Introduction
If you are new to MicroPython programming then you might think that a task like how to blink an LED (Light Emitting Diode) is a trivial one and does not give you much knowledge in the future. However, as I am about to show in this post, having this basic knowledge would be useful in the long run. After all, blinking an LED in MicroController programming is similar to the “Hello World” program in different languages such as C++/Python/Java/Javascript.
For a video demo presentation of this post please see below.
Prerequisites
The following are the components needed to follow along with this post.
- ESP32/ESP8266 (I used NodeMCU ESP32s)
- LED ( At least 4 different colors and 2 per color)
- Resistors (220 Ohms)
- Breadboard and Wires
You should have downloaded the latest MicroPython firmware for your MicroController Unit (MCU).
Related Content:
How to install MicroPython on ESP32 and download firmware
You can use any IDE that you are familiar with such as Thonny, Visual Studio Code using PyMakr, or even the Arduino Lab for MicroPython.
Related Content:
MicroPython using VSCode PyMakr on ESP32/ESP8266
Arduino officially supports MicroPython
I will be using Thonny in this post. Make sure that you have done all these prerequisites before moving forward.
How do you blink an LED in MicroPython?
Now let us answer first the very basic question of how to perform in MicroPython the blinking of an LED.
For this initial exercise, you won’t need any external components but only an ESP32 or ESP8266 as we will be blinking the onboard LED tied to the GPIO2 pin.
Option 1: Using an infinite loop
The code below is the very simple way of blinking an LED in MicroPython and what it does is initialize the pin that we are gonna be using and using a Python while loop we will just toggle the value of the LED. We will sleep for some time before continuing again.
Now as you might have noticed, we are using an infinite loop in making a continuous blinking of the LED.
from machine import Pin
from utime import sleep_ms
ON_BOARD_PIN = 2
led_pin = Pin(ON_BOARD_PIN, Pin.OUT)
while True:
led_pin.value(not led_pin.value())
sleep_ms(1000)
# The more verbose way
# led_pin.value(1)
# sleep_ms(1000)
# led_pin.value(0)
# sleep_ms(1000)
From the code above toggling the .value() of a machine.Pin would blink the LED. Isn’t it that simple?
Option 2: Using a Timer
Another option to explore is using the Timer class of MicroPython. The code below will show you how to blink an LED.
from machine import Pin, Timer
ON_BOARD_PIN = 2
led_pin = Pin(ON_BOARD_PIN, Pin.OUT)
def toggle_led(t):
led_pin.value(not led_pin.value())
led_timer = Timer(1)
led_timer.init(mode=Timer.PERIODIC,period=1000,callback=toggle_led)
Let us run through the code and explain what it does. Notice that there is no infinite loop in our program.
from machine import Pin, Timer
ON_BOARD_PIN = 2
led_pin = Pin(ON_BOARD_PIN, Pin.OUT)
We first import the Pin and Timer class from the machine package. Initialize the pin and set it for output.
def toggle_led(t):
led_pin.value(not led_pin.value())
This is the callback function that will be called when the configured period that we have in our Timer has expired.
led_timer = Timer(1)
led_timer.init(mode=Timer.PERIODIC,period=1000,callback=toggle_led)
We declare a Timer class and set it to an id of 1. We then call the init method passing in a mode of Timer.PERIODIC, a period of 1000, and the callback function that will be called.
Deploying or running our project
Using Thonny, we can just click the Run the Current Script button
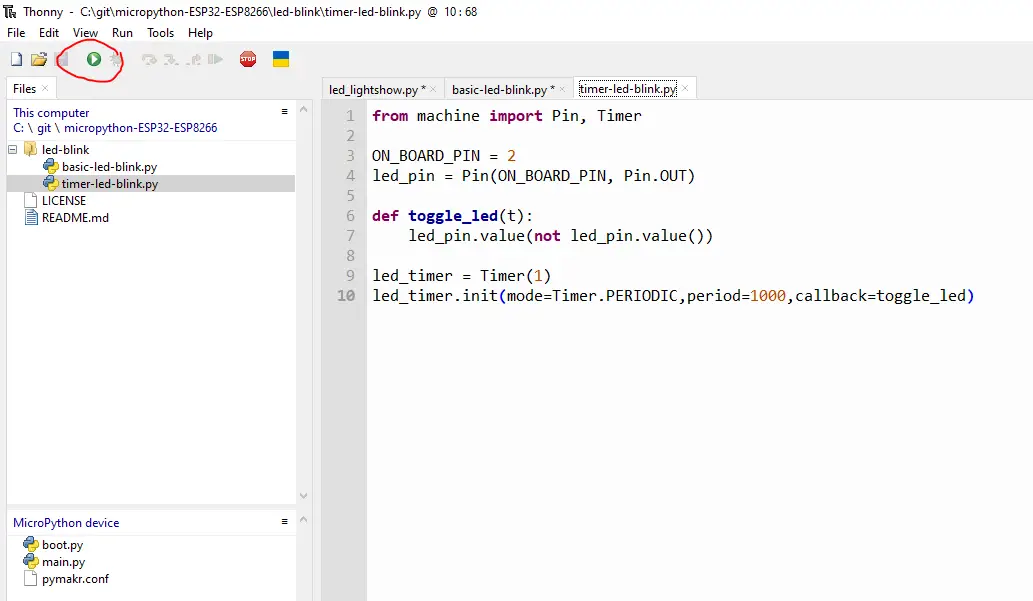
When you run your script you would see that it would blink your onboard LED like below. Congratulations! You are now a master of blinking an LED. 🙂
I now know how to blink an LED in MicroPython, now what?
As I have mentioned in the introduction of this post, learning how to blink an LED in MicroPython is the first step in exploring this language. Now that you know how to do it you are now ready to explore different features of the Python language.
I now present you my LED light show. We will use the knowledge that you have right now to create an LED Light show using MicroPython and control how to blink each LED(s). We will explore Python concepts such as iterating, slicing, and reversing lists with just this simple knowledge of MicroPython being able to blink your LED.
Let us now find out how to do this starting with the wiring and schematic.
Wiring/Schematic – LED Lightshow
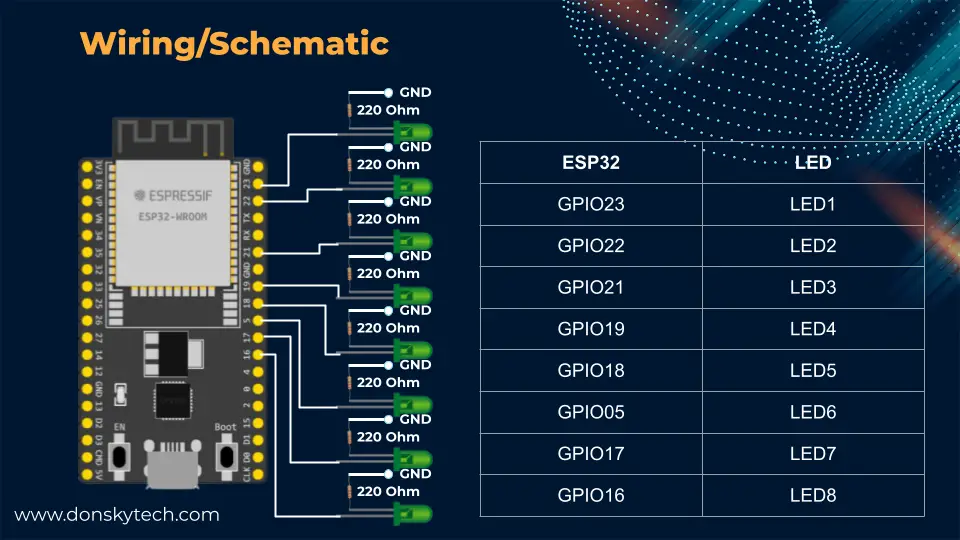
The image above is the wiring diagram of my LED light show. Just remember to connect a current-limiting 220-ohms resistor between the cathode of the LED(s) and the ground of your MCU.
Code
The code for this project is in my GitHub repository.
'''
Description: LED Light show using MicroPython with ESP32 and ESP8266 board
Author: donskytech
'''
from machine import Pin, Timer
from utime import sleep_ms
led_pin_numbers = [23, 22, 21, 19, 18, 5, 17, 16]
led_pins = []
OFF = 0
ON = 1
SHORT_SLEEP_MS = 100
MEDIUM_SLEEP_MS = 500
# Setup
def setup_pins():
for pin in led_pin_numbers:
led_pins.append(Pin(pin, Pin.OUT))
def turn_off_leds():
for pin in led_pins:
pin.value(OFF)
def turn_on_leds():
for pin in led_pins:
pin.value(ON)
def running_leds():
for pin in led_pins:
turn_off_leds()
pin.value(ON)
sleep_ms(SHORT_SLEEP_MS)
for pin in reversed(led_pins):
turn_off_leds()
pin.value(ON)
sleep_ms(SHORT_SLEEP_MS)
def mixer_leds():
turn_off_leds()
for pin in led_pins:
pin.value(ON)
sleep_ms(SHORT_SLEEP_MS)
turn_off_leds()
for pin in reversed(led_pins):
pin.value(ON)
sleep_ms(SHORT_SLEEP_MS)
def blink_blink_leds():
for _ in range(5):
turn_on_leds()
sleep_ms(SHORT_SLEEP_MS)
turn_off_leds()
sleep_ms(SHORT_SLEEP_MS)
def odd_even_leds():
turn_off_leds()
for pin in led_pins[::2]:
pin.value(ON)
sleep_ms(MEDIUM_SLEEP_MS)
turn_off_leds()
for pin in led_pins[1::2]:
pin.value(ON)
sleep_ms(MEDIUM_SLEEP_MS)
turn_off_leds()
for pin in led_pins[-1::-2]:
pin.value(ON)
sleep_ms(MEDIUM_SLEEP_MS)
turn_off_leds()
for pin in led_pins[-2::-2]:
pin.value(ON)
sleep_ms(MEDIUM_SLEEP_MS)
def dual_blink_leds():
for index in [2,6,0,4]:
turn_off_leds()
for pin in led_pins[index:index+2:]:
pin.value(ON)
sleep_ms(MEDIUM_SLEEP_MS)
def led_light_show(t):
led_effects = [blink_blink_leds, running_leds, dual_blink_leds, mixer_leds, odd_even_leds]
for effect in led_effects:
effect()
# blink_blink_leds()
# running_leds()
# dual_blink_leds()
# mixer_leds()
# odd_even_leds()
def main():
setup_pins()
led_timer = Timer(1)
led_timer.init(mode=Timer.PERIODIC,period=1000,callback=led_light_show)
if __name__ == '__main__':
main()
The code above is how we will drive our LED light show using MicroPython. Let us run through what each line does for you to understand.
from machine import Pin, Timer
from utime import sleep_ms
led_pin_numbers = [23, 22, 21, 19, 18, 5, 17, 16]
led_pins = []
OFF = 0
ON = 1
SHORT_SLEEP_MS = 100
MEDIUM_SLEEP_MS = 500
We import the necessary MicroPython packages and define several constants including our pin assignments.
# Setup
def setup_pins():
for pin in led_pin_numbers:
led_pins.append(Pin(pin, Pin.OUT))
We set up our LED pins and add everything to our led_pins
list.
def turn_off_leds():
for pin in led_pins:
pin.value(OFF)
def turn_on_leds():
for pin in led_pins:
pin.value(ON)
Functions that will iterate our led_pins
and turn it on or off.
'''
Description: LED Light show using MicroPython with ESP32 and ESP8266 board
Author: donskytech
'''
from machine import Pin, Timer
from utime import sleep_ms
led_pin_numbers = [23, 22, 21, 19, 18, 5, 17, 16]
led_pins = []
OFF = 0
ON = 1
SHORT_SLEEP_MS = 100
MEDIUM_SLEEP_MS = 500
# Setup
def setup_pins():
for pin in led_pin_numbers:
led_pins.append(Pin(pin, Pin.OUT))
def turn_off_leds():
for pin in led_pins:
pin.value(OFF)
def turn_on_leds():
for pin in led_pins:
pin.value(ON)
def running_leds():
for _ in range(2):
for pin in led_pins:
turn_off_leds()
pin.value(ON)
sleep_ms(SHORT_SLEEP_MS)
for pin in reversed(led_pins):
turn_off_leds()
pin.value(ON)
sleep_ms(SHORT_SLEEP_MS)
def mixer_leds():
turn_off_leds()
for pin in led_pins:
pin.value(ON)
sleep_ms(SHORT_SLEEP_MS)
turn_off_leds()
for pin in reversed(led_pins):
pin.value(ON)
sleep_ms(SHORT_SLEEP_MS)
def blink_blink_leds():
for _ in range(5):
turn_on_leds()
sleep_ms(SHORT_SLEEP_MS)
turn_off_leds()
sleep_ms(SHORT_SLEEP_MS)
def odd_even_leds():
turn_off_leds()
for pin in led_pins[::2]:
pin.value(ON)
sleep_ms(MEDIUM_SLEEP_MS)
turn_off_leds()
for pin in led_pins[1::2]:
pin.value(ON)
sleep_ms(MEDIUM_SLEEP_MS)
turn_off_leds()
for pin in led_pins[-1::-2]:
pin.value(ON)
sleep_ms(MEDIUM_SLEEP_MS)
turn_off_leds()
for pin in led_pins[-2::-2]:
pin.value(ON)
sleep_ms(MEDIUM_SLEEP_MS)
def dual_blink_leds():
for _ in range(2):
for index in [2,6,0,4]:
turn_off_leds()
for pin in led_pins[index:index+2:]:
pin.value(ON)
sleep_ms(MEDIUM_SLEEP_MS)
def led_light_show(t):
led_effects = [blink_blink_leds, running_leds, dual_blink_leds, mixer_leds, odd_even_leds]
for effect in led_effects:
effect()
# blink_blink_leds()
# running_leds()
# dual_blink_leds()
# mixer_leds()
# odd_even_leds()
def main():
setup_pins()
led_timer = Timer(1)
led_timer.init(mode=Timer.PERIODIC,period=1000,callback=led_light_show)
if __name__ == '__main__':
main()
These are our LED Lightshow effects function. As you can see, it uses list methods such as how to iterate, slice, and reverse. By only learning how to blink an LED and expanding that knowledge by adding more components you have the chance to increase your MicroPython programming knowledge.
def led_light_show(t):
led_effects = [blink_blink_leds, running_leds, dual_blink_leds, mixer_leds, odd_even_leds]
for effect in led_effects:
effect()
# blink_blink_leds()
# running_leds()
# dual_blink_leds()
# mixer_leds()
# odd_even_leds()
This is our callback function by our Timer class. It scans through the list of effects and calls it. We can call the function manually as per the commented code above but putting it in a list will allow us flexibility later to add more effects.
def main():
setup_pins()
led_timer = Timer(1)
led_timer.init(mode=Timer.PERIODIC,period=1000,callback=led_light_show)
if __name__ == '__main__':
main()
This is the main entry point of our program where we define a Timer class and the necessary callback.
Deploying it to our ESP32/ESP8266 file system
If you want to deploy this program into your ESP32/ESP8266 MCU then you can create your own main.py or boot.py and upload it into your device file system. You then can upload it using Thonny by right-clicking your file and uploading it to your device.
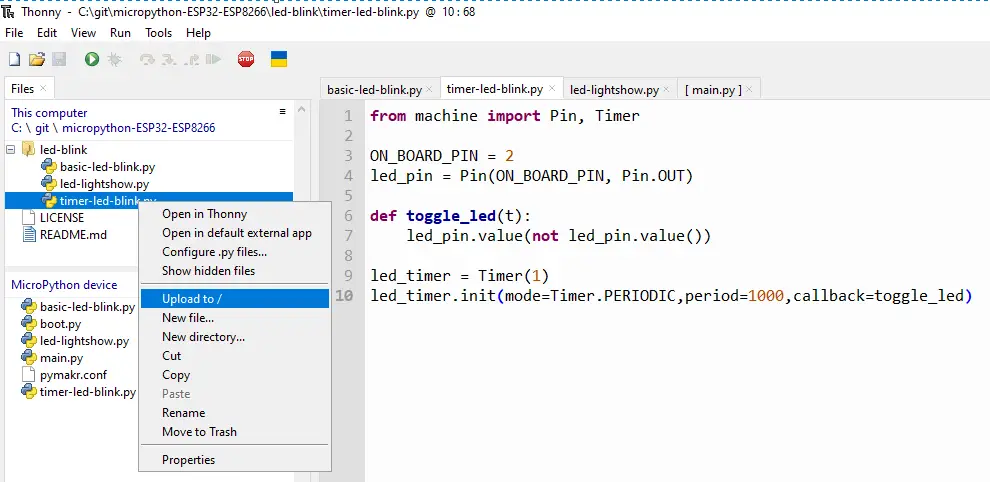
Just be aware that when you upload it as boot.py or main.py it will run the script when you restart or hard reset your ESP32/ESP8266 MCU.
However, for the sake of this tutorial, we will just click the “Run the current script button” in Thonny and see how it works. If you really want to upload it into the file system of your MCU then you can run your code by executing the below command in your REPL prompt.
exec(open("led-lightshow.py").read())
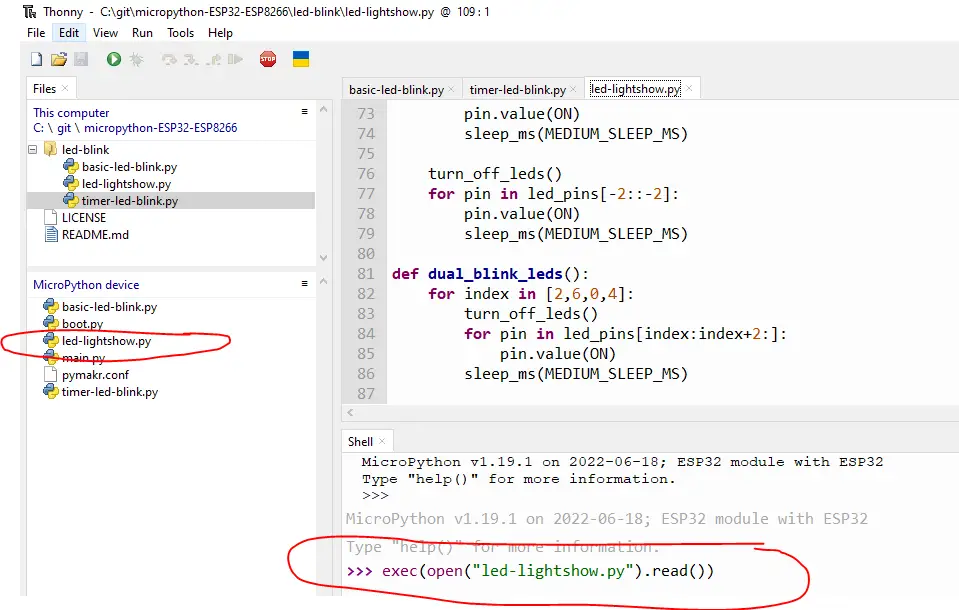
Wrap up
We have explored how to create a MicroPython program that will blink LED(s) in this post. Also, we had the chance to explore several Python concepts such as list iteration, slicing, and reversing data with only the basic knowledge of how to blink an LED.
I hope you had fun doing this simple exercise and have learned something from it.
Happy Exploring!
Read Next:
Raspberry Pi RFID Door Lock System with Database
Raspberry Pi Object Counting using an Infrared sensor
Support Me!
I love sharing what I know and hopefully, I was able to help you. Writing helpful content takes so much time and research. If you think you like my work and I have managed to help you then please consider supporting my channel. I would be very grateful and would boost my confidence that what I am doing is making a big change in the world. (No Pun Intended!) 😉
Become a Patron!
Leave a Reply