcf\xdb\xc2\xc1\x00\x00\x00\x00\x00\x00\x00\x01\x09\x0f\xff\xff\xff\xff\xc1\x01\x00\x00\x00\x00\x00\x00\x00\x01\x84\x8f\xff\xff\xff\xff\xc1\x07\x00\x00\x00\x00\x00\x00\x00\x0f\x00\x0f\xff\xff\xff\xff\xa4\x0e\x38\x00\x00\x00\x00\x00\x00\x38\x00\xe7\xff\xff\x80\x0f\x80\x00\x0c\x00\x00\x00\x00\x00\x00\x20\x00\x07\xfc\x1f\xff\xfc\x00\x00\x04\x00\x00\x00\x00\x00\x00\x30\x00\x06\x07\xc0\x00\x03\x80\x00\x04\x00\x00\x00\x00\x00\x00\x10\x00\x03\xf0\x3f\xff\xff\xc0\x00\x0e\x00\x00\x00\x00\x00\x00\x38\x00\x0c\x3f\xc0\x1f\xe0\x00\x00\x03\x00\x00\x00\x00\x00\x00\x60\x00\x0f\xe0\x7f\xff\xfe\x00\x00\x03\x00\x00\x00\x00\x00\x00\xc0\x00\x03\xff\xff\xff\xfe\x00\x00\x06\x00\x00\x00\x00\x00\x00\xc0\x00\x03\xff\xff\xff\xfc\x00\x00\x1c\x00\x00\x00\x00\x00\x00\xe0\x00\x00\x3f\xff\xff\xf0\x00\x00\xf0\x00\x00\x00\x00\x00\x00\x3c\x00\x00\x01\xff\xfe\x00\x00\x01\x80\x00\x00\x00\x00\x00\x00\x07\x80\x00\x00\x00\x06\x00\x00\x07\x00\x00\x00\x00\x00\x00\x00\x00\x60\x00\x00\x07\xff\x00\x01\xfc\x00\x00\x00\x00\x00\x00\x00\x00\x70\x00\x00\x1f\xff\x03\x03\x00\x00\x00\x00\x00\x00\x00\x00\x00\x0f\xe3\xc0\xff\xff\xbf\xfc\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x3f\xfb\xff\xff\xb8\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x3c\x2f\xff\xff\xdc\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\xf3\xdf\xff\xfb\xf7\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x03\x7e\x7f\xff\xf7\xfc\xe1\xf0\x00\x00\x00\x00\x00\x00\x00\x07\xfe\x7e\x47\xff\xe0\x1f\x80\x1f\x00\x00\x00\x00\x00\x00\x00\x7c\x38\xfe\x7c\xff\xff\xf0\xc0\x01\xe0\x00\x00\x00\x00\x00\x03\x9f\xf1\xfd\xff\xff\xff\xff\x80\x03\x81\xf8\x00\x00\x00\x00\x0e\xff\xf3\xfd\xff\xff\xff\xff\x80\x07\xff\x1f\x00\x00\x00\x00\x1b\xff\xe3\xfd\xff\xff\xff\xff\x80\x07\xff\xfd\xc0\x00\x00\x00\x37\xff\xe3\xfd\xff\xff\xff\xff\x00\x07\xff\xff\x70\x00\x00\x00\x6f\xff\xe3\xfd\xff\xff\xff\xfe\x00\x0f\xff\xff\xdc\x00\x00\x00\x5f\xff\xc3\xfc\xff\xff\xff\xfe\x00\x0f\xff\xff\xf6\x00\x00\x00\xdf\xff\x80\xfe\x7f\xff\xff\xfe\x00\x0f\xff\xff\xfb\x00\x00\x01\xbf\xff\x80\xfe\x7f\xff\xff\xfc\x00\x0f\xff\xff\xfd\x80\x00\x01\xbf\xff\x80\xff\x7f\xff\xff\xfc\x00\x1f\xff\xff\xfe\xc0\x00\x01\xbf\xff\x00\x7f\xbf\xff\xff\xfc\x00\x1f\xff\xff\xfe\xc0')
zoro = bytearray(b'\x00\x01\xf3\xff\xff\x7f\xfc\xff\xff\xe7\xff\xff\xf8\x7f\xf0\x00\x00\x01\xfd\xff\xff\x87\xff\x3f\xff\xff\xff\xff\xc7\xff\xe0\x00\x00\x00\x7d\xff\xff\xff\xff\xff\xff\xff\xff\xff\x3f\xff\x80\x00\x00\x00\x3e\xff\xff\xff\xff\xff\x9f\xbf\xfc\xdf\xff\xff\x00\x00\x00\x00\x7f\x3f\xff\xff\xfd\xff\x1f\x7f\xf9\x3f\xfc\xfc\x00\x00\x00\x03\xff\xff\xff\x7f\xfe\xff\x3c\x1f\xe6\xff\xe3\xfc\x00\x00\x00\x3c\xff\xff\xe7\xdf\xff\x3e\xf8\xcf\xcd\xff\x9f\xff\xe0\x00\x00\x0f\x9f\xff\xfb\xf7\xff\x99\xf9\xc7\xbd\xfe\x7f\xe7\xfc\x00\x00\x07\xfd\xff\xc9\xfd\xff\xc9\xf3\xe7\x33\xfc\xff\x9f\xfc\x00\x00\x03\xff\xff\xe7\x7d\xff\xff\xff\xf7\x7f\xf9\xfe\x7f\xf0\x00\x01\xfd\xff\xe7\xf9\xbf\xff\xdc\xff\xff\xff\xf3\xf1\xf7\xc0\x00\x07\xff\x7f\xf8\x7f\xfd\xff\xdc\x4b\xcf\xfb\xe7\xcf\x0f\x80\x00\x00\xff\xbf\xff\x8c\x1d\xf3\x10\x93\xcb\xb2\xce\x3c\xff\xfe\x00\x00\x03\xf7\xff\xf9\xf1\xcf\x33\xfd\xe7\x99\xcf\xe3\xff\xc0\x00\x00\x00\xfc\xff\xf7\xfd\xdf\x67\xfe\xf7\xdd\xf7\x1f\x87\xfe\x00\x00\x00\x7f\x81\xef\xf7\x3e\xe7\xff\x7b\xf1\xf9\xff\xff\xff\xc0\x00\x0f\xf8\x7f\xcf\xe5\xfe\xdf\xff\xa7\xf2\x79\xff\x87\xff\x00\x00\x1f\xff\xe0\xcf\xe7\xfd\x1f\xff\x67\xfe\x79\xff\x3f\xfe\x00\x00\x70\x7f\x03\xcf\x9f\xfc\x7f\xfe\x5f\xfe\x79\xf8\xff\xfe\x00\x00\x04\x7f\x1f\x9f\x7f\xff\xff\xff\xff\xff\x3e\x07\x3f\xfc\x00\x00\x01\xff\xff\x9c\xff\xff\xff\xff\xff\xff\x8e\xf8\x1f\xf0\x00\x00\x00\x06\x00\x98\xff\xff\xff\xff\xff\xff\xee\x80\x0f\x3e\x00\x00\x00\x07\xe0\x9b\xff\xff\xff\xff\xff\xff\xfe\xfc\x1e\x38\x00\x00\x00\x3f\xff\x93\xff\xff\xff\xff\xff\xff\xfe\xe1\xf0\xc0\x00\x00\x00\x03\xff\x7f\xff\xff\xff\xff\xff\xff\xff\x3f\xe7\x00\x00\x00\x00\x0c\x70\xff\xc7\xff\xff\xff\xff\xc0\x3f\xc7\xc8\x00\x00\x00\x00\x3c\x0f\xf8\xf8\x3f\xff\xff\xfe\x3f\xff\xf8\x0e\x00\x00\x00\x00\x3c\x0f\x07\xff\xc1\xbf\xff\xf0\xff\xff\xf8\x0a\x00\x00\x00\x00\x64\x0f\xfe\x00\x00\x77\xf7\x00\x3f\xff\xf8\x03\x00\x00\x00\x00\x52\x0f\xe0\x00\x07\xe7\xff\xf0\x00\xff\xf8\x24\x00\x00\x00\x01\xd2\x0f\xff\xc1\xf9\xf9\xf7\xc0\x7f\x07\xf8\x24\xc0\x00\x00\x00\xde\x0f\x9f\xff\xfe\x7c\xfe\x07\xff\xff\xf8\x34\x80\x00\x00\x00\x4e\x0f\xef\xff\xf9\xfc\xff\xc3\xff\xff\xf8\x38\x00\x00\x00\x00\x27\x0f\xfc\x00\x03\xfc\xff\xff\xff\xff\xf8\x7a\x00\x00\x00\x00\x19\x07\xff\xff\xff\xf9\xff\xff\xff\xff\xf0\x44\x00\x00\x00\x00\x0c\x27\xff\xff\xff\xf9\xff\xff\xff\xff\xe1\x88\x00\x00\x00\x00\x00\x17\xff\xff\xff\xf8\x3f\xff\xff\xff\xe4\x00\x00\x00\x00\x00\x03\x17\xff\xff\xff\x88\x0f\xff\xff\xff\xe4\x60\x00\x00\x00\x00\x01\xf7\xff\xff\xff\xc0\x37\xff\xff\xff\xe7\xe0\x00\x00\x00\x00\x00\x67\xff\xff\xff\xff\xff\xff\xff\xff\xe1\xe0\x00\x00\x00\x00\x00\x07\xff\xff\xff\xff\xff\xff\xff\xff\xe0\x38\x00\x00\x00\x00\x00\x07\xff\xff\xff\xff\xff\xff\xfe\x7f\xe0\x78\x00\x00\x00\x00\x00\x03\xff\xff\xff\xff\xff\xff\xfe\x1f\xe0\x78\x00\x00\x00\x00\x00\x01\xff\xff\xff\xff\xff\xff\xff\xdf\xc0\x78\x00\x00\x00\x00\x00\x00\xff\xf0\x00\x00\x00\x00\x03\xff\x80\x60\x00\x00\x00\x00\x00\x02\x7f\xfc\x0f\xff\xff\xf8\x0f\xff\x00\x00\x00\x00\x00\x00\x00\x07\x9f\xfe\x38\xff\xff\x86\x3f\xf8\xe0\x00\x00\x00\x00\x00\x00\x07\xe7\xff\xc0\x00\x00\x00\xff\xf1\xe0\x00\x00\x00\x00\x00\x00\x07\xfb\xff\xff\xff\xff\xff\xff\xc7\xe0\x00\x00\x00\x00\x00\x00\x07\xf8\xff\xff\xff\xff\xff\xff\x8f\xe0\x00\x00\x00\x00\x00\x00\x07\xff\x7f\xff\x3f\xfe\x7f\xfe\x3f\xe0\x00\x00\x00\x00\x00\x00\x07\xff\xdf\xff\xc0\x01\xff\xf8\xff\xe0\x00\x00\x00\x00\x00\x00\x07\xff\xf3\xff\xff\xff\xff\xe3\xff\xe0\x00\x00\x00\x00\x00\x00\x07\xff\xfc\x7f\xff\xff\xfe\x0f\xff\xe0\x00\x00\x00\x00\x00\x00\x07\xff\xfc\x1f\xff\xff\xfc\x4f\xff\xe0\x00\x00\x00\x00\x00\x00\x07\xff\xfc\x67\xff\xff\xe1\x0f\x1f\xe0\x00\x00\x00\x00\x00\x00\x07\xff\xfe\x70\xff\xff\x87\x3e\x3f\xe0\x00\x00\x00\x00\x00\x00\x07\xf9\xff\xbf\x00\x00\x7e\x79\xff\xe0\x00\x00\x00\x00\x00\x00\x07\xfc\xff\xcf\xff\xff\xfc\x61\xef\xe0\x00\x00\x00\x00\x00\x00\x07\xfe\x7f\xc7\xff\xff\xf0\xc7\x8f\xf0\x00\x00\x00\x00\x00\x00\x0f\xff\x3f\xe3\xf1\xff\xe3\x1e\x1f\xf8\x00\x00\x00\x00\x00\x00\x1f\xff\x9f\xf3\xf0\xff\xe6\x38\x7f\xf8\x00\x00\x00\x00\x00\x00\x1f\xff\xcf\xfd\xfc\x7f\xc0\xe3\xff\xfc\x00\x00\x00\x00\x00\x00\x3f\xff\xe7\xfe\x7f\x3f\x83\x07\xff\xfc\x00\x00\x00')
chopper = bytearray(b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x7f\xff\xff\xff\xe0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x3f\xff\xff\xff\xff\xff\xc0\x00\x00\x00\x00\x00\x00\x00\x00\x07\xff\xff\xfd\xff\xff\xff\xfc\x00\x00\x00\x00\x00\x1c\xf8\x00\x7f\xff\xff\xff\xff\xff\xff\xff\xe0\x01\xf3\x80\x00\x3c\xf8\x03\xff\xff\xff\xff\xff\xff\xff\xff\xf8\x01\xf3\xc0\x00\x7d\xf0\x0f\xff\xff\xff\x7f\xff\xff\xff\xff\xff\x00\xfb\xe0\x00\xfd\xf0\x3f\xff\xff\xff\xff\xff\xff\xff\xff\xff\xc0\xfb\xf0\x00\xfb\xe0\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xf0\x79\xf0\x00\xfb\xc3\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xfc\x39\xf0\x01\xfb\xcf\xff\xfb\xff\xff\xff\xff\xff\xff\xfd\xff\xff\x39\xf0\x01\xf3\xcf\xff\xfb\xff\xff\xff\xff\xff\xfd\xfd\xff\xff\x7d\xf0\x01\xf3\xef\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x7c\xf8\x01\xf7\xef\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xfe\xfe\xf8\x01\xf7\xf7\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xfe\xfe\xf8\x01\xf7\xf7\xff\xff\xfb\xbf\xff\xff\xff\xdf\xff\xff\xfe\xfe\xf8\x03\xef\xf7\xff\xff\xff\xff\xff\xff\xff\xfd\xff\xff\xfc\xfe\x78\x03\xef\xfb\xff\xff\xff\xff\xff\xff\xff\xfd\xf7\xff\xfd\xfe\x7c\x83\xef\xfb\xfe\xfe\xff\xff\xff\xff\xff\xfd\xf7\xff\xfb\xfe\x7c\x83\xe7\xfd\xff\xff\xff\xbf\xff\xff\xff\xdd\xff\xff\xfb\xfe\xfc\xc3\xf7\xfd\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xfb\xfe\xfc\xc1\xf3\xf9\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xfb\xfc\xf8\x81\xfb\xe3\xff\xff\xff\xff\xfd\xfb\xff\xff\xff\xff\xfb\xfd\xf8\x83\xff\x83\xff\xff\xff\xff\xdf\xff\xbf\xff\xff\xff\xfc\x0f\xf8\xe7\xfe\x0f\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xfe\x01\xfe\xff\xf0\x1f\xfd\xff\xff\xdf\xff\xff\xff\xbf\xfb\xfb\xff\x80\x3f\xff\x80\x7f\xff\xff\xff\xff\xff\xff\xff\xfd\xff\xff\xff\xc0\x0f\xfc\x03\x7f\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xc0\x00\x00\x03\xdf\xff\xdf\xff\xff\xff\xff\xff\xff\xff\xff\xfe\x50\x00\x00\x38\x79\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xf7\xe0\xc0\x00\x3c\x0e\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x6f\xc3\xc0\x00\x3f\xc0\x7f\xff\xff\xff\xff\xff\xfc\xff\xff\xfe\x6c\x1f\xc0\x00\x3c\x10\xf7\xff\xff\x0f\xff\xff\xfb\xcf\xff\xfe\xe0\x00\xc0\x00\x1f\xff\xb7\xff\xff\xff\xff\xff\xff\xff\xff\xfe\xdf\xff\x80\x00\x3f\xff\xdb\xff\xff\xff\xff\xff\xff\xff\xff\xfd\xbf\xff\xc0\x00\x3f\xff\xfc\xff\xff\xff\xff\xff\xff\xff\xff\xf1\xbf\xff\xc0\x00\x3f\xfc\xfe\xff\x70\x77\xff\xff\xff\xe0\xff\xf7\xe7\xff\xc0\x00\x0f\xf7\xfe\xfe\xc0\x0f\xff\xff\xff\x00\x37\xf7\xf8\xff\x00\x00\x00\x7f\xfb\xff\x80\x0d\xff\xff\xfe\x00\x1f\xff\xff\xc0\x00\x00\x01\xff\xfb\xfd\x80\x0d\xff\xff\xfe\x00\x1f\xff\xff\xf8\x00\x00\x00\x7f\xfb\xfe\xc0\x0f\xff\xfb\xfb\x00\x37\xfb\xff\xe0\x00\x00\x00\x3f\xfb\xff\xf0\x3f\xfd\xff\xff\xc0\xff\xf9\xff\xc0\x00\x00\x00\x1f\xfb\xff\xff\xff\xff\x6f\xff\xff\xff\xf9\xff\x80\x00\x00\x00\x07\xbb\xff\xff\xff\xff\xff\xff\xff\xff\xf9\x9e\x00\x00\x00\x00\x01\xdb\xff\xff\xff\xff\xff\xff\xff\xff\xf9\xb0\x00\x00\x00\x00\x00\x1b\xff\xff\xff\xff\xdf\xff\xff\xff\xfd\x80\x00\x00\x00\x00\x00\x0b\xff\xff\xff\xfe\x07\xff\xff\xff\xf9\x80\x00\x00\x00\x00\x00\x0a\xff\xff\xff\xe0\x00\x7f\xff\xff\xf9\x80\x00\x00\x00\x00\x00\x0a\xff\xff\xff\xe0\x00\x7f\xff\xff\xe1\x80\x00\x00\x00\x00\x00\x0a\x7f\xff\xff\xf1\xfc\x7f\xff\xff\x41\x80\x00\x00\x00\x00\x00\x1a\x07\xff\xff\xf3\xfe\xff\xff\xff\x01\xc0\x00\x00\x00\x00\x00\x1c\x01\xff\xff\xff\xff\xff\xff\xfe\x02\xc0\x00\x00\x00\x00\x00\x18\x00\x7f\xff\xff\xff\xff\xff\xf0\x02\xc0\x00\x00\x00\x00\x00\x1c\x00\x03\xff\xfd\xff\xff\xfe\x00\x02\xc0\x00\x00\x00\x00\x00\x1c\x00\x00\x0f\xff\xff\xff\x00\x00\x02\xc0\x00\x00\x00\x00\x00\x1c\x00\x00\x00\x07\xfe\x00\x00\x00\x01\xc0\x00\x00\x00\x00\x00\x30\x00\x00\x01\x8b\xfc\x8c\x00\x00\x01\xc0\x00\x00\x00\x00\x00\x30\x00\x00\x00\x3e\x61\x44\x00\x00\x01\xc0\x00\x00\x00\x00\x00\x38\x00\x00\x02\x7f\xff\xfe\x00\x00\x01\xc0\x00\x00\x00\x00\x00\x38\x00\x00\x1a\xff\xef\xff\x80\x00\x06\xe0\x00\x00\x00\x00\x00\x60\x00\x00\x3d\xff\x7f\xff\xc0\x00\x05\xd0\x00\x00\x00\x00\x00\x70\x00\x00\xfb\xff\xff\xff\x30\x00\x07\xd0\x00\x00\x00\x00\x00\x70\x00\x00\xfb\xfe\xff\xfe\xf8\x00\x07\xf0\x00\x00\x00\x00\x00\x00\x00\x03\xff\xff\xfb\xfe\xf4\x00\x00\x00\x00\x00')
nami = bytearray(b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x1f\xff\xff\xff\x80\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x1f\xff\xff\xff\xff\xff\xc0\x00\x00\x00\x00\x00\x00\x00\x00\x03\xff\xff\xff\xff\xff\xff\xfe\x00\x00\x00\x00\x00\x00\x00\x00\x3f\xff\xff\xff\xf7\xff\xdf\xef\xf0\x00\x00\x00\x00\x00\x00\x03\xff\xff\xef\xfe\xf7\xff\xef\xf7\xf8\x00\x00\x00\x00\x00\x00\x3f\xff\xff\xbf\xff\x7b\xff\xfb\xfe\xfe\x00\x00\x00\x00\x00\x00\xff\xff\xfe\xff\xff\x7b\xff\xfd\xff\x7f\x80\x00\x00\x00\x00\x03\xff\xff\xfb\xff\x3f\xbc\xff\xfe\xff\xbf\xc0\x00\x00\x00\x00\x0f\xff\xff\xf7\xfe\xff\xfc\xff\xff\xff\xef\xf0\x00\x00\x00\x00\x3f\xff\xff\xf7\xfd\xff\xee\x7f\xff\xdf\xf7\xf8\x00\x00\x00\x00\x3f\xff\xff\xcf\xfb\xfe\xef\x7f\xff\xef\xfb\xfc\x00\x00\x00\x00\x7f\xfe\xff\xdf\xf3\xfe\xff\xbf\x7f\xf7\xfb\xfc\x00\x00\x00\x00\xff\xfd\xff\xbf\xa3\xff\x7f\x9f\xbf\xf7\xfb\xff\x00\x00\x00\x01\xff\xfb\xef\xbf\xa3\xff\x1f\xf7\xbd\xf9\xfb\xff\x80\x00\x00\x03\xff\xf7\x9f\xbf\x93\xff\x4f\xfd\xef\x79\xfc\xff\xc0\x00\x00\x03\xff\xef\xbf\xbe\x53\xfe\x77\xfe\x7f\xde\xfc\xff\xc0\x00\x00\x07\xff\xdf\x7f\x7e\x33\xfe\xfb\xf7\xb1\xef\x7f\x7f\xc0\x00\x00\x0f\xff\xfe\xfc\x7e\x33\xff\x79\xfb\xdc\x79\x7f\x7f\xe0\x00\x00\x1f\xff\x3e\xf9\x7c\xfd\xff\x7f\x7d\xc1\xde\x3f\x7f\xe0\x00\x00\x3f\xf3\xde\xf3\xbd\xfd\x3f\x7f\xfe\x6f\xef\x1f\xbf\xe0\x00\x00\x3f\xfb\xd9\xef\xdb\xfd\xbf\x3f\xfb\xb3\xf3\x9f\xbf\xe0\x00\x00\x7f\xef\xe9\xdf\xcb\xfe\xdf\x9f\xfe\x21\xfc\x9f\xdd\xf0\x00\x00\x7f\xef\xeb\xbf\xe3\xfe\xdf\x9f\xff\xd1\xfe\x8f\xce\x70\x00\x00\x4f\xef\xe7\x78\x7f\xff\x2f\x9f\xff\xf0\xff\x4f\xcf\xb0\x00\x00\x7f\xff\xe6\xff\x87\xff\xd7\xef\xff\x0f\xff\xf7\xf7\xd8\x00\x00\x77\xf3\xe7\xff\xff\x8f\xe9\xf7\x87\xff\xff\xf7\xf7\xdc\x00\x00\x7b\xbd\xdf\xf7\xbf\xcf\xfc\xfb\x9f\xbf\xff\xfb\xfb\xf8\x00\x00\x7b\xdd\xdf\x70\x00\x7f\xff\x07\xf6\x00\x1f\xfb\xfb\xfc\x00\x00\x3d\xde\x3f\x8c\x03\xdf\xff\xff\xf9\xc0\x7b\xfc\xfb\xfc\x00\x00\x3d\xee\x3f\x70\x00\x7f\xff\xff\xfe\x00\x0e\xfc\xf8\x78\x00\x00\x3e\x3a\xff\x8b\xe1\x9f\xfe\xff\xf9\xbe\x11\xff\x7b\xc0\x00\x00\x3d\xb2\xff\x39\xe0\xcf\xfe\xff\xf3\x3e\x1c\xff\x7b\xf0\x00\x00\x3d\xbc\xff\xf0\x00\x7f\xfe\xff\xfe\x00\x0f\xff\x79\xf0\x00\x00\x0e\x3e\xff\xf0\x00\x7f\xfe\xff\xfe\x00\x0f\xff\x7e\x70\x00\x00\x0e\x52\x3f\xf9\xfd\xff\xf9\xff\xff\xbf\x9f\xfc\x7c\xe0\x00\x00\x0f\x3d\x3f\xfc\x03\xbf\xf9\xff\xfd\xc0\x3f\xfc\x7d\xe0\x00\x00\x0f\x3d\x3f\xf7\xfe\xff\xf9\xff\xff\x7f\xef\xfc\x7d\xe0\x00\x00\x07\xd9\xdf\xff\xff\xff\xf7\xff\xff\xff\xff\xf8\x79\xc0\x00\x00\x07\xd1\xdf\xff\xff\xff\xf7\xff\xff\xff\xff\xf9\x79\xc0\x00\x00\x07\xdc\xdf\xff\xff\xff\xfb\xef\xff\xff\xff\xf8\x7b\xc0\x00\x00\x03\xfe\xdf\xff\xff\xff\xfe\xff\xff\xff\xff\xf8\xfb\x80\x00\x00\x03\xfb\x6f\xff\xff\xff\xff\xff\xff\xff\xff\xf4\xfb\x80\x00\x00\x03\xde\x2f\xff\xff\xfe\x00\x00\x7f\xff\xff\xf4\xfd\x80\x00\x00\x01\xde\xef\xff\xff\xe1\xff\xff\x87\xff\xff\xf4\xfd\xc0\x00\x00\x01\xef\xd7\xff\xff\xef\xff\xff\xf7\xff\xff\xcc\xfd\xc0\x00\x00\x01\xff\xdd\xff\xff\xe0\x00\x00\x07\xff\xff\x9b\xfb\xc0\x00\x00\x00\xfb\xef\x7f\xff\xf8\x00\x00\x1f\xff\xfe\xfb\xfb\xe0\x00\x00\x00\x7d\x99\x3f\xff\xfc\x7f\xfe\x3f\xff\xfc\xfb\xfb\xe0\x00\x00\x00\x7d\x99\x0f\xff\xfe\x00\x00\x7f\xff\xf3\xf7\x77\xe0\x00\x00\x00\x7d\xbe\x91\xff\xff\xff\xff\xff\xff\x83\xf7\x77\xf0\x00\x00\x01\xfd\x3c\x9f\x7f\xff\xfc\x3f\xff\xfe\x39\xf7\x67\xf0\x00\x00\x01\xf3\x38\x9f\x8f\xff\xff\xff\xff\xe1\x3c\xee\xce\x70\x00\x00\x01\xfa\xf6\x9f\xf8\xff\xf8\x1f\xff\x1f\x9e\xcc\xcf\x7c\x00\x00\x03\xee\xee\xcf\xfc\x1f\xff\xff\xf8\x3f\xcf\xcc\x9f\x3c\x00\x00\x03\xee\xef\x6f\x7c\xe7\xff\xff\xe7\xbf\xcf\xdd\xbf\x3c\x00\x00\x03\xde\xf7\x6f\x7e\x7e\xff\xff\x7f\xbf\x9f\x9b\xbf\x3e\x00\x00\x07\xdc\xf3\x6f\x7e\x7f\x83\xc1\xff\xdf\x9f\x3b\x7f\xbe\x00\x00\x07\xdd\xf9\x6f\x7c\xff\xff\xff\xff\xdf\x3f\x7b\x7f\xde\x00\x00\x0f\xbd\xf9\x37\xbd\xff\xff\xff\xff\xef\x3f\x76\x4f\xef\x00\x00\x0f\xbd\xfe\x97\xbd\xff\xff\xff\xff\xe7\x3f\x74\xce\x73\x00\x00\x0f\xbd\xfe\x97\xbb\xff\xff\xff\xff\xe6\xff\x74\xcf\xb3\x00')
sanji = bytearray(b'\x00\x00\x00\x7f\xf7\xfe\xef\xff\xf7\xf7\xfb\xf7\xff\x80\x00\x00\x00\x00\x01\xff\xef\xfd\xdf\xff\xdf\xfd\xfd\xff\xff\xe0\x00\x00\x00\x00\x03\xff\xff\xfb\xdf\xff\xff\xff\xfe\xfe\xff\xf8\x00\x00\x00\x00\x07\xef\xff\xff\xff\xf7\xff\xff\xff\xdf\xff\xfc\x00\x00\x00\x00\x0f\xff\xff\xff\xff\xff\xff\x7f\xff\xef\xef\xfe\x00\x00\x00\x00\x1e\xff\xff\xff\xff\xef\xff\x67\xff\xf7\xf7\xff\x00\x00\x00\x00\x3b\xff\xff\x7f\xff\xdf\xff\x01\xff\xff\xff\xff\xc0\x00\x00\x00\x7e\xff\xff\x7f\xff\xdf\xfc\xdc\x7f\xff\xff\x7f\xc0\x00\x00\x00\x6e\xff\xff\x7f\xff\x3f\xfc\xff\xdf\xff\xff\xbf\xc0\x00\x00\x01\xee\xff\xff\x7f\xff\x7f\xfb\xff\xe7\xff\xff\xbf\xe0\x00\x00\x01\xdd\xf9\xff\x7d\xfe\xff\xfb\xff\xfd\xff\xff\xdf\xe0\x00\x00\x03\xfd\xff\xfc\xfd\xfe\xff\xf7\xff\xfe\xff\xff\xf7\xf0\x00\x00\x03\xbd\xef\xfc\xfb\xff\xdf\xf7\xc3\xff\x77\xfb\xfb\xf0\x00\x00\x03\xfd\xff\xfc\xfb\xff\xdf\xef\x73\xfe\x3f\xfb\xfd\xf0\x00\x00\x07\xff\x7f\xfc\xfb\xff\xdf\xef\x7e\xf9\xe9\xf7\xfd\xf0\x00\x00\x07\xed\xff\xfb\xe7\xff\x3f\x7f\x9f\x9f\xf6\xf7\xfd\xf8\x00\x00\x07\xdd\xff\xf7\xc7\xff\x3f\x7f\xf0\xff\xf4\xf7\xfd\xe0\x00\x00\x0f\x9f\xdf\xef\x77\xff\x3d\x7f\x03\xf8\x1e\xfb\xfe\x60\x00\x00\x1f\xff\xff\x9f\xfb\xfe\xf9\xff\xff\xef\xee\xf3\xfe\x60\x00\x00\x1f\xff\x3f\x67\xfb\xfe\xf9\xeb\xff\xbf\xf6\xec\xfe\x60\x00\x00\x1f\xff\xff\x3f\xfb\xfe\xf9\xcb\xff\xff\xf6\xec\xfe\x60\x00\x00\x3f\x7f\xdf\xff\xfb\xfe\xf3\xcb\xff\xff\xf1\x9f\x7d\xc0\x00\x00\x7f\xbf\xef\xff\xff\xfe\xf3\xcf\x7f\xff\xd9\x9f\x7d\xc0\x00\x00\x3f\xdf\xef\xff\xfb\xf9\x37\x9f\xbf\xff\x77\x7f\xbb\xc0\x00\x00\x37\xef\x33\xff\xf5\xf3\x2f\x9f\xff\xfd\xf6\x7f\xbb\xc0\x00\x00\x37\xff\x3e\xfc\xe4\xe3\x8f\x9f\xfb\xff\xe9\x7f\xff\xe0\x00\x00\x1f\xfb\x9e\xfc\xe6\x4f\xff\x9f\xff\xdf\xef\x7b\xff\xb8\x00\x00\x1f\xbd\x9e\xf8\x4f\x1f\xff\x9f\xff\xff\xff\x79\xff\xc0\x00\x00\x0c\x7d\x8e\xf8\x4f\xff\xff\x9f\xff\xff\xfe\xf4\x7a\x60\x00\x00\x04\x7f\xc6\x33\x1d\xff\xff\xf7\xff\xfd\xfe\xe4\xba\x00\x00\x00\x02\x7f\xed\x07\xff\xdd\xdf\xff\xfd\xdf\xfe\xc9\xfa\x00\x00\x00\x02\x7f\xe7\x7f\xbe\x3f\xff\x77\x7f\xf9\xf6\xc3\xdc\x00\x00\x00\x00\x3f\xc8\x7f\x7f\xc0\x07\xff\x80\x0f\xf8\x07\x0c\x00\x00\x00\x00\x31\x9e\x7f\x83\xff\xff\xff\xff\xff\x87\xeb\x34\x1c\x00\x00\x00\x20\x0c\x3f\x80\x01\xff\xff\xfe\x00\x07\x91\xb8\x39\x00\x00\x00\x20\x08\x1f\xc0\x00\xff\x03\xfc\x00\x0f\x34\xbc\x03\x00\x00\x00\x00\x00\x0f\xf0\x01\xff\xff\xff\x00\x1f\xcf\xff\x00\x80\x00\x00\x00\x00\x07\xf8\x33\xff\xff\xff\xb0\x7f\xbf\xfe\x01\x00\x00\x00\x00\x00\x01\xff\x3c\x1f\xff\xf0\x73\xfe\xff\xe0\x00\x00\x00\x00\x00\x00\x00\x7f\xcf\xe0\x00\x1f\xcf\xf8\xf0\x00\x00\x00\x00\x00\x00\x00\x00\xdf\xff\xc0\xfe\x07\xed\xef\xc0\x00\x00\x00\x00\x00\x00\x01\xfc\xf7\xff\xff\x03\xff\xfa\xdf\xf8\x00\x00\x00\x00\x00\x01\xff\xfc\xfd\xff\xff\xff\xff\xfe\xde\xfe\x00\x00\x00\x00\x00\x1f\xff\xfc\xff\x7f\xf8\xfc\x3f\xfd\xbc\xff\xe0\x00\x00\x00\x00\x3f\xff\xfc\xff\xdf\xff\x03\xff\xef\xfe\xff\x7e\x00\x00\x00\x00\x7f\xcf\xfc\xff\xf7\xff\x87\xff\x9f\xfe\xff\x8f\xc0\x00\x00\x00\xff\x9f\xfc\xff\xfd\xff\x87\xfe\x7f\xfe\xff\xcf\xf8\x00\x00\x01\xff\x3f\xfc\xff\xff\x00\x00\x03\xff\xfe\xff\xcf\xfe\x00\x00\x01\xff\x3f\xfe\x7f\xff\xc0\x00\x07\xff\xf9\xff\x8f\xff\x00\x00\x01\xff\x3f\xfe\x7f\xff\xff\xff\xff\xff\xf9\xff\xbf\xff\x00\x00\x00\xff\x9f\xfe\x7f\xbf\xff\xff\xff\xf3\xf9\xff\xbf\xff\x00\x00\x00\x7f\xef\xfe\x7f\xdf\xff\x9f\xff\xe7\xf9\xff\xbf\xfe\x00\x00\x00\x7f\xe3\xfe\x7f\xcf\xff\xcf\xff\xcf\xf9\xfe\x7f\xfe\x00\x00\x00\x3f\xf3\xfe\x7f\xe7\xff\xcf\xff\xdf\xf9\xfc\xff\xfe\x00\x00\x00\x3f\xf9\xfe\x7f\xf3\xff\xff\xff\xbf\xf9\xf3\xff\xfe\x00\x00\x00\x3f\xfe\xfe\x7f\xfd\xff\xff\xfe\x7f\xf9\xe7\xff\xfe\x00\x00\x00\x1f\xff\x7c\xff\xfe\xff\xff\xfc\xff\xfc\x9f\xff\xfc\x00\x00\x00\x1f\xff\x89\xff\xfe\xff\xff\xfd\xff\xfe\x3f\xff\xfc\x00\x00\x00\x0f\xff\xf7\xff\xff\xff\xff\xff\xff\xfe\xff\xff\xfc\x00\x00\x00\x07\xff\xf9\xff\xff\xff\xff\xff\xff\xf9\xff\xff\xfc\x00\x00\x00\x07\xff\xff\x7f\xff\xff\xff\xff\xff\xf1\xff\xff\xf0\x00\x00\x00\x03\xff\xfe\x1f\xff\xff\xff\xff\xff\xe8\xff\xff\xf0\x00\x00\x00\x03\xff\xfc\xe7\xff\xff\xff\xff\xff\xdf\x7f\xff\xf0\x00\x00\x00\x01\xff\xf8\xf9\xff\xff\xff\xff\xff\x3f\x7f\xff\xf0\x00')
robin = bytearray(b'\x00\x01\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xf0\x00\x00\x07\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xfc\x00\x00\x0f\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xfc\x00\x00\x1f\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xfe\x00\x00\x1f\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xfe\x00\x00\x1f\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xfe\x00\x00\x1f\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xfe\x00\x00\x1f\xff\xff\xf8\x1f\xff\xff\xff\xff\xf8\x1f\xff\xff\xfe\x00\x00\x3f\xff\xfd\xfe\x3f\xe7\xff\xff\xe7\xfc\x7f\xbf\xff\xff\x00\x00\x3f\xff\xf7\x03\xc0\x3d\xff\xff\xbc\x03\xc0\xef\xff\xff\x00\x00\x3f\xff\xdd\xff\xff\xf3\xff\xff\xff\xff\xff\xbb\xff\xff\x00\x00\x7f\xff\xf7\xff\xff\xfd\xdf\xfb\xbf\xff\xff\xcf\xff\xff\x80\x00\x7f\xff\x2f\xff\xff\xfe\xdc\x3b\x7f\xff\xff\xcc\xff\xff\xc0\x00\x7f\xfd\x2f\xff\xff\xfe\xec\x37\x7f\xff\xff\xcc\xbf\xff\xc0\x00\x7f\xfd\x2f\xff\xff\xff\x27\xe4\xff\xff\xff\xcc\xbf\xff\xc0\x00\x7f\xfd\x37\xff\xff\xfe\xef\xf7\x7f\xff\xff\xcc\xbf\xff\xc0\x00\x7f\xfd\x17\xff\xff\xfc\xdf\xfb\x7f\xff\xff\xc8\xbf\xff\xc0\x00\x7f\xfb\x2d\xff\xff\xfd\xbf\xfd\xbf\xff\xff\xb4\xdf\xff\xc0\x00\x7f\xfb\x2e\x3f\xff\xf3\xff\xff\xcf\xff\xfc\x77\xdf\xff\xc0\x00\x7f\xfa\xf7\xe3\xfe\x0e\xff\xff\x70\x7f\xc7\xef\x77\xff\xc0\x00\x3f\xfa\xff\xff\xcf\xff\xff\xff\xff\xf3\xff\xff\x77\xff\x00\x00\x3f\xee\xff\xf8\x78\xff\xff\xff\xff\x1e\x1f\xff\xb7\xff\x00\x00\x1f\xed\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xbb\xfe\x00\x00\x1f\xed\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xbb\xfe\x00\x00\x0f\xcd\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xdb\xfe\x00\x00\x07\xdd\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xdd\xfc\x00\x00\x07\xdb\xff\xff\xff\xf8\x00\x03\xff\xff\xff\xff\xf5\xfc\x00\x00\x07\xdb\xff\xff\xff\x00\x00\x3e\x3f\xff\xff\xff\xf5\xf8\x00\x00\x03\xdb\xff\xff\x80\x00\x00\xff\xfc\xff\xff\xff\xf5\xf8\x00\x00\x03\xdb\xff\xf0\x00\x00\x07\xff\xff\x8f\xff\xff\xf6\x70\x00\x00\x01\xdb\xff\xc0\x00\x00\x1f\xff\xff\xfb\xff\xff\xf6\x60\x00\x00\x01\xaf\xff\x00\x00\x01\xff\xff\xff\xfe\xff\xff\xfa\x60\x00\x00\x00\x2f\xfc\x00\x00\x0f\xff\xff\xff\xfb\x3f\xff\xfb\xa0\x00\x00\x00\x27\xf8\x00\x0c\xf0\xff\xfc\x3f\xde\x0f\xff\xf5\x80\x00\x00\x00\x2f\xf0\x03\x00\x07\xbf\xf3\x86\x32\x03\xff\xf1\x80\x00\x00\x00\x19\xe0\x00\x00\x00\xef\xef\x60\x05\x00\xff\xfa\x00\x00\x00\x00\x0f\xc0\x00\x0f\x21\xf7\xf9\x8f\x00\x00\x3f\xfc\x00\x00\x00\x00\x1f\x80\x03\xce\x2f\xff\xff\xce\x2f\x00\x6f\xfe\x00\x00\x00\x00\x3e\xe0\x07\xc8\x6d\xff\xff\xc8\x6f\xc0\x3b\xff\xc0\x00\x00\x00\xfe\x40\x07\x27\xd3\xff\xff\xa7\xd1\xc0\x68\xff\xe0\x00\x00\x03\xfc\xe0\x07\xe0\x3f\xf9\x3f\xf0\x1f\xc0\x78\x1f\xfc\x00\x00\x0f\xf0\x80\x07\xff\xff\xf9\x7f\xff\xff\xc0\x10\x37\xff\x00\x00\x07\xd0\x80\x0f\xff\xff\xf9\x7f\xff\xff\xe0\x10\x3f\xfe\x00\x00\x03\xf0\x80\x0f\xff\xff\xf9\x7f\xff\xff\xe0\x00\x0f\xf8\x00\x00\x00\xfc\x00\x0f\xff\xff\xf9\x7f\xff\xff\xe0\x00\x07\xf0\x00\x00\x00\x1c\x00\x0f\xff\xff\xf9\x7f\xff\xff\xe0\x00\x07\x80\x00\x00\x00\x00\x00\x1f\xff\xff\xfe\xff\xff\xff\xf0\x00\x00\x00\x00\x00\x00\x00\x00\x0f\xff\xff\xff\xff\xff\xff\xe0\x00\x00\x00\x00\x00\x00\x00\x00\x0f\xff\xff\xff\xff\xff\xff\xe0\x00\x00\x00\x00\x00\x00\x00\x00\x17\xff\xff\xff\xff\xff\xff\xc0\x00\x00\x00\x00\x00\x00\x02\x00\x07\xff\xff\xff\xff\xff\xff\x98\x00\x00\x00\x00\x00\x00\x03\x00\x03\xff\xff\xcf\xf3\xff\xff\x00\x00\x04\x00\x00\x00\x00\x01\x00\x00\xff\xff\xff\xff\xff\xfe\x00\x00\x00\x40\x00\x00\x00\x00\x00\x00\x7f\xff\xff\xff\xff\xfc\x00\x00\x02\x00\x00\x00\x00\x00\x00\x00\x1f\xff\xf8\x1f\xff\xf0\x00\x00\x00\x20\x00\x00\x00\x00\x00\x00\x03\xff\xff\xff\xff\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x02\xff\xff\xff\xfd\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\x87\xff\xff\xe3\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\xfc\xff\xfc\x7e\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xff\x8f\xe3\xfc\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x7f\xf7\x9f\xfc\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x7f\xfc\x7f\xfc\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x7f\xff\xff\xfc\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\xff\xff\xff\xfe\x00\x00\x00\x00\x00\x00')
jinbei = bytearray('\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xfd\x3f\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xf0\x1f\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xe0\x0f\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xe0\x1f\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xc1\x1f\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xc1\x9f\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xe1\xbf\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xf9\xcf\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xf8\xe1\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xe0\x60\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xc1\x30\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xc3\xf0\xbf\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x87\xf9\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x87\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x83\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x07\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x8f\x9f\xbf\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xcf\xbf\x87\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xf9\x7f\xbf\xaf\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xc1\xdf\xff\xb7\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xc1\xfd\xff\xf7\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xf1\xdf\xff\xef\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xfb\xff\xf9\xfe\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xf0\xff\xbf\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xf0\x7f\x7f\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xfd\xff\xff\xf0\xfe\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xf8\xff\xff\xff\x8f\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xdf\xff\xff\x7b\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xc7\xcf\xff\xf8\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xfe\x5b\x07\xff\xf9\xbf\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xfc\xef\x83\xff\xf9\xff\xbf\xff\xff\xff\xff\xff\xff\xff\xff\xff\xfb\xff\xe1\xff\xf1\x7f\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xf3\x93\xf0\x7f\xf3\x3f\x7f\xff\xff\xff\xff\xff\xff\xff\xff\xff\xe7\xf7\xc8\x3f\xe6\x1f\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xc7\xe9\xe4\x1f\xc4\x49\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x8f\xdf\x76\x0f\x8d\x66\x7f\xff\xff\xff\xff\xff\xff\xff\xff\xff\x0f\x9f\x7b\x0f\x08\x70\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x1f\xaf\xf9\x86\x11\x2e\xff\xff\xff\xff\xff\xff\xff\xff\xff\xfe\x1f\x33\xfd\xc0\x31\x2d\xff\xff\xff\xff\xff\xff\xff\xff\xff\xfe\x1f\x44\x2b\xe0\x73\x91\xff\xff\xff\xff\xff\xff\xff\xff\xff\xf8\x5f\x76\x17\xe0\xf8\x1f\xff\xff\xff\xff\xff\xff\xff\xff\xff\xf8\x5e\x17\x0f\xc1\xfd\xcf\xff\xff\xff\xff\xff\xff\xff\xff\xff\xfc\x0e\x67\x9f\x80\xfc\xc6\xff\xff\xff\xff\xff\xff\xff\xff\xff\xf0\x0f\x2f\xfe\x0b\x7f\x80\xff\xff\xff\xff\xff\xff\xff\xff\xff\xf8\x07\x4f\xf8\x34\x3f\xa0\xff\xff\xff\xff\xff\xff\xff\xff\xff\xe8\x13\x9e\xe0\xeb\xb9\xa0\xff\xff\xff\xff\xff\xff\xff\xff\xff\xe8\x00\x1f\xdf\xc0\x9f\x21\x7f\xff\xff\xff\xff\xff\xff\xff\xff\xe0\x07\xe3\xff\x7f\xfe\x81\x7f\xff\xff\xff\xff\xff\xff\xff\xff\xe0\x23\xd0\x7f\xff\xf0\x01\x7f\xff\xff\xff\xff\xff\xff\xff\xff\xe0\x0c\xe6\x6f\x80\x10\x00\x7f\xff\xff\xff\xff\xff\xff\xff\xff\xc0\x1f\x62\xe1\xdc\xe0\x80\x7f\xff\xff\xff\xff\xff\xff\xff\xff\xd0\x5f\xb4\xcd\xff\xe3\x00\xbf\xff\xff\xff\xff\xff\xff\xff\xff\xd0\x1f\x90\xdd\xff\xfc\xe4\xbf\xff\xff\xff\xff\xff\xff\xff\xff\xd9\xff\x0e\x1d\xbf\xfb\xf7\xbf\xff\xff\xff\xff\xff\xff\xff\xff\xe7\xff\xfe\xfb\xff\xf1\xfc\x7f\xff\xff\xff\xff')
images_list = [strawhat_jolly_roger, luffy_image, ussop, zoro, chopper, nami, sanji, robin, jinbei]
Next, we will display the images using the following code.
from machine import Pin, SoftI2C
import ssd1306
import framebuf
import images_repo
import utime
# using default address 0x3C
i2c = SoftI2C(sda=Pin(22), scl=Pin(23))
display = ssd1306.SSD1306_I2C(128, 64, i2c)
while True:
for image in images_repo.images_list:
buffer = image
fb = framebuf.FrameBuffer(buffer, 128, 64, framebuf.MONO_HLSB)
display.fill(0)
display.blit(fb, 8, 0)
display.show()
utime.sleep_ms(2000)
Let’s run through what each line of code does.
from machine import Pin, SoftI2C
import ssd1306
import framebuf
import images_repo
import utime
Import the necessary packages to drive our SSD1306 OLED component including our list of images using the image_repo.py Python file.
# using default address 0x3C
i2c = SoftI2C(sda=Pin(22), scl=Pin(23))
display = ssd1306.SSD1306_I2C(128, 64, i2c)
Declare our SoftI2C
and SSD1306_I2C
classes to enable communication with our SSID1306 OLED display from our MicroPython code.
while True:
for image in images_repo.images_list:
buffer = image
fb = framebuf.FrameBuffer(buffer, 128, 64, framebuf.MONO_HLSB)
display.fill(0)
display.blit(fb, 8, 0)
display.show()
utime.sleep_ms(2000)
Using an infinite while loop and a for loop then we will scan all the images in Python bytearray
format.
As MicroPython does not support loading images from files then we are using the FrameBuffer blit() method or bit block transfer to transfer the image in memory into our SSD1306 display. More details about this can be found in the documentation of MicroPython.
By using the code above then we are able to display bitmap or images into our SSD1306 OLED display using MicroPython.
That is all for the two mini projects using our SSD1306 and MicroPython code. I really had so much fun doing this project, especially the display of Anime images in our SSD1306 OLED display :).
Wrap Up
Using the SSD1306 OLED as a way to display information for your Internet of Things (IoT) project is an excellent way. Programming them using MicroPython is really easy and all you need to do is perform trial and error to achieve your desired layout.
I hope you learn something! Happy Exploring!
Read Next:
Building a MicroPython Wifi Robot Car
Control DS18B20 using MicroPython with a Weather Station Project
Leave a Reply